spring boot 配置文件:
默认会生成一个配置文件 src/main/resources 目录下的application.properties配置文件
作用:spring boot 自动配置(约定:8080) 可以使用配置文件,对默认的配置进行修改
默认的全局配置文件:
application.properties : 语法 : k=v
application.yml : YAML Ain't Markup Language (“YAML不是一种标记语言”的外语缩写)
xml 是一个标记语言
<server>
<port>8881</port>
<path>/home/xxx</path>
</server>
yml 语法实现:
server:
port: 8881
path: /home/xxx
1、即:k:空格v
2、通过垂直对齐
扫描二维码关注公众号,回复:
6083238 查看本文章
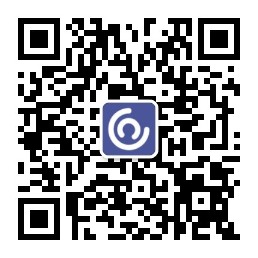
3、默认不需要加单/双引号 ,但如果值里面有转义符时,使用双引号。
yml各种类型赋值:
新建类People:
@ConfigurationProperties(prefix="people") : 为实例注入属性值
@Component
@ConfigurationProperties(prefix="people")
public class People {
private String name;
private int age;
private int sex;
private Date birthday;
/*
* {province:湖南,city:郴州}
* */
private Map<String, Object> location;
private String[] hobbies[];
private List<String> skills;
private Pet pet;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getSex() {
return sex;
}
public void setSex(int sex) {
this.sex = sex;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public Map<String, Object> getLocation() {
return location;
}
public void setLocation(Map<String, Object> location) {
this.location = location;
}
public Pet getPet() {
return pet;
}
public void setPet(Pet pet) {
this.pet = pet;
}
public String[][] getHobbies() {
return hobbies;
}
public void setHobbies(String[][] hobbies) {
this.hobbies = hobbies;
}
public List<String> getSkills() {
return skills;
}
public void setSkills(List<String> skills) {
this.skills = skills;
}
@Override
public String toString() {
return "People [name=" + name + ", age=" + age + ", sex=" + sex + ", birthday=" + birthday + ", location="
+ location + ", hobbies=" + Arrays.toString(hobbies) + ", skills=" + skills + ", pet=" + pet + "]";
}
}
以及子类 Pet:
public class Pet {
private String name;
private String type;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public String toString() {
return "Pet [name=" + name + ", type=" + type + "]";
}
}
application.yml 配置如下:
server:
port: 8881
people:
name: feifei
age: 19
sex: 0
birthday: 2010/01/05
location: #{province: 湖南,city: 郴州}
province: 湖南
city: 郴州
hobbies: [游泳,轮滑]
#-游泳
#-轮滑
skills: [编程,金融]
#-编程
#-金融
pet:
name: zuzu
type: dog
测试类:
@RunWith(SpringRunner.class)
@SpringBootTest
public class FirstWarProjApplicationTests {
@Autowired
People people;
@Test
public void contextLoads() {
System.out.print(people);
}
}
执行 Run As -> JUnit Test,输出结果 :
People [name=feifei, age=19, sex=0, birthday=Tue Jan 05 00:00:00 CST 2010, location={province=湖南, city=郴州}, hobbies=[[Ljava.lang.String;@9255c05, [Ljava.lang.String;@5da7cee2], skills=[编程, 金融], pet=Pet [name=zuzu, type=dog]]