boost库也提供了和STL一样的字符串处理方法,不过比STL更加丰富,使用boost的字符串处理方法,需要包含头文件:
#include <boost/algorithm/string.hpp>
using namespace boost;
boost库有很多以“i”开头,"_copy"结尾的函数方法,简述如下:
(1) 以“i”开头,表示ignore,忽略。 例如:
istarts_with 方法,表示“忽略大小写以什么开头的字符串”
ifind_all 忽略大小写,查找所有
(2)以“_copy”结尾的方法,表示保存原来的副本,例如:
trim 与 trim_copy
boost的字符串处理,主要有以下方法
1. 字符串拼接
使用join()方法
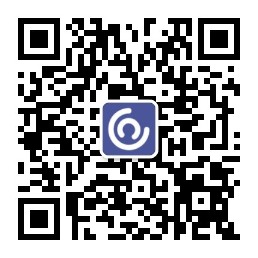
deque<string> d;
d.push_back("stu1");
d.push_back("stu2");
d.push_back("tea1");
d.push_back("tea2");
//调用boost库的 join() 方法进行字符串拼接
string str1 = join(d, "**");
cout << "拼接后的结果是" << str1 << endl;
上述是把序列里分散的字符串用join()方法进行拼接,间隔符号“*”,这里可以任意指定。
如果只想拼接指定的字符串,例如,只想拼接"stu"开头的字符串,该如何做呢?重载方法starts_with()即可。增加一个函数对象,用operator()进行重载。代码如下:
//只拼接stu开头的信息
struct is_stu
{
//操作符重载,判断是否是stu开头
bool operator()(const string &str)
{
return starts_with(str, "stu");
}
};
string str2 = join_if(d, "--", is_stu());
cout << "str2 = " << str2 << endl;
2. 字符串分割
使用 split()方法,
string str3 = "AA**BB*CC-DD";
list<string> slist;
//有多个形同的符号时可能会出现空格
//is_any_of("*-") 以*或-中的任何一个进行分割
split(slist, str3, is_any_of("*-"));
for (auto e : slist)
{
cout << e << endl;
}
字符串中间有特殊的符号进行间隔时,用is_any_of方法进行指定,然后分割,分割的结果放到了list中。
3. 字符串查找
boost对字符串的查找方法很多,例如
查找第一次出现的字符串 find_first
查找最后一次出现的字符串 find_last
查找特定字符第几次出现 find_nth
查找前几个字符 find_head
查找所有中指定的字符 ifind_all
示例代码如下:
//3. 字符串查找
//3.1 查找所有
string str4 = "aaBBccAaWWaa";
deque<string> dq;
ifind_all(dq, str4, "aa");
cout << "查找结果" << endl;
cout << "aa的个数" << dq.size() << endl;
//遍历方式1
for (auto dqElem : dq)
{
cout << dqElem << endl;
}
//遍历方式2
for (BOOST_AUTO(it, dq.begin()); it != dq.end(); it++)
{
cout << *it << endl;
}
// 3.2 查找特定位置,例如第一次出现,最后一次出现,任意位置
string str32 = "Hello, hello,hello,Boost!!!";
iterator_range<string::iterator> rge;
//查找第一次出现的字符串
rge = find_first(str32, "hello");
// rge = ifind_first(str4, "hello");
cout << rge << endl;
//定位出现的位置
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
rge = find_last(str32, "hello"); //查找最后一次出现的字符串
cout << rge << endl;
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
rge = find_nth(str32, "o", 0);//查找第几次出现的字符串
cout << rge << endl;
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
rge = find_head(str32, 4);//查找前4个字符
cout << rge << endl;
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
4. 字符串修剪
例如,去掉两端的空格,去掉特定的字符等。如下是调用trim()方法,去掉字符串两端空格的代码:
//4. 修剪(去掉左右的空格)
string str5 = " Boost ";
cout << "str5 =" << str5 << endl;
trim(str5); //直接修改原字符串
//trim_copy(str5); //不修改原字符串
cout << "修剪后的str5 =" << str5 << endl;
如果不是空格,而是特定的字符呢,例如“*”, “%”等,这种如何去除呢,需要自定义函数对象,重载方法,示例代码如下:
// 定义函数对象,修剪特定的字符
struct is_xing
{
bool operator()(const char &c)
{
return c == '*';
}
};
string str6 = "****China****";
trim_if(str6, is_xing());
cout << "str6 = " << str6 << endl;
5. 替换、删除
这部分比较简单,直接上代码了,如下:
//5. 替换、删除
cout << "-------替换、删除------" << endl;
string str7 = "Hello, hello,hello,Boost!!!";
ireplace_first(str7, "hello", "XXX");//替换第一个,不区分大小写
cout << str7 << endl;
replace_all(str7, "hello", "YYY");//替换全部
cout << str7 << endl;
erase_last(str7, "YYY");//删除最后一个
cout << str7 << endl;
6. 大小写转换
比较简单,直接上代码:
//6. 大小写转换
cout << "大小写转换" << endl;
string str8 = "abc";
to_upper(str8);//改变原字符串
cout << str8 << endl;
string str9 = "AsDf";
to_lower(str9);//改变原字符串
cout << str9 << endl;
string str10 = "Hello Boost";
string retStr = to_upper_copy(str10);//不改变原字符串
cout << str10 << endl;
cout << retStr << endl;
7. 判断式
boost提供了一系列字符串判断的方法,例如是否以指定的字符串开头,结尾,是否包含指定字符等。示例代码如下:
//判断是否以"He, he开头"
cout << starts_with("Hello World", "He") << endl; //大小写敏感
cout << starts_with("Hello World", "he") << endl;
cout << istarts_with("Hello World", "he") << endl; //i忽略大小写
//判断是否以"ld, Ld"结尾
cout << ends_with("Hello World", "ld") << endl;//大小写敏感
cout << iends_with("Hello World", "Ld") << endl;//i忽略大小写
//是否包含"llo"
cout << contains("Hello World", "llo") << endl;//大小写敏感
cout << icontains("Hello World", "LLo") << endl;//i忽略大小写
//内容是否相等
cout << equals("abc", "abc") << endl;//内容是否相等
cout << iequals("abc", "Abc") << endl;//内容是否相等,i忽略大小写
cout << all("Hello", is_lower()) << endl;//判断每一个字符是否全部是小写
cout << all("hello", is_lower()) << endl;//判断每一个字符是否全部是小写
cout << lexicographical_compare("zabc", "xyz") << endl;//字典次序第一个是否小于第二个
cout << "判断式,函数对象" << endl;
cout << is_equal()("abc", "abc") << endl;//内容是否相等,i忽略大小写
is_equal eq;
cout << eq("abc", "abc") << endl;//等价于上面的写法
cout << is_less()("abc", "zbc") << endl;//内容是否小于
cout << is_not_greater()("abc", "zbc") << endl;//内容是否不大于
cout << "分类" << endl;
cout << is_alnum()('1') << endl;//判断是否是字母或数字
cout << is_alnum()('a') << endl;
cout << is_alnum()(',') << endl;
cout << is_alpha()('A') << endl;//是否字母
cout << is_digit()('1') << endl;//是否数字
cout << is_cntrl()('\n') << endl;//是否控制字符
cout << is_any_of("ABCDE")('F') << endl;//是否是ABCDE里面的任何一个
cout << is_xdigit()('Q') << endl;//是否16进制的字符
cout << is_lower()('a') << endl;//是否小写字符
附上一张boost字符串处理的思维导图
关于本节的boost字符串操作的全部示例代码如下:
// boost_03.cpp : 定义控制台应用程序的入口点。
//
/*
boost库字符串操作
*/
#include "stdafx.h"
#include <iostream>
#include <boost/algorithm/string.hpp>
#include <boost/typeof/typeof.hpp>
using namespace std;
using namespace boost;
int main()
{
//1. 字符串合并拼接
deque<string> d;
d.push_back("stu1");
d.push_back("stu2");
d.push_back("tea1");
d.push_back("tea2");
//调用boost库的 join() 方法进行字符串拼接
string str1 = join(d, "**");
cout << "拼接后的结果是" << str1 << endl;
//只拼接stu开头的信息
struct is_stu
{
//操作符重载,判断是否是stu开头
bool operator()(const string &str)
{
return starts_with(str, "stu");
}
};
string str2 = join_if(d, "--", is_stu());
cout << "str2 = " << str2 << endl;
//2. 字符创分割
string str3 = "AA**BB*CC-DD";
list<string> slist;
//有多个形同的符号时可能会出现空格
//is_any_of("*-") 以*或-中的任何一个进行分割
split(slist, str3, is_any_of("*-"));
for (auto e : slist)
{
cout << e << endl;
}
//3. 字符串查找
//3.1 查找所有
string str4 = "aaBBccAaWWaa";
deque<string> dq;
ifind_all(dq, str4, "aa");
cout << "查找结果" << endl;
cout << "aa的个数" << dq.size() << endl;
//遍历方式1
for (auto dqElem : dq)
{
cout << dqElem << endl;
}
//遍历方式2
for (BOOST_AUTO(it, dq.begin()); it != dq.end(); it++)
{
cout << *it << endl;
}
// 3.2 查找特定位置,例如第一次出现,最后一次出现,任意位置
string str32 = "Hello, hello,hello,Boost!!!";
iterator_range<string::iterator> rge;
//查找第一次出现的字符串
rge = find_first(str32, "hello");
// rge = ifind_first(str4, "hello");
cout << rge << endl;
//定位出现的位置
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
rge = find_last(str32, "hello"); //查找最后一次出现的字符串
cout << rge << endl;
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
rge = find_nth(str32, "o", 0);//查找第几次出现的字符串
cout << rge << endl;
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
rge = find_head(str32, 4);//查找前4个字符
cout << rge << endl;
cout << rge.begin() - str32.begin() << "," << rge.end() - str32.begin() << endl;
//4. 修剪(去掉左右的空格)
string str5 = " Boost ";
cout << "str5 =" << str5 << endl;
trim(str5); //直接修改原字符串
//trim_copy(str5); //不修改原字符串
cout << "修剪后的str5 =" << str5 << endl;
// 定义函数对象,修剪特定的字符
struct is_xing
{
bool operator()(const char &c)
{
return c == '*';
}
};
string str6 = "****China****";
trim_if(str6, is_xing());
cout << "str6 = " << str6 << endl;
//5. 替换、删除
cout << "-------替换、删除------" << endl;
string str7 = "Hello, hello,hello,Boost!!!";
ireplace_first(str7, "hello", "XXX");//替换第一个,不区分大小写
cout << str7 << endl;
replace_all(str7, "hello", "YYY");//替换全部
cout << str7 << endl;
erase_last(str7, "YYY");//删除最后一个
cout << str7 << endl;
//6. 大小写转换
cout << "大小写转换" << endl;
string str8 = "abc";
to_upper(str8);//改变原字符串
cout << str8 << endl;
string str9 = "AsDf";
to_lower(str9);//改变原字符串
cout << str9 << endl;
string str10 = "Hello Boost";
string retStr = to_upper_copy(str10);//不改变原字符串
cout << str10 << endl;
cout << retStr << endl;
//7. 判断式,返回bool
cout << "判断式" << endl;
//判断是否以"He, he开头"
cout << starts_with("Hello World", "He") << endl; //大小写敏感
cout << starts_with("Hello World", "he") << endl;
cout << istarts_with("Hello World", "he") << endl; //i忽略大小写
//判断是否以"ld, Ld"结尾
cout << ends_with("Hello World", "ld") << endl;//大小写敏感
cout << iends_with("Hello World", "Ld") << endl;//i忽略大小写
//是否包含"llo"
cout << contains("Hello World", "llo") << endl;//大小写敏感
cout << icontains("Hello World", "LLo") << endl;//i忽略大小写
//内容是否相等
cout << equals("abc", "abc") << endl;//内容是否相等
cout << iequals("abc", "Abc") << endl;//内容是否相等,i忽略大小写
cout << all("Hello", is_lower()) << endl;//判断每一个字符是否全部是小写
cout << all("hello", is_lower()) << endl;//判断每一个字符是否全部是小写
cout << lexicographical_compare("zabc", "xyz") << endl;//字典次序第一个是否小于第二个
cout << "判断式,函数对象" << endl;
cout << is_equal()("abc", "abc") << endl;//内容是否相等,i忽略大小写
is_equal eq;
cout << eq("abc", "abc") << endl;//等价于上面的写法
cout << is_less()("abc", "zbc") << endl;//内容是否小于
cout << is_not_greater()("abc", "zbc") << endl;//内容是否不大于
cout << "分类" << endl;
cout << is_alnum()('1') << endl;//判断是否是字母或数字
cout << is_alnum()('a') << endl;
cout << is_alnum()(',') << endl;
cout << is_alpha()('A') << endl;//是否字母
cout << is_digit()('1') << endl;//是否数字
cout << is_cntrl()('\n') << endl;//是否控制字符
cout << is_any_of("ABCDE")('F') << endl;//是否是ABCDE里面的任何一个
cout << is_xdigit()('Q') << endl;//是否16进制的字符
cout << is_lower()('a') << endl;//是否小写字符
system("pause");
return 0;
}