版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/chengqiuming/article/details/89742887
一 点睛
虚函数的作用是允许在派生类中重新定义与基类同名的函数,并且可以通过基类指针或引用来访问基类和派生类中同名函数。
使用虚函数可以使得基类指针访问派生类中的同名函数。
二 实战
1 代码
#include <iostream>
#include <string>
using namespace std;
/*声明基类Box*/
class Box{
public:
Box(int,int,int); //声明构造函数
virtual void display();//声明输出函数
protected: //受保护成员,派生类可以访问
int length,height,width;
};
/*Box类成员函数的实现*/
Box:: Box (int l,int h,int w){//定义构造函数
length =l;
height =h;
width =w;
}
void Box::display(){//定义输出函数
cout<<"length:" << length <<endl;
cout<<"height:" << height <<endl;
cout<<"width:" << width <<endl;
}
/*声明公用派生类FilledBox*/
class FilledBox : public Box{
public:
FilledBox (int, int, int, int, string);//声明构造函数
virtual void display();//虚函数
private:
int weight;//重量
string fruit;//装着的水果
};
/* FilledBox类成员函数的实现*/
void FilledBox :: display(){//定义输出函数
cout<<"length:"<< length <<endl;
cout<<"height:"<< height <<endl;
cout<<"width:"<< width <<endl;
cout<<"weight:"<< weight <<endl;
cout<<"fruit:"<< fruit <<endl;
}
FilledBox:: FilledBox (int l, int h, int w, int we, string f ) : Box(l,h,w), weight(we), fruit(f){}
int main(){//主函数
Box box(1,2,3);//定义Box类对象box
FilledBox fbox(2,3,4,5,"apple");//定义FilledBox类对象fbox
Box *pt = &box;//定义指向基类对象的指针变量pt
pt->display( );
pt = &fbox;
pt->display( );
return 0;
}
2 运行
[root@localhost charpter02]# g++ 0229.cpp -o 0229
[root@localhost charpter02]# ./0229
length:1
height:2
width:3
length:2
height:3
width:4
weight:5
fruit:apple
3 说明
该实例中将基类Box中的成员函数display定义为虚函数,就能使得基类对象的指针变量既可以访问基类的成员函数display,也可以访问派生类的成员函数display。
pt是基类的指针,它可以调用同一类族中不同类的虚函数。这就是多态,即对同一消息,不同对象有不同的响应方式。
如果基类中的display函数不是虚函数,是无法通过基类指针去调用派生类对象中的成员函数的。虚函数突破了这一限制,在派生类的基类部分中,派生类的虚函数取代了基类原来的虚函数,因此在使用基类指针指向派生类对象后,调用虚函数时就调用了派生类的虚函数。需要注意,只有用virtual声明了虚函数后才具有以上作用,如果不声明虚函数,企图通过基类指针调用派生类的非虚函数是不行的。
虚函数实现了同一类族中不同类对象可以对同一函数调用作出不同响应的动态多态性。
扫描二维码关注公众号,回复:
6093614 查看本文章
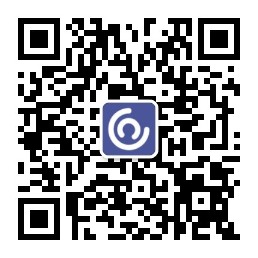