具体实现内容长这个样子:
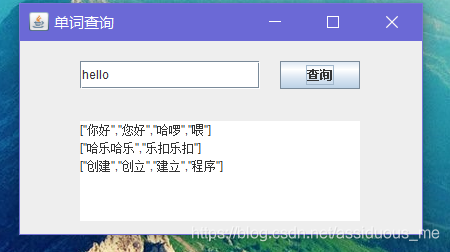
下面开始具体的内容准备:
要调用有道词典的API,必须先申请API
先登录注册再说!
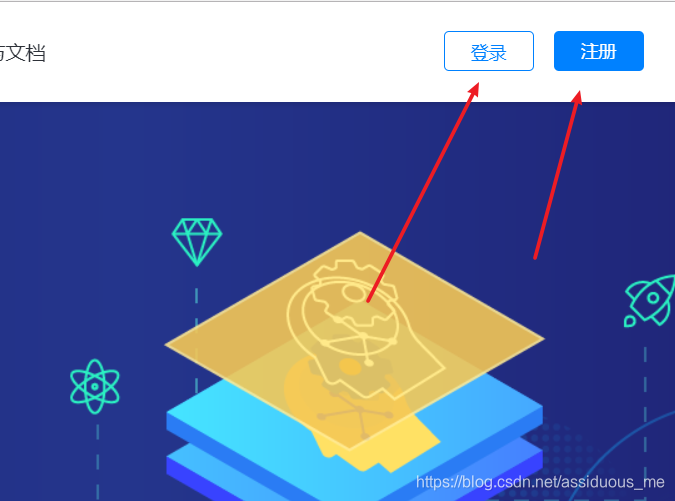
创建应用,创建实例,将实例绑定到应用上
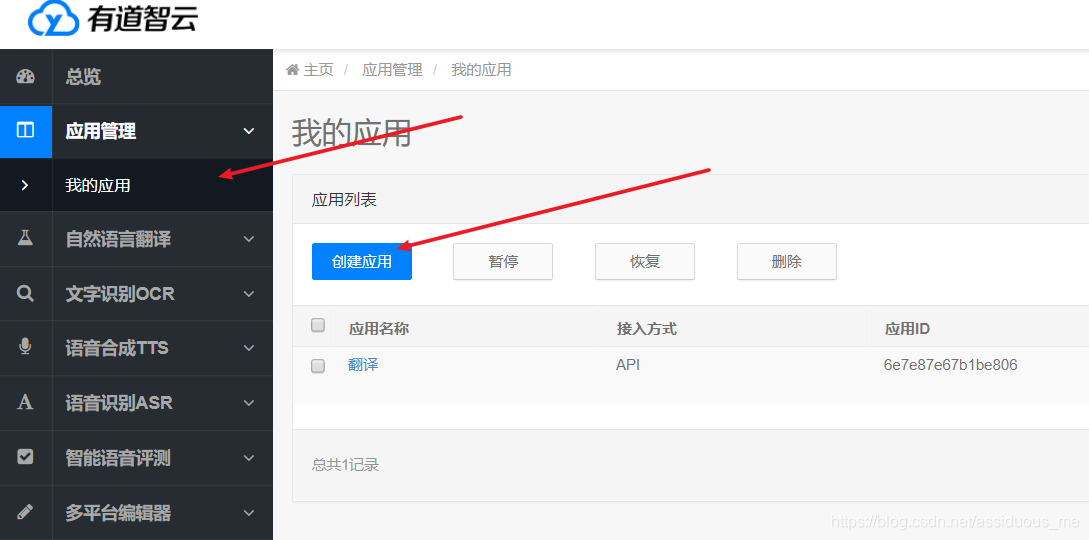
完成后,在我的应用里点进去,获得应用ID,和应用密钥
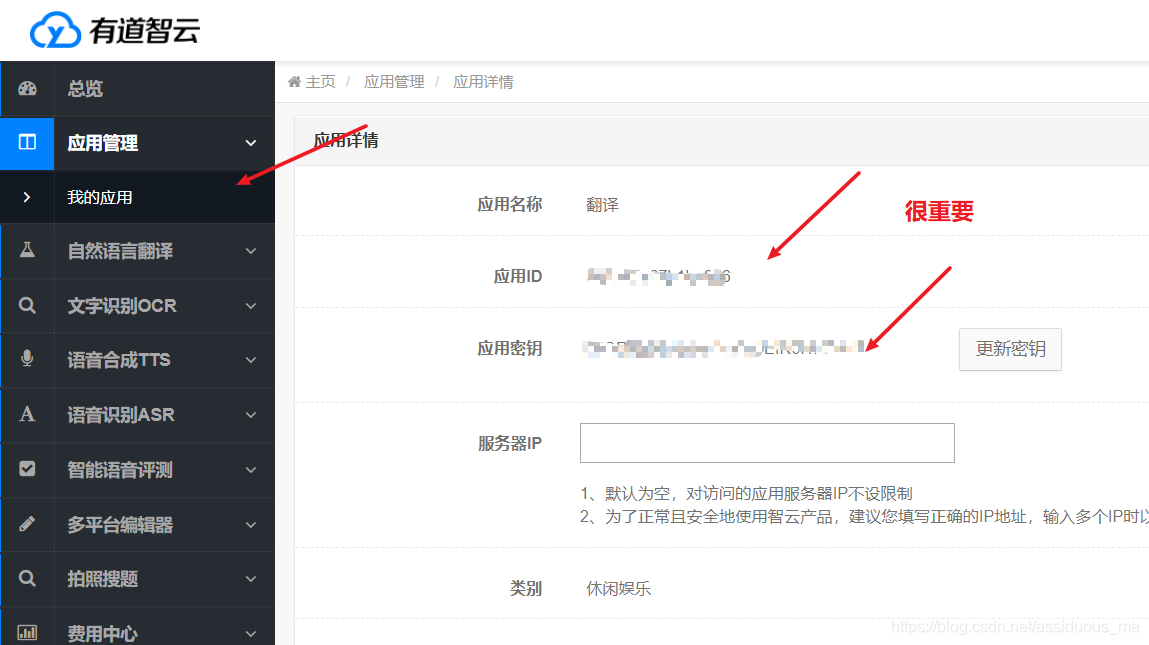
ok了,准备已经做好了,现在写代码(项目具体目录,如下,没有jar包的自己去下载就好了)
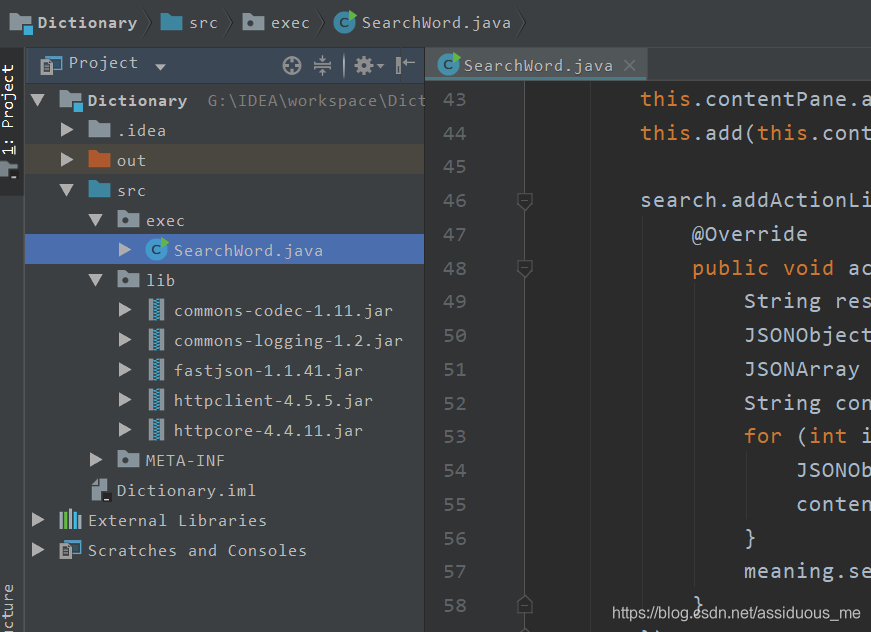
ok,代码如下,将你申请的应用ID和应用密钥替换就可以使用了!
package exec;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.IOException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.*;
public class SearchWord extends JFrame {
private JPanel contentPane;
private JButton search;
private JTextField word;
private JTextArea meaning;
public SearchWord(){
this.setTitle("单词查询");//设置标题
this.setSize(420, 240); //设置窗口大小
this.setLocationRelativeTo(null);//居中显示
this.contentPane = new JPanel();//初始化面板
this.contentPane.setLayout(null);//设置布局NULL
word = new JTextField(20);//设置查询框
search = new JButton("查询");//查询按钮
meaning = new JTextArea(20, 4);//单词意思显示
word.setBounds(60, 20, 180, 28);
search.setBounds(260, 20, 80, 28);
meaning.setBounds(60, 80, 280, 100);
this.contentPane.add(word);
this.contentPane.add(search);
this.contentPane.add(meaning);
this.add(this.contentPane);
search.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String result = HttpRequest.searchWord(word.getText());
JSONObject jsonObject = JSONObject.parseObject(result);
JSONArray jsonArray = JSONArray.parseArray(jsonObject.get("web").toString());
String content = "";
for (int i = 0; i < jsonArray.size(); i++){
JSONObject json = jsonArray.getJSONObject(i);
content += json.get("value").toString() + "\r\n";
}
meaning.setText(content);
}
});
this.setVisible(true);//设置可见
this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);//设置关闭
}
public static void main(String[] args) {
new SearchWord();
}
}
class HttpRequest{
private static final String YOUDAO_URL = "http://openapi.youdao.com/api";
private static final String APP_KEY = "你自己的应用ID";
private static final String APP_SECRET = "你自己的应用密钥";
public static String searchWord(String word){
Map<String,String> params = new HashMap<String,String>();
String q = word;
String salt = String.valueOf(System.currentTimeMillis());
params.put("from", "EN");
params.put("to", "zh-CHS");
params.put("signType", "v3");
String curtime = String.valueOf(System.currentTimeMillis() / 1000);
params.put("curtime", curtime);
String signStr = APP_KEY + truncate(q) + salt + curtime + APP_SECRET;
String sign = getDigest(signStr);
params.put("appKey", APP_KEY);
params.put("q", q);
params.put("salt", salt);
params.put("sign", sign);
/** 处理结果 */
try {
return requestForHttp(YOUDAO_URL,params);
}catch (Exception e){
e.printStackTrace();
}
return null;
}
public static String requestForHttp(String url,Map<String,String> params) throws IOException {
/** 创建HttpClient */
CloseableHttpClient httpClient = HttpClients.createDefault();
/** httpPost */
HttpPost httpPost = new HttpPost(url);
List<NameValuePair> paramsList = new ArrayList<NameValuePair>();
Iterator<Map.Entry<String,String>> it = params.entrySet().iterator();
while(it.hasNext()){
Map.Entry<String,String> en = it.next();
String key = en.getKey();
String value = en.getValue();
paramsList.add(new BasicNameValuePair(key,value));
}
httpPost.setEntity(new UrlEncodedFormEntity(paramsList,"UTF-8"));
CloseableHttpResponse httpResponse = httpClient.execute(httpPost);
try{
HttpEntity httpEntity = httpResponse.getEntity();
String json = EntityUtils.toString(httpEntity,"UTF-8");
EntityUtils.consume(httpEntity);
return json;
}finally {
try{
if(httpResponse!=null){
httpResponse.close();
}
}catch(IOException e){
e.printStackTrace();
}
}
}
/**
* 生成加密字段
*/
public static String getDigest(String string) {
if (string == null) {
return null;
}
char hexDigits[] = {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F'};
byte[] btInput = string.getBytes();
try {
MessageDigest mdInst = MessageDigest.getInstance("SHA-256");
mdInst.update(btInput);
byte[] md = mdInst.digest();
int j = md.length;
char str[] = new char[j * 2];
int k = 0;
for (byte byte0 : md) {
str[k++] = hexDigits[byte0 >>> 4 & 0xf];
str[k++] = hexDigits[byte0 & 0xf];
}
return new String(str);
} catch (NoSuchAlgorithmException e) {
return null;
}
}
public static String truncate(String q) {
if (q == null) {
return null;
}
int len = q.length();
return len <= 20 ? q : (q.substring(0, 10) + len + q.substring(len - 10, len));
}
}
ok,就这样了,大家觉得我写的博客好的话,欢迎在下方留言,或者加关注呦!