题干:
中文:
给定具有头节点头的非空的单链表,返回链表的中间节点。 如果有两个中间节点,则返回第二个中间节点。
Example 1:
Input: [1,2,3,4,5]
Output: Node 3 from this list (Serialization: [3,4,5])
返回的节点的值为3.(该节点的判断序列化为[3,4,5])。 请注意,我们返回了一个ListNode对象ans,这样: ans.val = 3,ans.next.val = 4,ans.next.next.val = 5,ans.next.next.next = NULL。
Example 2:
Input: [1,2,3,4,5,6]
Output: Node 4 from this list (Serialization: [4,5,6])
由于列表有两个值为3和4的中间节点,我们返回第二个节点。
注意:
给定列表中的节点数将介于1和100之间。
英文:
Given a non-empty, singly linked list with head node head
, return a middle node of linked list.
If there are two middle nodes, return the second middle node.
Example 1:
Input: [1,2,3,4,5]
Output: Node 3 from this list (Serialization: [3,4,5])
The returned node has value 3. (The judge's serialization of this node is [3,4,5]).
Note that we returned a ListNode object ans, such that:
ans.val = 3, ans.next.val = 4, ans.next.next.val = 5, and ans.next.next.next = NULL.
Example 2:
扫描二维码关注公众号,回复:
5825021 查看本文章
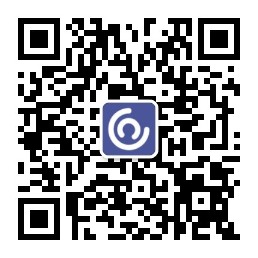
Input: [1,2,3,4,5,6]
Output: Node 4 from this list (Serialization: [4,5,6])
Since the list has two middle nodes with values 3 and 4, we return the second one.
Note:
- The number of nodes in the given list will be between
1
and100
.
解题思路:
先获取链表的长度,获取最中间的结点;
然后将头指针指向最中间的结点,并返回新的链表。
package cn.leetcode.easy;
import cn.kimtian.linkedlist.Node;
/**
* Given a non-empty, singly linked list with head node head, return a middle node of linked list.
* If there are two middle nodes, return the second middle node.
*
* @author kimtian
* @date 2019.02.13
* @num 390
*/
public class MiddleOfTheLinkedList {
/**
* 先获取链表的长度,获取最中间的结点;
* 然后将头指针指向最中间的结点,并返回新的链表
* @param head
* @return
*/
public static Node middleNode(Node head) {
//链表长度为1
int length = 1;
//将头结点的下一个结点作为新结点
Node zz = head.next;
//如果不是最后一个结点,还有下一个结点,则将长度+1,求出链表的长度
while (zz != null) {
length++;
//将下一个结点赋值给当前结点
zz = zz.next;
}
//求出数组的中位结点
int middle = length / 2 + 1;
//移动头指针到中位结点
for (int i = 1; i < middle; i++) {
head = head.next;
}
//并将其返回
return head;
}
/**
* 测试类
*
* @param args
*/
public static void main(String[] args) {
Node n1 = new Node(1);
Node n2 = new Node(2);
Node n3 = new Node(3);
Node n4 = new Node(4);
Node n5 = new Node(5);
Node n6 = new Node(6);
n1.appendNode(n2).appendNode(n3).appendNode(n4).appendNode(n5).appendNode(n6);
Node a = middleNode(n1);
a.showNodes();
}
}