21.栈的压入,弹出
按照压入数组压入栈,每次对最顶的元素进行能判断,如果等于弹出数组当中的数值,则弹出,弹出数组的指针向后移动一位,当弹出数组的所有元素弹出后,如果栈为空,则说明相匹配。
import java.util.ArrayList;
import java.util.*;
public class Solution {
public boolean IsPopOrder(int [] pushA,int [] popA) {
if(pushA.length==0||popA.length==0){
return false;
}
Stack<Integer> st = new Stack();
int j =0;
for(int x :pushA){
st.push(x);
while(!st.isEmpty()&&st.peek()==popA[j]){
st.pop();
j++;
}
}
if(st.isEmpty()){
return true;
}else{
return false;
}
}
}
22.从上到下打印树
树的层序遍历,需要借助一个队列来实现。
import java.util.ArrayList;
import java.util.*;
/**
public class TreeNode {
int val = 0;
TreeNode left = null;
TreeNode right = null;
public TreeNode(int val) {
this.val = val;
}
}
*/
public class Solution {
public ArrayList<Integer> PrintFromTopToBottom(TreeNode root) {
Queue<TreeNode> qu = new LinkedList();
ArrayList<Integer> res = new ArrayList();
if(root==null){
return res;
}
qu.add(root);
while(!qu.isEmpty()){
int count = qu.size();
while(count>0){
TreeNode temp = qu.remove();
res.add(temp.val);
if(temp.left!=null){
qu.add(temp.left);
}
if(temp.right!=null){
qu.add(temp.right);
}
count--;
}
}
return res;
}
}
23.二差搜索树的后序遍历序列
二叉搜索树的特点,父节点的值大于左右节点,且左子树的值小于右子树,借助这个特性进行递归判断。
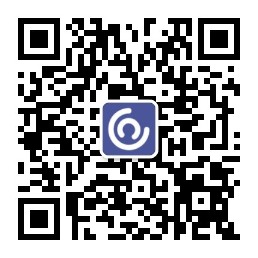
public class Solution {
public boolean VerifySquenceOfBST(int [] sequence) {
if(sequence.length==0){
return false;
}
int len = sequence.length-1;
return test(sequence,0,len);
}
public boolean test(int []num,int start,int end){
if(start>end){
return true;
}
int root = num[end];
int i = start;
while(num[i]<root){
i++;
}
int j = i;
while(j<end){
if(num[j]<root){
return false;
}
j++;
}
boolean left = test(num,start,i-1);
boolean right =test(num,i,end-1);
return left&&right;
}
}
24.二叉树中和为某一值的路径
注意如果的路径不符合,需要进行回退。即最后一步。
public class Solution {
ArrayList<Integer> arr = new ArrayList();
ArrayList<ArrayList<Integer>> res = new ArrayList();
public ArrayList<ArrayList<Integer>> FindPath(TreeNode root,int target) {
if(root==null){
return res;
}
arr.add(root.val);
target = target-root.val;
if(target==0&&root.left==null&&root.right==null){
res.add(new ArrayList<Integer>(arr));
}
FindPath(root.left,target);
FindPath(root.right,target);
arr.remove(arr.size()-1);
return res;
}
}
25.复杂链表的复制
思路借鉴了一个评论区大佬,总共分为三个步骤:
遍历链表,复制每个结点,如复制结点A得到A1,将结点A1插到结点A后面;
重新遍历链表,复制老结点的随机指针给新结点,如A1.random = A.random.next;
拆分链表,将链表拆分为原链表和复制后的链表
public class Solution {
public RandomListNode Clone(RandomListNode pHead)
{
if (pHead==null){
return null;
}
RandomListNode cur = pHead;
while(cur!=null){
RandomListNode cl= new RandomListNode(cur.label);
RandomListNode next = cur.next;
cur.next = cl;
cl.next = next;
cur = next;
}
cur = pHead;
while(cur!=null){
cur.next.random = cur.random==null?null:cur.random.next;
cur = cur.next.next;
}
cur = pHead;
RandomListNode pcl = pHead.next;
while(cur!=null){
RandomListNode clnode = cur.next;
cur.next = clnode.next;
clnode.next = clnode.next==null?null:clnode.next.next;
cur = cur.next;
}
return pcl;
}
}