产生临时变量的三种情况:
以传值的方式给函数传递参数:
拷贝构造函数的参数,如果是: int cTest::add( cTest& A) ;这里就会生成一个临时变量(假的临时变量因为有地址)
改进: 用引用的方式 来传值
#include <iostream>
using namespace std;
class cTest
{
public :
int add(cTest t)
{
m_a = t.m_a;
m_b = t.m_b;
cout << "结果为:" << m_a + m_b << endl;
return m_a + m_b;
}
//拷贝构造函数
cTest(const cTest& t) : m_a(t.m_a), m_b(t.m_b)
{
cout << "调用了拷贝构造函数" << endl;
}
//构造函数
cTest()
{
cout <<"这里调用了构造函数" << endl;
}
//构造函数的重载
cTest( int a , int b)
{
m_a = a;
m_b = b;
cout << "调用了重载的构造函数" << endl;
}
//析构函数
virtual ~cTest()
{
cout << "这里调用了析构函数" << endl;
}
//=操作符重载
cTest & operator= (const cTest& Test)
{
m_a = Test.m_a;
m_b = Test.m_b;
cout << "调用=操作符重载函数" << endl;
}
private:
int m_a;
int m_b;
};
int main()
{
cTest tm(10, 20);//调用了重载的函数
int Sum = tm.add(tm);
system("pause");
}
结果为: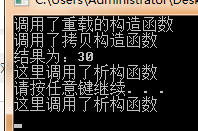
改进后:
类型转换生成的临时对象/ 隐式类型转换以保证函数调用成功
//前提:100为第一个默认参数的值,且没有拷贝构造函数(求成员变量a和b 的和)
cTest ct; //这里会调用构造函数
ct = 100; //这里也会调用构造函数,因为这是一个真正的临时对象(没地址)
//然后:添加一个拷贝构造函数再次,运行上述代码
cTest ct; //这里会调用构造函数
ct = 100; //这里也会调用构造函数,因为这是一个真正的临时对象(没地址)
(1) :用100这个函数创建一个类型为cTest的临时对象
(2):调用拷贝构造函数,把值传送给ct对象
(3):销毁这个临时创建的cTest对象
改进:cTest ct = 100; //只调用一次构造函数 为ct预留空间,用100来构造。
#include <iostream>
using namespace std;
class cTest
{
public:
//构造函数的重载
cTest(int a = 10, int b = 0)
{
m_a = a;
m_b = b;
cout << "调用了重载的构造函数" << endl;
}
//析构函数
virtual ~cTest()
{
cout << "这里调用了析构函数" << endl;
}
public:
//cTest operator= (const cTest & ct)
//{
// m_a = ct.m_a;
// m_b = ct.m_b;
// cout << "调用了=操作符重载函数" << endl;
// return *this;
//}
private:
int m_a;
int m_b;
};
int main()
{
cTest ct;
ct= 100;
system("pause");
}
隐式类型转换以保证函数调用成功
#include <iostream>
using namespace std;
//统计字符ch在字符串srcstring里出现的次数
int calc(const string& srcstring, char ch)
{
const char *p = srcstring.c_str();
int icount = 0;
//........
return icount;
}
int main()
{
char mystr[100] = "I Love China ";
int result = calc(mystr, 'o'); //从char* 到string
//c++语言只会为const引用(const string &srcstring) //产生临时变量。
//不会为 string &srcstring
}
函数返回对象的时候
#include <iostream>
using namespace std;
class cTempValue{
public:
cTempValue(int a = 0, int b = 0)
{
val1 = a; val2 = b;
cout << "调用了构造函数" << endl;
cout << val1 << "和" << val2 << endl;
}
cTempValue(const cTempValue & tm){
cout << "拷贝构造" << endl;
}
virtual ~cTempValue()
{
cout << "析构" << endl;
}
public:
int val1;
int val2;
};
cTempValue Double(cTempValue &ts ) {
cTempValue temp; //这个会消耗一个构造和析构的调用
temp.val1 = ts.val1 * 2;
temp.val2 = ts.val2 * 2;
return temp;//产生临时对象,会有拷贝构造和析构
}
int main()
{
cTempValue test(10,20);
Double(test); //因为返回临时对象,占用一个拷贝构造函数和析构
system("pause");
}
改进:、、
还有一个 类外运算符重载
参考的是:网易云精通c++111417