概述
- 在软件开发中,我们经常需要使用聚合对象来存储一系列数据。聚合对象拥有两个职责:一是存储数据;二是遍历数据。从依赖性来看,前者是聚合对象的基本职责;而后者既是可变化的,又是可分离的。因此,可以将遍历数据的行为从聚合对象中分离出来,封装在一个被称之为“迭代器”的对象中,由迭代器来提供遍历聚合对象内部数据的行为,这将简化聚合对象的设计,更符合“单一职责原则”的要求。
- 定义:提供一种方法访问一个容器(container)对象中各元素,而又不需暴露该对象的内部细节。
- 又叫做游标(Cursor)模式。
- 由客户程序来控制遍历的进程,被称为外部迭代器;还有一种实现方式便是由迭代器自身来控制迭代,被称为内部迭代器。
- 迭代器模式是一种对象行为型模式。
- 学习难度:★★★☆☆
- 使用频率:★★★★★
优缺点
类图
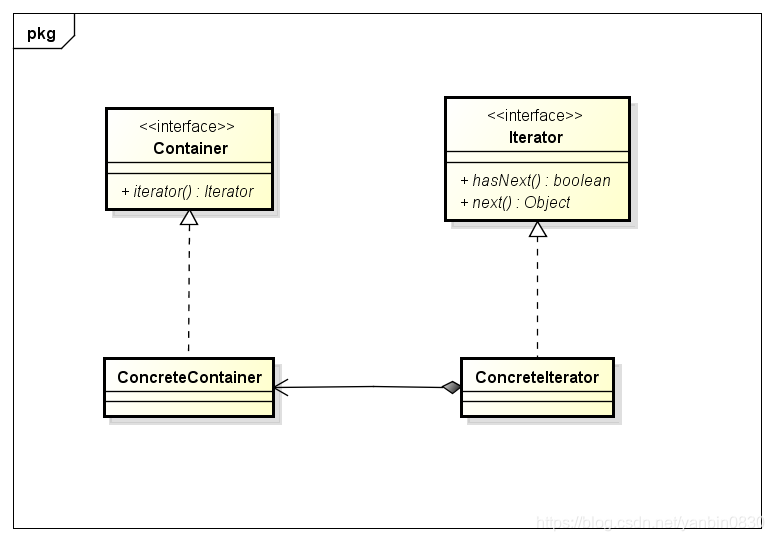
组成角色
- 迭代器角色(Iterator)
- 具体迭代器角色(ConcreteIterator)
- 容器角色(Container)
- 具体容器角色(ConcreteContainer)
Code Example
迭代器角色(Iterator)
public interface Iterator {
public boolean hasNext();
public Object next();
}
具体迭代器角色(ConcreteIterator)
public class BallIterator implements Iterator {
private BallContainer ballContainer;
private int index;
public BallIterator(BallContainer ballContainer) {
this.ballContainer = ballContainer;
this.index = 0;
}
public boolean hasNext() {
if (index < ballContainer.getCount()) {
return true;
} else {
return false;
}
}
public Object next() {
Ball ball = ballContainer.getBallAt(index);
index++;
return ball;
}
}
容器角色(Container)
public interface Container {
public Iterator iterator();
}
具体容器角色(ConcreteContainer)
public class Ball {
private String ballColor;
public Ball(String ballColor) {
this.ballColor = ballColor;
}
public String getBallColor() {
return this.ballColor;
}
}
public class BallContainer implements Container {
private ArrayList<Ball> balls;
public BallContainer() {
balls = new ArrayList<Ball>();
}
public Ball getBallAt(int index) {
return balls.get(index);
}
public void appendBall(Ball ball) {
balls.add(ball);
}
public int getCount() {
return balls.size();
}
public ArrayList<Ball> getBalls() {
return this.balls;
}
public Iterator iterator() {
return new BallIterator(this);
}
}
客户端
public class IteratorPattern {
public static void main(String args[]) {
BallContainer ballContainer = new BallContainer();
ballContainer.appendBall(new Ball("red"));
ballContainer.appendBall(new Ball("blue"));
ballContainer.appendBall(new Ball("yellow"));
Iterator ite = ballContainer.iterator();
while (ite.hasNext()) {
Ball ball = (Ball) ite.next();
System.out.println(ball.getBallColor());
}
}
}