0.版本选择
我这里选择了5.6.x,记得如果spring-boot-starter-parent是1.x可以选择2.x版本的elasticsearch,版本要对应,不然会有莫名其妙的问题
1.安装ElasticSearch
https://www.elastic.co/downloads/past-releases
windows 测试的,解压就能用
解压,到bin目录,双击elasticsearch.bat
1.1安装elasticsearch-head
https://github.com/mobz/elasticsearch-head
elasticsearch-head是一个网页查看elasticsearch状态,操作的第三方东西
git clone git://github.com/mobz/elasticsearch-head.git
cd elasticsearch-head
npm install
npm run start
open http://localhost:9100/
要先安装node.js
config/elasticsearch.yml 文件增加
http.cors.enabled: true
http.cors.allow-origin: “*”
elasticsearch-head/Gruntfile.js
connect: {
server: {
options: {
hostname: '0.0.0.0',
port: 9100,
base: '.',
keepalive: true
}
}
}
8082改成你自己的端口
http://127.0.0.1:8082/swagger-ui.html#/
0是第一页
扫描二维码关注公众号,回复:
5909218 查看本文章
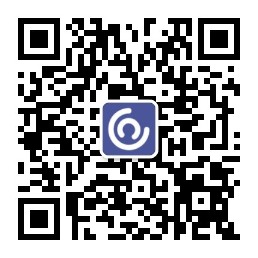
application.properties
#===es start===
spring.data.elasticsearch.repositories.enabled = true
spring.data.elasticsearch.cluster-nodes = 127.0.0.1:9300
#===es end===
2.porm
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.1.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<packaging>jar</packaging>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<!--Swagger-UI API文档生产工具-->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.6.1</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.6.1</version>
</dependency>
<!--Swagger-UI API文档生产工具-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
主要添加spring-boot-starter-data-elasticsearch,注意spring-boot-starter-parent的版本号
3. GoodsInfo
package com.example.demo.domain;
import org.springframework.data.elasticsearch.annotations.Document;
import java.io.Serializable;
@Document(indexName = "testgoods", type = "goods")
public class GoodsInfo implements Serializable {
private Long id;
private String name;
private String description;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public GoodsInfo(Long id, String name, String description) {
this.id = id;
this.name = name;
this.description = description;
}
public GoodsInfo() {
}
}
indexName 类似数据库名称,type类似表名字
4. GoodsRepository
package com.example.demo.repository;
import com.example.demo.domain.GoodsInfo;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
import org.springframework.stereotype.Component;
@Component
public interface GoodsRepository extends ElasticsearchRepository<GoodsInfo, Long> {
}
这里会帮你封装了很多了
5. HelloWorldController
package com.example.demo.controller;
import com.example.demo.domain.GoodsInfo;
import com.example.demo.repository.GoodsRepository;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiOperation;
import org.elasticsearch.index.query.QueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.index.query.functionscore.FunctionScoreQueryBuilder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.elasticsearch.core.query.NativeSearchQuery;
import org.springframework.data.elasticsearch.core.query.NativeSearchQueryBuilder;
import org.springframework.data.elasticsearch.core.query.SearchQuery;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.Optional;
import static org.elasticsearch.index.query.QueryBuilders.queryStringQuery;
@Controller
@Api(value = "HelloWorldController|一个用来测试swagger注解的控制器",tags = "HelloWorldController", description = "HelloWorldController")
public class HelloWorldController {
@Autowired
private GoodsRepository goodsRepository;
@ResponseBody
@RequestMapping(value = "/hello", method = RequestMethod.GET)
@ApiOperation(value = "根据用户编号获取用户姓名", notes = "test: 仅1和2有正确返回")
@ApiImplicitParam(paramType="query", name = "userNumber", value = "用户编号", required = true, dataType = "Integer")
public String index(@RequestParam Integer userNumber){
if(userNumber == 1){
return "小白";
}
else if(userNumber == 2){
return "小红";
}
else{
return "未知";
}
}
@ResponseBody
@RequestMapping(value = "/save", method = RequestMethod.POST)
@ApiOperation(value = "新增商品")
public String save(){
GoodsInfo goodsInfo = new GoodsInfo(System.currentTimeMillis(), "商品" + System.currentTimeMillis(), "这是一个测试商品");
goodsRepository.save(goodsInfo);
return "success";
}
@ResponseBody
@RequestMapping(value = "/delete", method = RequestMethod.POST)
@ApiOperation(value = "删除商品")
@ApiImplicitParam(paramType="query", name = "id", value = "商品id", required = true, dataType = "long")
public String delete(@RequestParam(required = true) long id){
goodsRepository.deleteById(id);
return "success";
}
@ResponseBody
@RequestMapping(value = "/update", method = RequestMethod.POST)
@ApiOperation(value = "更新商品")
@ApiImplicitParam(paramType="query", name = "id", value = "商品id", required = true, dataType = "long")
public String update(@RequestParam(required = true) long id,
@RequestParam(required = false) String name,
@RequestParam(required = false) String description){
Optional<GoodsInfo> goodsInfo = goodsRepository.findById(id);
if(goodsInfo.isPresent()){
if(name != null){
goodsInfo.get().setName(name);
}
if(description != null){
goodsInfo.get().setDescription(description);
}
goodsRepository.save(goodsInfo.get());
return "success";
}else{
return "no find";
}
}
@ResponseBody
@RequestMapping(value = "/getOne", method = RequestMethod.GET)
@ApiOperation(value = "得到一个商品")
@ApiImplicitParam(paramType="query", name = "id", value = "商品id", required = true, dataType = "long")
public Optional<GoodsInfo> getOne(@RequestParam(required = true) long id){
Optional<GoodsInfo> goodsInfo = goodsRepository.findById(id);
return goodsInfo;
}
private SearchQuery getEntitySearchQuery(int pageNumber, String searchContent){
Pageable pageable = PageRequest.of(pageNumber, 20);
SearchQuery searchQuery = new NativeSearchQueryBuilder().withQuery(queryStringQuery(searchContent)).withPageable(pageable).build();
return searchQuery;
}
@ResponseBody
@RequestMapping(value = "/search", method = RequestMethod.GET)
@ApiOperation(value = "搜索商品")
public List<GoodsInfo> search(@RequestParam(required = true) Integer pageNumber, @RequestParam(required = true) String query){
SearchQuery searchQuery = getEntitySearchQuery(pageNumber, query);
Page<GoodsInfo> goodsPage = goodsRepository.search(searchQuery);
return goodsPage.getContent();
}
}