今日学习的是http编程续
我不是特别懂老师,今天说我昨天学习的内容是老版本的知识了,现在几乎不使用了,现在有更好的包了,有点头大。
今天学习的是jdk9才开始自带的包HttpClient 和apache 的包 HttpComponents
相比较之下我更喜欢HttpComponents包 我感觉这个更简单易懂一点
但是今日学习还是只是有点懵懂 加油吧
我都只学到了他们的GET 和 POST 获取http信息 和 输入数据
相对来说POST 要比 GET难 ,不是特别懂那个&符号
HttpComponents包是第三方包 需要去下载
但是我们在之前学习了maven 可以自动下载
建立一个maven项目 在他的pom.xml文件里写入第三方包的依赖文件代码就可以自动下载了
附今日代码:
扫描二维码关注公众号,回复:
5914498 查看本文章
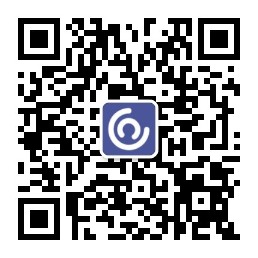
1 import java.io.IOException; 2 3 import org.apache.http.HttpEntity; 4 import org.apache.http.HttpResponse; 5 import org.apache.http.client.ClientProtocolException; 6 import org.apache.http.client.ResponseHandler; 7 import org.apache.http.client.config.RequestConfig; 8 import org.apache.http.client.methods.HttpGet; 9 import org.apache.http.impl.client.CloseableHttpClient; 10 import org.apache.http.impl.client.HttpClients; 11 import org.apache.http.util.EntityUtils; 12 13 public class HttpComponentsGetTest { 14 15 public final static void main(String[] args) throws Exception { 16 17 CloseableHttpClient httpClient = HttpClients.createDefault(); 18 RequestConfig requestConfig = RequestConfig.custom() 19 .setConnectTimeout(5000) //设置连接超时时间 20 .setConnectionRequestTimeout(5000) // 设置请求超时时间 21 .setSocketTimeout(5000) 22 .setRedirectsEnabled(true)//默认允许自动重定向 23 .build(); 24 //设置需要获取信息的URL 25 HttpGet httpGet = new HttpGet("http://www.baidu.com"); 26 //将需要获取信息的配置设置给HttpGet对象 27 httpGet.setConfig(requestConfig); 28 //定义字符串用来存放返回结果 29 String srtResult = ""; 30 try { 31 //将httpClient执行execute方法 将返回的结果给Response 32 HttpResponse httpResponse = httpClient.execute(httpGet); 33 //判断返回的代码 200 代表成功 404 代表未找到 500代表服务器问题 34 if(httpResponse.getStatusLine().getStatusCode() == 200){ 35 //将返回结果放入字符串 36 srtResult = EntityUtils.toString(httpResponse.getEntity(), "UTF-8");//获得返回的结果 37 System.out.println(srtResult); 38 }else 39 { 40 //异常处理 41 } 42 } catch (IOException e) { 43 e.printStackTrace(); 44 }finally { 45 try { 46 httpClient.close(); 47 } catch (IOException e) { 48 e.printStackTrace(); 49 } 50 } 51 } 52 }
1 import java.io.IOException; 2 import java.net.URLEncoder; 3 import java.util.ArrayList; 4 import java.util.List; 5 6 import org.apache.http.HttpResponse; 7 import org.apache.http.client.config.RequestConfig; 8 import org.apache.http.client.entity.UrlEncodedFormEntity; 9 import org.apache.http.client.methods.HttpPost; 10 import org.apache.http.impl.client.CloseableHttpClient; 11 import org.apache.http.impl.client.HttpClientBuilder; 12 import org.apache.http.impl.client.LaxRedirectStrategy; 13 import org.apache.http.message.BasicNameValuePair; 14 import org.apache.http.util.EntityUtils; 15 16 public class HttpComponentsPostTest { 17 18 public final static void main(String[] args) throws Exception { 19 20 //获取可关闭的 httpCilent 21 //CloseableHttpClient httpClient = HttpClients.createDefault(); 22 CloseableHttpClient httpClient = HttpClientBuilder.create().setRedirectStrategy(new LaxRedirectStrategy()).build(); 23 //配置超时时间 24 RequestConfig requestConfig = RequestConfig.custom(). 25 setConnectTimeout(10000).setConnectionRequestTimeout(10000) 26 .setSocketTimeout(10000).setRedirectsEnabled(false).build(); 27 28 HttpPost httpPost = new HttpPost("https://tools.usps.com/go/ZipLookupAction.action"); 29 //设置超时时间 30 httpPost.setConfig(requestConfig); 31 32 //装配post请求参数 33 List<BasicNameValuePair> list = new ArrayList<BasicNameValuePair>(); 34 list.add(new BasicNameValuePair("tAddress", URLEncoder.encode("1 Market Street", "UTF-8"))); //请求参数 35 list.add(new BasicNameValuePair("tCity", URLEncoder.encode("San Francisco", "UTF-8"))); //请求参数 36 list.add(new BasicNameValuePair("sState", "CA")); //请求参数 37 try { 38 UrlEncodedFormEntity entity = new UrlEncodedFormEntity(list,"UTF-8"); 39 //设置post求情参数 40 httpPost.setEntity(entity); 41 httpPost.setHeader("User-Agent", "HTTPie/0.9.2"); 42 //httpPost.setHeader("Content-Type","application/form-data"); 43 HttpResponse httpResponse = httpClient.execute(httpPost); 44 String strResult = ""; 45 if(httpResponse != null){ 46 System.out.println(httpResponse.getStatusLine().getStatusCode()); 47 if (httpResponse.getStatusLine().getStatusCode() == 200) { 48 strResult = EntityUtils.toString(httpResponse.getEntity()); 49 } 50 else { 51 strResult = "Error Response: " + httpResponse.getStatusLine().toString(); 52 } 53 }else{ 54 55 } 56 System.out.println(strResult); 57 } catch (Exception e) { 58 e.printStackTrace(); 59 }finally { 60 try { 61 if(httpClient != null){ 62 httpClient.close(); //释放资源 63 } 64 } catch (IOException e) { 65 e.printStackTrace(); 66 } 67 } 68 } 69 }
1 import java.io.IOException; 2 import java.net.URI; 3 import java.net.URLEncoder; 4 import java.net.http.HttpClient; 5 import java.net.http.HttpRequest; 6 import java.net.http.HttpResponse; 7 import java.nio.charset.Charset; 8 9 public class JDKHttpClientGetTest { 10 11 public static void main(String[] args) throws IOException, InterruptedException { 12 doGet(); 13 } 14 15 public static void doGet() { 16 try{ 17 HttpClient client = HttpClient.newHttpClient(); 18 HttpRequest request = HttpRequest.newBuilder(URI.create("http://www.baidu.com")).build(); 19 HttpResponse response = client.send(request, HttpResponse.BodyHandlers.ofString()); 20 System.out.println(response.body()); 21 } 22 catch(Exception e) { 23 e.printStackTrace(); 24 } 25 } 26 }
1 import java.io.IOException; 2 import java.net.URI; 3 import java.net.URLEncoder; 4 import java.net.http.HttpClient; 5 import java.net.http.HttpRequest; 6 import java.net.http.HttpResponse; 7 8 public class JDKHttpClientPostTest { 9 10 public static void main(String[] args) throws IOException, InterruptedException { 11 doPost(); 12 } 13 14 public static void doPost() { 15 try { 16 HttpClient client = HttpClient.newBuilder().build(); 17 HttpRequest request = HttpRequest.newBuilder() 18 .uri(URI.create("https://tools.usps.com/go/ZipLookupAction.action")) 19 //.header("Content-Type","application/x-www-form-urlencoded") 20 .header("User-Agent", "HTTPie/0.9.2") 21 .header("Content-Type","application/x-www-form-urlencoded;charset=utf-8") 22 //.method("POST", HttpRequest.BodyPublishers.ofString("tAddress=1 Market Street&tCity=San Francisco&sState=CA")) 23 //.version(Version.HTTP_1_1) 24 .POST(HttpRequest.BodyPublishers.ofString("tAddress=" 25 + URLEncoder.encode("1 Market Street", "UTF-8") 26 + "&tCity=" + URLEncoder.encode("San Francisco", "UTF-8") + "&sState=CA")) 27 //.POST(HttpRequest.BodyPublishers.ofString("tAddress=" + URLEncoder.encode("1 Market Street", "UTF-8") + "&tCity=" + URLEncoder.encode("San Francisco", "UTF-8") + "&sState=CA")) 28 .build(); 29 HttpResponse response = client.send(request, HttpResponse.BodyHandlers.ofString()); 30 System.out.println(response.statusCode()); 31 System.out.println(response.headers()); 32 System.out.println(response.body().toString()); 33 34 } 35 catch(Exception e) { 36 e.printStackTrace(); 37 } 38 } 39 }