139 | 34.3% | Medium |
139. Word Break
Medium
1867107FavoriteShare
Given a non-empty string s and a dictionary wordDict containing a list of non-empty words, determine if s can be segmented into a space-separated sequence of one or more dictionary words.
Note:
- The same word in the dictionary may be reused multiple times in the segmentation.
- You may assume the dictionary does not contain duplicate words.
Example 1:
Input: s = "leetcode", wordDict = ["leet", "code"] Output: true Explanation: Return true because"leetcode"
can be segmented as"leet code"
.
Example 2:
Input: s = "applepenapple", wordDict = ["apple", "pen"] Output: true Explanation: Return true because"
applepenapple"
can be segmented as"
apple pen apple"
. Note that you are allowed to reuse a dictionary word.
Example 3:
Input: s = "catsandog", wordDict = ["cats", "dog", "sand", "and", "cat"]
Output: false
Accepted
303,378
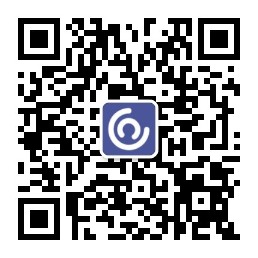
Submissions
883,755
DP+暴力
class Solution {
public:
bool dp[100001];
bool wordBreak(string s, vector<string>& wordDict) {
//dp[i]=可以由dict拼接出前i个字符
s="0"+s;
string t;
memset(dp,0,sizeof(dp));
dp[0]=1;
for(int i=0;i<=s.length();i++) //n
{
if(dp[i]==0)
continue;
for(int j=0;j<wordDict.size();j++) // size
{
if(s.substr(i+1,wordDict[j].length())==wordDict[j]) // m
{
dp[i+wordDict[j].length()]=1;
}
}
}
return dp[s.length()-1];
}
};
6 | 30.7% | Medium |
6. ZigZag Conversion
Medium
9032832FavoriteShare
The string "PAYPALISHIRING"
is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)
P A H N
A P L S I I G
Y I R
And then read line by line: "PAHNAPLSIIGYIR"
Write the code that will take a string and make this conversion given a number of rows:
string convert(string s, int numRows);
Example 1:
Input: s = "PAYPALISHIRING", numRows = 3
Output: "PAHNAPLSIIGYIR"
Example 2:
Input: s = "PAYPALISHIRING", numRows = 4
Output: "PINALSIGYAHRPI"
Explanation:
P I N
A L S I G
Y A H R
P I
Accepted
288,258
Submissions
939,295
最容易想到的:使用vector或string压缩列间距即可,注意numRows==1特判。后面还有更优的方法。
class Solution {
public:
string convert(string s, int numRows) {
if(numRows==1)
{
return s; //这里很重要
}
vector<char>mp[1000];
for(int i=0;i<=numRows;i++)
mp[i].clear();
int y,d;
y=0;
d=1;
for(int i=0;i<s.length();i++)
{
mp[y].push_back(s[i]);
y+=d;
if(y>=numRows)
{
y-=2;
d=-1;
}
else if(y<0)
{
y+=2;
d=1;
}
}
string ret="";
for(int i=0;i<numRows;i++)
{
for(int j=0;j<mp[i].size();j++)
{
ret=ret+mp[i][j];
}
}
return ret;
}
};
当然,,更优的方法就是找规律,然后省略掉模拟的过程和空间。(就是麻烦点)
class Solution {
public:
string convert(string s, int numRows) {
if(numRows==1)
{
return s; //这里很重要
}
int cicle=numRows*2-2;
string ret("");
for(int i=0;i<s.length();) // 第一行
{
ret=ret+s[i];
i+=cicle;
}
//中间
int idx[2];
int t;
for(int i=1;i<=numRows-2;i++)
{
idx[0]=i;
idx[1]=numRows-1+(numRows-1-i);
for(int j=0;;j++)
{
t=j/2*cicle+idx[j&1];
if(t>=s.length())
break;
ret=ret+s[t];
}
}
for(int i=numRows-1;i<s.length();) //最后一行
{
ret=ret+s[i];
i+=cicle;
}
return ret;
}
};