1.明明想在学校中请一些同学一起做一项问卷调查,为了实验的客观性,他先用计算机生成了N个1到1000之间的随机整数(N≤1000),对于其中重复的数字,只保留一个,把其余相同的数去掉,不同的数对应着不同的学生的学号。然后再把这些数从小到大排序,按照排好的顺序去找同学做调查。请你协助明明完成“去重”与“排序”的工作(同一个测试用例里可能会有多组数据,希望大家能正确处理)。
1.1 分析
去重:Set
排序:TreeSet
多组数据,要使用sc.hasNext()
import java.util.Scanner;
import java.util.TreeSet;
public class Main{
public static void main(String[] args){
Scanner sc=new Scanner(System.in);
while(sc.hasNext()){
int n=sc.nextInt();
int i=0;
TreeSet<Integer> set=new TreeSet<Integer>();
while(i<n){
set.add(sc.nextInt());
i++;
}
while(!set.isEmpty())
System.out.println(set.pollFirst());
}
}
}
2.分割字符串
- 连续输入字符串,请按长度为8拆分每个字符串后输出到新的字符串数组;
- 长度不是8整数倍的字符串请在后面补数字0,空字符串不处理。
2.1 分析
分为三种情况:
- 长度小于8;
- 后面直接append 0 即可
- 等于8
- 直接输出
- 大于8
- 分割
import java.util.Scanner;
import java.util.List;
import java.util.ArrayList;
public class Main{
public static void main(String[] args){
Scanner sc=new Scanner(System.in);
String str1=sc.nextLine();
String str2=sc.nextLine();
getResult(str1);
getResult(str2);
}
public static void getResult(String str){
if(str==null)
return;
int len=str.length();
StringBuffer sb=new StringBuffer(str);
if(len<8){
int i=len;
while(i<8){
sb.append("0");
i++;
}
System.out.println(sb.toString());
}
else if(len==8)
System.out.println(str);
else{
List<String> list=new ArrayList<String>();
int k=0;
for(;k+8<=len;k+=8){
list.add(str.substring(k,k+8));
}
if(k!=len){
StringBuffer sb2=new StringBuffer(str.substring(k));
int num=8-(len-k)%8;
for(k=0;k<num;k++)
sb2.append("0");
list.add(sb2.toString());
}
for(int j=0;j<list.size();j++)
System.out.println(list.get(j));
}
}
}
注:
1.substring(int beginindex) sustring(int beginindex,int endindex)
- beginIndex -- 起始索引(包括), 索引从 0 开始。
- endIndex -- 结束索引(不包括)。
import java.util.Scanner;
public class Main{
public static void main(String[] args){
Scanner sc=new Scanner(System.in);
String str1=sc.nextLine();
String str2=sc.nextLine();
getResult(str1);
getResult(str2);
}
public static void getResult(String str){
while(str.length()>=8){
System.out.println(str.substring(0,8));
str=str.substring(8);
}
if(str.length()<8&&str.length()>0){
str+="00000000";
System.out.println(str.substring(0,8));
}
}
}
3. 写出一个程序,接受一个十六进制的数值字符串,输出该数值的十进制字符串。(多组同时输入 )
import java.util.Scanner;
public class Main{
public static void main(String[] args){
Scanner sc=new Scanner(System.in);
while(sc.hasNextLine()){
String str=sc.nextLine();
getResult(str);
}
}
public static void getResult(String str){
char[] chs=str.toCharArray();
int len=chs.length-1;
int result=0;
int num=0;
for(int i=len;i>1;i--){
switch (chs[i]){
case 'A':
case 'B':
case 'C':
case 'D':
case 'E':
case 'F':
num=chs[i]-'7';
result+=num*((int)Math.pow(16,(len-i)));
break;
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
num=chs[i]-'0';
result+=num*((int)Math.pow(16,(len-i)));
break;
}
}
System.out.println(result);
}
}
注:
1.switch用法
扫描二维码关注公众号,回复:
6148931 查看本文章
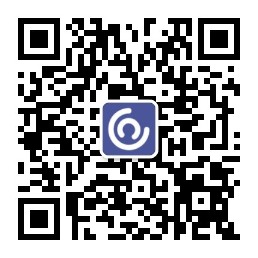
package codeAnal;
public class SwitchDemo {
public static void main(String[] args) {
stringTest();
breakTest();
defautTest();
}
/*
* default不是必须的,也可以不写
* 输出:case two
*/
private static void defautTest() {
char ch = 'A';
switch (ch) {
case 'B':
System.out.println("case one");
break;
case 'A':
System.out.println("case two");
break;
case 'C':
System.out.println("case three");
break;
}
}
/*
* case语句中少写了break,编译不会报错
* 但是会一直执行之后所有case条件下的语句,并不再进行判断,直到default语句
* 下面的代码输出: case two
* case three
*/
private static void breakTest() {
char ch = 'A';
switch (ch) {
case 'B':
System.out.println("case one");
case 'A':
System.out.println("case two");
case 'C':
System.out.println("case three");
default:
break;
}
}
/*
* switch用于判断String类型
* 输出:It's OK!
*/
private static void stringTest() {
String string = new String("hello");
switch (string) {
case "hello":
System.out.println("It's OK!");
break;
default:
System.out.println("ERROR!");
break;
}
}
}
2.ASCII