590. N叉树的后序遍历
590. N-ary Tree Postorder Traversal
题目描述
给定一个 N 叉树,返回其节点值的后序遍历。
LeetCode590. N-ary Tree Postorder Traversal
例如,给定一个 3 叉树:
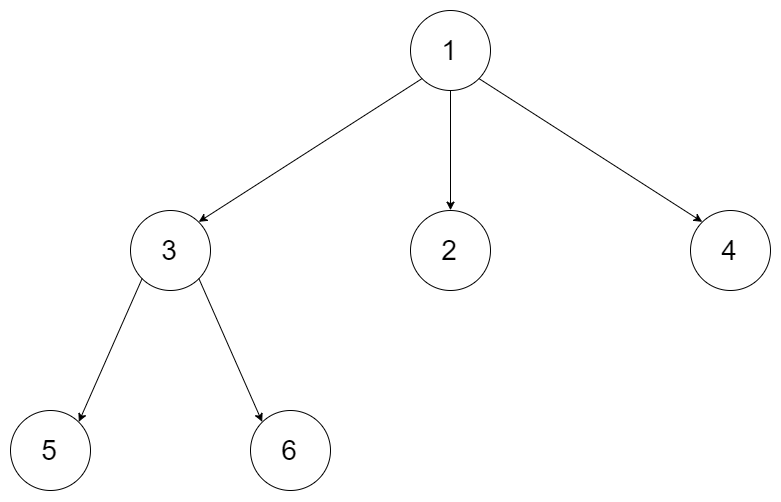
返回其后序遍历: [5,6,3,2,4,1].
说明: 递归法很简单,你可以使用迭代法完成此题吗?
Java 实现
Iterative Solution
import java.util.LinkedList;
import java.util.List;
import java.util.Stack;
class Node {
public int val;
public List<Node> children;
public Node() {
}
public Node(int _val, List<Node> _children) {
val = _val;
children = _children;
}
}
class Solution {
public List<Integer> postorder(Node root) {
List<Integer> result = new LinkedList<>();
if (root == null) {
return result;
}
Stack<Node> stack = new Stack<>();
stack.push(root);
while (!stack.isEmpty()) {
Node node = stack.pop();
for (Node child:node.children){
stack.push(child);
}
result.add(0, node.val);
}
return result;
}
}
Recursive Solution
import java.util.LinkedList;
import java.util.List;
class Node {
public int val;
public List<Node> children;
public Node() {
}
public Node(int _val, List<Node> _children) {
val = _val;
children = _children;
}
}
class Solution {
List<Integer> result = new LinkedList<>();
public List<Integer> postorder(Node root) {
if (root == null) {
return result;
}
List<Node> node = root.children;
for (int i = 0; i < node.size(); i++) {
postorder(node.get(i));
}
result.add(root.val);
return result;
}
}
相似题目
参考资料