作用域链:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
</head>
<body>
<script>
function a(){
var b = {
name :'',
sex:'',
eat:function(){
},
play:function(){
}
};
return b; // 指向b函数的地址
}
var global = 100;
var demo = a(); //a 函数直接销毁 按道理来说 a not defined
demo();
demo();
</script>
</body>
</html>
js获取元素:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
<style>
/*属性选择器 E[属性名="属性值"] */
.dv {
}
input[type="text"] {
}
input[index='1'] {
}
input[class^="as"]{/* //^ 开头 *包含 $ 结束*/
/*挑选类名以as字符开头的Input标签元素*/
}
</style>
</head>
<body>
<input type="text" index="1" class="asd">
<input type="text" class="as" >
<input type="button" >
<div class="dv"></div>
<div class="dv"></div>
<div class="dv" id="dv1"></div>
<div class="dv a"></div>
<div class="dv b"></div>
<script>
/* var dv = document.getElementById('dv1')
var dvs = document.getElementsByClassName('dv') // 数组
dvs[0].style.width='200px'
dvs[0].style.height='200px'
dvs[0].style.backgroundColor='#342512'*/
var divs = document.getElementsByTagName('div') // 一组 数组
console.log(divs)
//查询选择器方法
var a = document.querySelector('div:nth-of-type(-n+3)') //css选择器方法
var a = document.querySelectorAll('div:nth-of-type(-n+3)') //css选择器方法
// 一个数组 [,,] document.querySelector 他只能返回一个 即使数组 他也只返回数组第一个
var input = document.querySelectorAll('input[class^="as"]')
console.log(input)
</script>
</body>
</html>
对象:
扫描二维码关注公众号,回复:
5813636 查看本文章
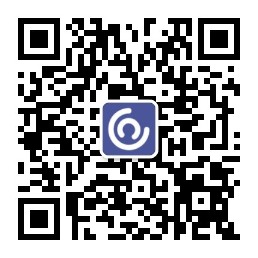
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
</head>
<body>
<script>
/*对象 引用类型 无序键值对的集合 属性+方法
* 定义:糖衣法
* var obj = { } */
function a () {
console.log(this) // this 指的是顶级对象window
}
var obj = {
/*this 指本对象*/
id:1200,
name:'wangdafa',
salary:2300,
hobby:"18years old girls",
eat:function () {
console.log(this.name+"喜欢吃榴莲")
}
}
/*遍历对象 for in*/
for(var index in obj){
/*判断数据的类型 判断基本数据类型 typeof
* 引用 类型 instanceof*/
/*不是对象的属性进行输出*/
/* var obj1 = {}
console.log(obj1 instanceof Object )*/
/*if(!条件){} 条件不成立 则进行{}里面的代码*/
if(!(obj[index] instanceof Object)){
console.log(index,obj[index])
}
}
</script>
</body>
</html>
字符创对象方法:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
</head>
<body>
<script>
/*1:charAt() 根据下标返回第几个字符*/
var str = 'abcdef' //字符串以数组方式存储
/* console.log(str.charAt(3))*/
console.log(str.charCodeAt(3)) // d a 97 A 65
/*连接字符串 concat()*/
/*var str1 = str.concat('hhhhh','kkkkk')*/
console.log(str+'hhhhhkkkk')
/*indexof() 返回值是子串在父串里开始的第一个下标
如果查找失败则返回-1
* string.indexOf(substring) substring 子串
string.indexOf(substring,start) start 是从哪个下标开始找起*/
console.log(str.indexOf('de',4))
var str2 = "http://localhost:63342/5.html?name='lili'&&sex='女'"
/*截取子串 slice(start ,end )*/
var askIndex = str2.indexOf('?')
var a = str2.slice(askIndex+1)
console.log('当前子串'+a)
/*将字符串转换成数组 split('分隔符')*/
//name='lili'&&sex='女'
var b = a.split('&&') // b = [ 'name="lili"','sex="女"']
var obj = {}
for(var i =0;i<b.length;i++){
// shuxm 代表属性名变量
var shuxm = b[i].split('=')[0]; //属性名 [name ,'lili']
obj[shuxm] =b[i].split('=')[1]
}
console.log(obj)
</script>
</body>
</html>
时间对象:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
</head>
<body>
<script>
/*三元表达式 条件?表达式1:表达式2 true 执行 1 */
var time = new Date()
//Wed Mar 27 2019 11:22:54 GMT+0800 (中国标准时间) 1970 1月1日
console.log(time.valueOf())//1553657061549 ms 数
console.log(time.getTime())//1553657061549 ms 数
/* 2019-03-27 11:29:34 */
/* 格式化时间函数 2019-03-27 11:29 */
function formatTime(T) {
var year = T.getFullYear() // 年
var mounth = T.getMonth()+1 //月 0 月开始 11代表12月
var day = T.getDate() //一月中的第几天 1 开始
var hour = T.getHours();
var minutes = T.getMinutes(); // 11:03 ===> 11 : 3
var seconds = T.getSeconds();
console.log(year,mounth,day,hour,minutes,seconds)
var timeStr = year+'-'+(mounth>10?mounth:('0'+mounth)) +'-'+(day>10?day:'0'+day)+' '+
(hour>10?hour:'0'+hour)+':'+(minutes>10?minutes:'0'+minutes)+':'+
( seconds>10?seconds:'0'+seconds);
return timeStr;
}
var T = new Date()
var a = formatTime(T) ;
console.log(a)
</script>
</body>
</html>
js操作文本:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
</head>
<body>
<button class="btn">点击</button>
<div class="dv">
var a = document.querySelector('div:nth-of-type(-n+3)') //css选择器方法
var a = document.querySelectorAll('div:nth-of-type(-n+3)') //css选择器方法
</div>
<script>
var btn = document.querySelector('.btn')
var div = document.querySelector('.dv')
/*
* 1:点击按钮 按钮身上绑定点击事件
* 2:替换div里的文本内容 对象.innerText 获取 对象.innerText = '内容'
* */
/*触发 才执行 回调函数*/
btn.onclick = function () {
/*div.innerText = '哈哈哈嘿嘿嘿 '*/
div.innerHTML= '<a href="http://baidu.com">百度</a> '
}
console.log('safffffffffffff')
</script>
</body>
</html>
js操作属性:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
</head>
<body>
<a href="http://baidu.com" target="">百度</a>
<button id="btn">点击热点文字链接地址</button>
<script src="获取元素.js"></script>
<script>
/* var obj = {
name:'lili',
1:'rty'
}
obj.name 不可以获取属性名是数字的属性
obj.1
obj['1']
obj['name'] 可以获取属性名是数字的属性
obj['index'] ="123"*/
/* 默认属性 对象.属性名 对象['属性名']*/
var btn = my$('#btn');
var mya = my$('a') ;
/*1:点击按钮*/
btn.onclick = function () {
/*修改链接和热点文字*/
mya.innerText = '火狐'
mya.href = 'https://home.firefoxchina.cn/'
}
/*自定义属性*/
</script>
</body>
</html>
点击小图切换大图:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
img:nth-of-type(-n+4) {
width:200px;
height:200px;
float:left;
}
img:nth-of-type(5) {
width:800px;
height:auto;
}
</style>
</head>
<body>
<img src="images/fj1.png" alt="">
<img src="images/fj2.png" alt="">
<img src="images/fj3.png" alt="">
<img src="images/fj4.png" alt="">
<div style="clear:both"></div>
<img src="images/bigimg.png" alt="" id="bigimg">
<script>
window.onload = function () {
var imgs = document.querySelectorAll('img:nth-of-type(-n+4)')//获取前四张图片
// console.log(imgs)
var bigImg = document.querySelector('#bigimg')//获取第五张图片
// console.log(bigImg)
for(var i=0;i<imgs.length;i++){//四张小图片分别是imgs[0],imgs[1],imgs[2],imgs[3]
imgs[i].onmouseover = function () {//点击事件,点击之后执行function里的代码
var srcs = this.src//获取点击的小图片的路径
// console.log(srcs)
bigImg.src=srcs;//修改大图片的路径
}
}
}
</script>
</body>
</html>
表单元素:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
<style>
form {
width: 500px;
}
input,select{
display: block;
}
</style>
</head>
<body>
<!--表单-->
<form action="" method="get" name="form1">
<fieldset>
<legend>学生信息</legend>
昵称: <!--required-->
<input type="text" required name="username">
密码:
<input type="password" required name="password" >
邮箱:
<input type="email" >
<select name="city" id="">
<option value="0" >北京</option>
<option value="1" >北京</option>
<option value="2" selected>成都</option>
<option value="3" >北京</option>
<option value="4" >北京</option>
</select>
爱好:
<input type="checkbox" style="display:inline-block" name="hobby" checked > 运动
<input type="checkbox" style="display:inline-block" name="hobby" > 唱歌
<input type="submit" id="sub">
</fieldset>
</form>
<script>
/*表单元素通过name名字获取
* 获取表单元素的值 对象.value*/
var form = document.form1;//整个表单
/*表单的下拉列表里的选项 form.selectname.options */
console.log(form.password.value)
console.log(form.city.options)
var ops = form.city.options //数组
ops[2].innerText = '成都'
ops[2].value = '45'
console.log(ops[2])
/*提交事件*/
document.getElementById('sub').onclick= function(){
}
var count = 0;
var chk = form.hobby
for(var i =0;i<chk.length ; i++ ){
if(!chk[i].checked){
//不被选中的有几个
console.log(chk[i])
}
}
</script>
</body>
</html>
自定义属性:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
</head>
<body>
<div >div元素1</div>
<div >div元素2</div>
<div >div元素3</div>
<div class="a">div元素4</div>
<script src="获取元素.js"></script>
<script>
/*自定义属性 作用:保存数据 区分*/
/*js操作自定义属性
* 设置:document.setAttribute('属性名',‘属性值’)
* 获取:document.getAttribute('属性名') ===>返回值字符串
* 移除:document.removeAttribute('属性名')*/
var divs = my$All('div');
for(var i = 0;i<divs.length;i++){
divs[i].setAttribute('index',i)
console.log(divs[i].getAttribute('index'))
if(i === 3){
divs[i].removeAttribute('class')
}
}
</script>
</body>
</html>
排他思想:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$美少女战士$</title>
<style>
div {
width: 200px;
height: 200px;
background-color: #a71d5d;
float: left;
margin-left: 50px;
}
</style>
</head>
<body>
<div></div>
<div></div>
<div></div>
<div></div>
<script>
var divs = document.getElementsByTagName('div')
for(var i =0;i<divs.length;i++){
divs[i].onmouseover = function () {
/*先全部变成上面默认背景色*/
for(var j =0;j<divs.length;j++){
divs[j].style.backgroundColor ='#a71d5d'
}
this.style.backgroundColor = 'yellow'
}
}
for(var i =0;i<divs.length;i++){
divs[i].onmouseout = function () {
/*先全部变成上面默认背景色*/
// for(var j =0;j<divs.length;j++){
// divs[j].style.backgroundColor ='#a71d5d'
// }
this.style.backgroundColor = '#a71d5d'
}
}
</script>
</body>
</html>
选项卡切换:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
* {
padding:0;
margin:0;
box-sizing: border-box;
}
.tab {
border:black 1px solid;
width:600px;
height:auto;
margin: 300px auto;
}
.tab-title {
width:600px;
}
.tab-title li {
width:25%;
height:50px;
float:left;
background-color: #9ff3ff;
color:gold;
font-size:25px;
list-style: none;
line-height: 50px;
text-align: center;
}
.tab-body {
width:600px;
height:auto;
position: relative;
}
.tab-body li {
width:100%;
height:500px;
background-color: pink;
color: orange;
font-size: 40px;
list-style: none;
line-height: 500px;
text-align: center;
position: absolute;
top:50px;
left:0;
display: none;
}
</style>
</head>
<body>
<div class="tab">
<ul class="tab-title">
<li style="background-color: greenyellow">国内新闻</li>
<li>国外新闻</li>
<li>娱乐新闻</li>
<li>科技新闻</li>
</ul>
<ul class="tab-body">
<li style="display: block">中国十大最美城市</li>
<li>习大大访问法国</li>
<li>《四季》:好听到爆</li>
<li>震惊:苹果公司竟于昨日倒闭</li>
</ul>
</div>
<script src="获取元素.js"></script>
<script>
window.onload=function(){
var TitleLis = my$All('.tab-title li')//获取类名为title的ul中的li,结果为数组
var BodyLis = my$All('.tab-body li')//获取类名为body的ul中的li,结果为数组
// console.log(TitleLis,BodyLis)
for (var i=0;i<TitleLis.length;i++){
TitleLis[i].setAttribute('index',i)//将小li在数组中的下标标记为index
TitleLis[i].onmouseover = function () {//鼠标移入上面四个小li时执行
var index = this.getAttribute('index')//将小li的下标保存
// console.log(index)
for (var j=0;j<BodyLis.length;j++){
TitleLis[j].style.backgroundColor='#9ff3ff'//将所有小li的背景色变回蓝色
BodyLis[j].style.display="none"//将所有大li隐藏
}
TitleLis[index].style.backgroundColor='greenyellow'//改变下标为index的小li的颜色
BodyLis[index].style.display='block'//显示下标为index的大li
}
}
}
</script>
</body>
</html>
js中遍历对象的方法:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
//遍历对象的方法:
var obj = {
'name':'张三',
'age' :'22',
'sex' :'男'
}
Object.prototype.pro1=function () {};//在原型链上添加属性
Object.defineProperty(obj,'country',{Enumerable:true,value:'ccc'});//可枚举
Object.defineProperty(obj,'nation',{Enumerable:false});//不可枚举
obj.contry = 'china';
//方法1:for...in
//遍历输出的是对象自身的属性以及原型链上可枚举的属性(不含Symbol属性),原型链上的属性最后输
//出说明先遍历的是自身的可枚举属性,后遍历原型链上的
for(var index in obj) {
console.log(index,obj[index])
}
//输出为:name 张三
// age 22
// sex 男
// contry china
// prol f(){}
// //方法2:Object.keys()
// //遍历对象返回的是一个包含对象自身可枚举属性的数组(不含Symbol属性)
// Object.keys(obj).forEach(function(index){
// console.log(index,obj[index])
// })
// //输出为:name 张三
// // age 22
// // sex 男
// // contry china
// //方法3:Objcet.getOwnPropertyNames()
// //输出对象自身的可枚举和不可枚举属性的数组,不输出原型链上的属性
// Object.getOwnPropertyNames(obj).forEach(function(index) {
// console.log(index, obj[index])
// });
// //输出为:name 张三
// // age 22
// // sex 男
// // country ccc
// // nation undefined
// // contry china
</script>
</body>
</html>