1、属性驱动
(1)官方推荐,现实中不常用,不推荐
(2)使用方法:在action中添加属性(要写get、set方法),属性名和页面提交参数的name值一样,一个name对应一个属性
(3)优点:
- 可以自动完成类型转换(只能转换八大数据类型和包装类)
- 支持特定字符串转换为Date,例如:yyyy-MM-dd
(4)servlet不可以使用属性获得参数
原因:
- servlet是线程不安全的,一个servlet只会创建一个实例,如果十个人同时访问,使用属性就有问题了
- servlet参数是在方法中接收的,就算十个人同时访问,也木有问题
(5)为什么action是线程安全的?
- 一个请求会创建一个action,不存在并发
- 验证方法:为action写一个构造方法,里面打印一句话,会发现每次访问,都会打印一句话
jsp:
<form action="${pageContext.request.contextPath }/getValue_add" method="post">
姓名:<input type="text" name="name"/><br/>
密码:<input type="password" name="password"/><br/>
年龄:<input type="text" name="age"/><br/>
生日:<input type="text" name="birthday"/><br/>
<input type="submit" value="提交" /><br/>
</form>
action:
//属性驱动
public class GetValue extends ActionSupport {
private String name;
private String password;
private Integer age;
private Date birthday;
public String add() {
System.out.println("name:"+name);
System.out.println("password:"+password);
System.out.println("age:"+age);
System.out.println("birthday:"+birthday);
return "success";
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
}
控制台:
2、对象驱动
- 使用方法:在action中添加一个对象的属性(要写set、get方法),页面中name值必须加属性名前缀,如:name=user.name
- 优点:直接把参数封装到对象中
jsp:
扫描二维码关注公众号,回复:
6025506 查看本文章
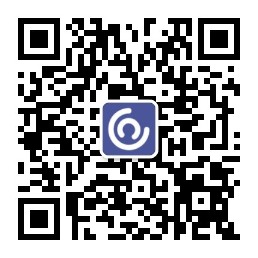
<form action="${pageContext.request.contextPath }/getValue2_execute" method="post">
姓名:<input type="text" name="user.name"/><br/>
密码:<input type="password" name="user.password"/><br/>
年龄:<input type="text" name="user.age"/><br/>
生日:<input type="text" name="user.birthday"/><br/>
<input type="submit" value="提交" /><br/>
</form>
action:
//对象驱动
public class GetValue2 extends ActionSupport {
public User user;
public String execute() {
System.out.println(user.toString());
return SUCCESS;
}
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
}
3、模型驱动
(1)使用方法:
- 实现一个ModelDriven<T>接口
- 在action中准备一个成员变量(不用写get、set方法),注意new一个对象
- 实现一个getModel方法,返回成员变量
(2)优点:直接把参数封装到对象中,不用像对象驱动一样在页面修改name的值
(3)缺点:只能接受一个对象的参数
jsp:
<form action="${pageContext.request.contextPath }/getValue3_execute" method="post">
姓名:<input type="text" name="name"/><br/>
密码:<input type="password" name="password"/><br/>
年龄:<input type="text" name="age"/><br/>
生日:<input type="text" name="birthday"/><br/>
<input type="submit" value="提交" /><br/>
</form>
action:
public class GetValue3 extends ActionSupport implements ModelDriven<User> {
public User user = new User();
public String execute() {
System.out.println(user.toString());
return SUCCESS;
}
@Override
public User getModel() {
// TODO Auto-generated method stub
return user;
}
}
4、封装集合类型参数
使用方法和对象驱动一样,只是在页面中不一样
(1)list:
前端页面中可以写
name="list"
name="list[5]",指定索引,会封装到指定索引中
jsp:
<form action="${pageContext.request.contextPath }/getValue4_execute" method="post">
姓名:<input type="text" name="list"/><br/>
密码:<input type="password" name="list[2]"/><br/>
年龄:<input type="text" name="list[1]"/><br/>
生日:<input type="text" name="list[3]"/><br/>
<input type="submit" value="提交" /><br/>
</form>
action:
//封装集合对象
public class GetValue4 extends ActionSupport {
public List<String> list;
public String execute() {
System.out.println(list);
return SUCCESS;
}
public List<String> getList() {
return list;
}
public void setList(List<String> list) {
this.list = list;
}
}
运行结果,控制台:
(2)map:
前端页面中要指定key值;name="map['haha']"
jsp:
<form action="${pageContext.request.contextPath }/getValue5_execute" method="post">
姓名:<input type="text" name="map['name']"/><br/>
密码:<input type="password" name="map['password']"/><br/>
年龄:<input type="text" name="map['age']"/><br/>
生日:<input type="text" name="map['birthday']"/><br/>
<input type="submit" value="提交" /><br/>
</form>
action:
//封装集合对象map
public class GetValue5 extends ActionSupport {
public Map<String, String> map;
public String execute() {
System.out.println(map);
return SUCCESS;
}
public Map<String, String> getMap() {
return map;
}
public void setMap(Map<String, String> map) {
this.map = map;
}
}
注意:重定向return命名规则to+页面名:例return "tolist";