版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/Jane_yuhui/article/details/82423810
算法是线性时间的:
Partition
头文件 <algorithm>
函数功能: 根据指定的函方法重新排列数组、向量的等。
指定的方法一定是一个一元函数,并且返回值是true : bool pred(const Type &a);
返回值是一个迭代器指向的是排序后的第二个队列的第一个元素 (Iterator to the first element of the second group.)(也可以理解成是排序后的满足条件的队列的队尾)。不过, 这个排序算法可能会改变元素的排列顺序。
示例:
bool IsOdd(int c)
{
return (c%2) == 1;
}
int _tmain(int argc, _TCHAR* argv[])
{
vector<int> vArray;
vArray.push_back(4);
vArray.push_back(5);
vArray.push_back(3);
vArray.push_back(2);
vArray.push_back(3);
vArray.push_back(0);
std::vector<int>::iterator bound;
bound = partition(vArray.begin(), vArray.end(), IsOdd);
cout << "vArray : \n" ;
for (auto n : vArray)
{
std::cout << n << " " ;
}
std::cout << std::endl << "*****************************" << endl;
cout << "Bound : \n" ;
for (vector<int>::iterator it = vArray.begin(); it != bound; it ++)
{
cout << *it << " " ;
}
}
输出结果 :
stable_partition
和 partition 很类似不同的是不会改变数据在队列中的顺序
扫描二维码关注公众号,回复:
6141963 查看本文章
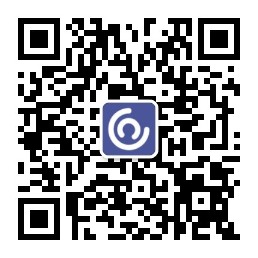
示例:
bool IsOdd(int c)
{
return (c%2) == 1;
}
int _tmain(int argc, _TCHAR* argv[])
{
vector<int> vArray;
vArray.push_back(4);
vArray.push_back(5);
vArray.push_back(3);
vArray.push_back(2);
vArray.push_back(3);
vArray.push_back(0);
auto bound = stable_partition(vArray.begin(), vArray.end(), IsOdd);
cout << "vArray : \n" ;
for (auto n : vArray)
{
std::cout << n << " " ;
}
std::cout << std::endl << "*****************************" << endl;
cout << "Bound : \n" ;
for (vector<int>::iterator it = vArray.begin(); it != bound; it ++)
{
cout << *it << " " ;
}
cout << "\n the last : \n " ;
std::copy(bound, std::end(vArray), std::ostream_iterator<int>(std::cout, " "));
}
结果
nth_element
函数说明
官网示例
#include <iostream>
#include <vector>
#include <algorithm>
#include <functional>
#include <iterator>
int main()
{
std::vector<int> v;
v.push_back(5);
v.push_back(6);
v.push_back(4);
v.push_back(3);
v.push_back(2);
v.push_back(6);
v.push_back(7);
v.push_back(9);
v.push_back(3);
//{5, 6, 4, 3, 2, 6, 7, 9, 3};
std::copy(v.begin(), v.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\nThe median is " << v[v.size()/2] << '\n';
std::cout << "*******************\n";
// 从小到大排序
std::nth_element(v.begin(), v.begin() + v.size()/2, v.end());
std::copy(v.begin(), v.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\nThe median is " << v[v.size()/2] << '\n';
std::cout << "The largest element is " << v[v.size() - 1] << '\n';
std::cout << "*******************\n";
// 从大到小排序
std::nth_element(v.begin(), v.begin()+1, v.end(), std::greater<int>());
std::copy(v.begin(), v.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\nThe second largest element is " << v[1] << '\n';
getchar();
}
结果:
需要随机访问迭代器
Partial_sort
stable_sort