文件操作
- fopen与fclose
- fread与fwrite
- fseek
- fputs与fgets
- fscanf与fprintf
fopen与fclose
#include<stdio.h>
int main() {
FILE *fp;
char c;
fp = fopen("1.txt", "r");
if (NULL == fp) {
perror("fopen"); //perror()函数打印str(字符串)和一个相应的执行定义的错误消息到全局变量errno中.
}
while ((c = fgetc(fp)) != EOF) {
putchar(c);
}
fclose(fp);
return 0;
}
fread与fwrite
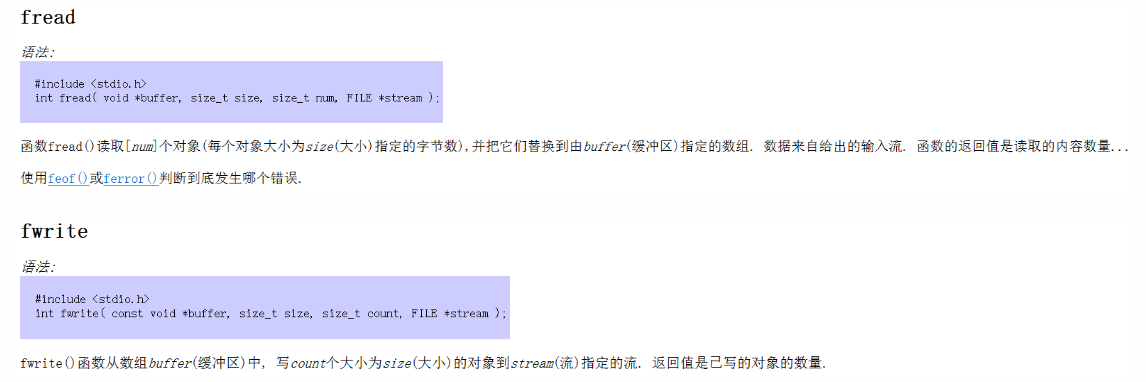
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
FILE *fp;
char buf[128] = { 0 };
int i = 0x12340a78;
int ret;
fp = fopen("1.txt", "rb+");
if (NULL == fp)
{
perror("fopen");
}
//打印文件内容
//while(memset(buf,0,sizeof(buf)),(ret=fread(buf,sizeof(char),sizeof(buf)-1,fp))>0)
//{
// printf("%s",buf);
//}
//写入文件
strcpy(buf, "hello\nworld");
fwrite(buf, sizeof(char), strlen(buf), fp);
//fwrite的返回值是写的对象的数量
/*ret=fwrite(&i,sizeof(int),1,fp);
i=0;*/
/*fread(&i,sizeof(int),1,fp);
printf("i=%x\n",i);*/
fclose(fp);
return 0;
}
fseek
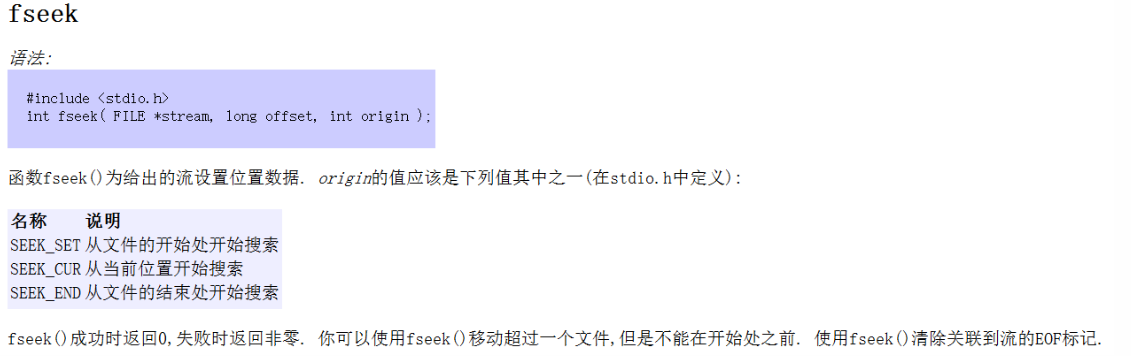
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
FILE *fp;
int ret;
char buf[128]={0};
fp=fopen("1.txt","r+");
if(NULL==fp)
{
perror("fopen");
}
ret=fseek(fp,6,SEEK_SET); //成功返回0,失败返回非零
ret=fread(buf,sizeof(char),2,fp);
printf("buf=%s\n",buf);
fseek(fp,0,SEEK_CUR);
ret=fwrite("you are how",sizeof(char),11,fp);
fclose(fp);
return 0;
}
fputs与fgets
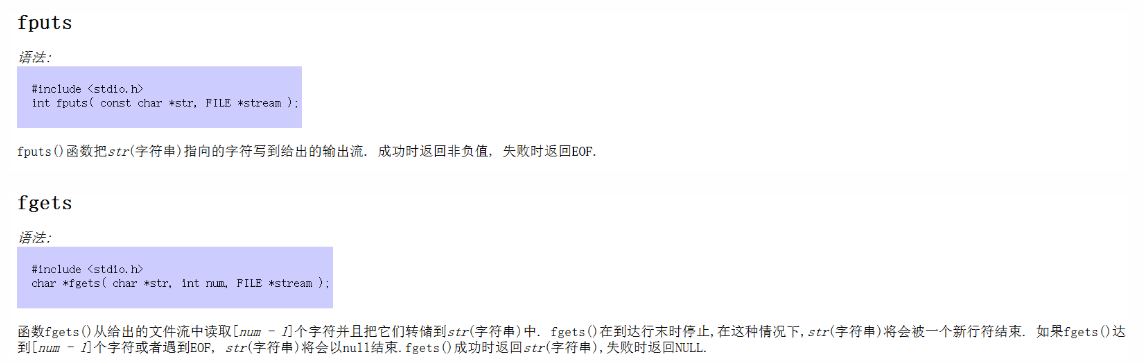
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
FILE *fp;
int ret;
char buf[128];
fp=fopen("1.txt","r+");
if(NULL==fp)
{
perror("fopen");
}
while(fgets(buf,sizeof(buf),stdin))
{
fputs(buf,fp); //等价于printf("%s\n",buf);
}
return 0;
}
fscanf与fprintf
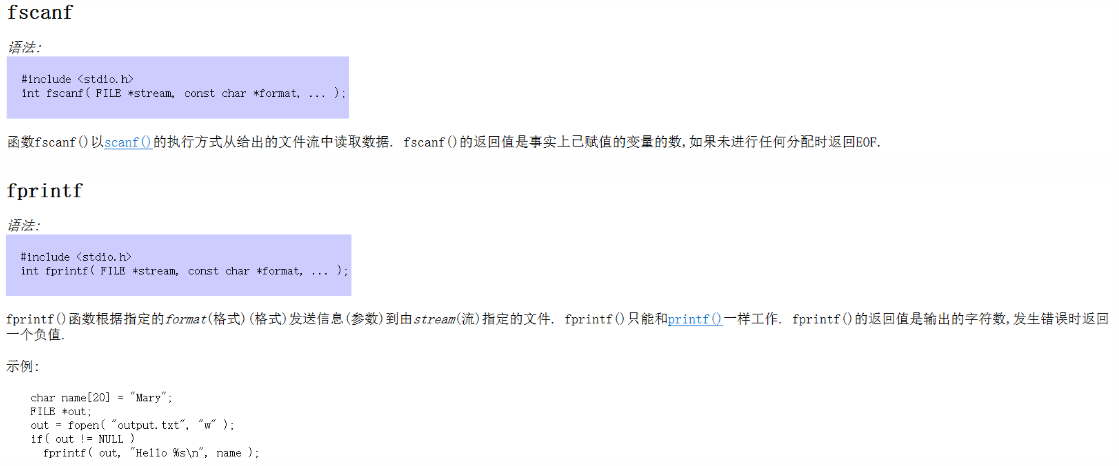
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct{
int num;
char name[20];
float score;
}Stu;
int main()
{
int num=20;
float score=98.5;
Stu sArr[3]={1001,"zhangfei",66.5,1007,"liubei",96.5,1005,"guanyu",97.3};
int ret,i;
FILE *fp=fopen("file.txt","r+");
if(NULL==fp)
{
perror("fopen");
}
//ret=fprintf(fp,"%d %5.2f\n",num,score);
//num=0;
//score=0;
//ret=fscanf(fp,"%d%f",&num,&score);
for(i=0;i<3;i++)
{
ret=fprintf(fp,"%d %s %5.2f\n",sArr[i].num,sArr[i].name,sArr[i].score);
}
fseek(fp,0,SEEK_SET);
memset(sArr,0,sizeof(sArr));
for(i=0;i<3;i++)
{
ret=fscanf(fp,"%d%s%f",&sArr[i].num,sArr[i].name,&sArr[i].score);
}
fclose(fp);
return 0;
}