上一篇文章中介绍了Java调用条码打印机的相关方法,但是在实际生产环境中发现了一些问题,于是在此给出解决办法。
上一个Demo存在的一些问题包括:
1.打印浓度和宽度必须在打印机控制面板设置;
2.主机新添加的打印机获取不到;
3.严重:当某些中文字符无法打印时会终止程序。
4.添加了一个getMessage方法来获取打印结果。
第一个问题,只要在打印指令中加入~SDa和^PWa来控制打印深度和宽度即可。
第二个问题我采用了多种方法均无效,最终在一篇文章中偶然提到的一个在PrintServiceLookup.lookupPrintServices之前执行AppContext.getAppContext().put(PrintServiceLookup.class.getDeclaredClasses()[0], null)就能够解决问题。
第三个问题,通过try...catch捕获异常,并且替换掉这个无法打印的字符就行了。
完整的Demo如下
- import java.io.File;
- import java.io.FileInputStream;
- import java.io.IOException;
- import java.io.UnsupportedEncodingException;
- import javax.print.Doc;
- import javax.print.DocFlavor;
- import javax.print.DocPrintJob;
- import javax.print.PrintException;
- import javax.print.PrintService;
- import javax.print.PrintServiceLookup;
- import javax.print.SimpleDoc;
- import javax.print.attribute.standard.PrinterName;
- import sun.awt.AppContext;
- /**
- * 采用点阵字库ts24.lib
- * @author Labrador
- *
- */
- public class ZplPrinter {
- private String printerURI = null; //打印机完整路径
- private PrintService printService = null; //打印机服务
- private byte[] dotFont;
- private String darkness = "~SD22"; //Set Darkness设置色带颜色的深度 0-30
- private String width = "^PW1200"; //Print Width打印宽度0-1500
- private String length = "^LL800"; //Label Length标签长度0-x(暂无作用)
- private String begin = "^XA" + darkness + width; //标签格式以^XA开始
- private String end = "^XZ"; //标签格式以^XZ结束
- private String content = ""; //打印内容
- private String message = ""; //打印的结果信息
- /**
- * 构造方法
- * @param printerURI 打印机路径
- */
- public ZplPrinter(String printerURI){
- this.printerURI = printerURI;
- //加载字体
- File file = new File("C://ts24.lib");
- if(file.exists()){
- FileInputStream fis;
- try {
- fis = new FileInputStream(file);
- dotFont = new byte[fis.available()];
- fis.read(dotFont);
- fis.close();
- } catch (IOException e) {
- e.printStackTrace();
- }
- }else{
- System.out.println("C://ts24.lib文件不存在");
- }
- //初始化打印机
- AppContext.getAppContext().put(PrintServiceLookup.class.getDeclaredClasses()[0], null);//刷新打印机列表
- PrintService[] services = PrintServiceLookup.lookupPrintServices(null,null);
- if (services != null && services.length > 0) {
- for (PrintService service : services) {
- if (printerURI.equals(service.getName())) {
- printService = service;
- break;
- }
- }
- }
- if (printService == null) {
- System.out.println("没有找到打印机:["+printerURI+"]");
- //循环出所有的打印机
- if (services != null && services.length > 0) {
- System.out.println("可用的打印机列表:");
- for (PrintService service : services) {
- System.out.println("["+service.getName()+"]");
- }
- }
- }else{
- System.out.println("找到打印机:["+printerURI+"]");
- System.out.println("打印机名称:["+printService.getAttribute(PrinterName.class).getValue()+"]");
- }
- }
- /**
- * 设置条形码
- * @param barcode 条码字符
- * @param zpl 条码样式模板
- */
- public void setBarcode(String barcode,String zpl) {
- content += zpl.replace("${data}", barcode);
- }
- /**
- * 中文字符、英文字符(包含数字)混合
- * @param str 中文、英文
- * @param x x坐标
- * @param y y坐标
- * @param eh 英文字体高度height
- * @param ew 英文字体宽度width
- * @param es 英文字体间距spacing
- * @param mx 中文x轴字体图形放大倍率。范围1-10,默认1
- * @param my 中文y轴字体图形放大倍率。范围1-10,默认1
- * @param ms 中文字体间距。24是个比较合适的值。
- */
- public void setText(String str, int x, int y, int eh, int ew, int es, int mx, int my, int ms) {
- byte[] ch = str2bytes(str);
- for (int off = 0; off < ch.length;) {
- if (((int) ch[off] & 0x00ff) >= 0xA0) {//中文字符
- try {
- int qcode = ch[off] & 0xff;
- int wcode = ch[off + 1] & 0xff;
- content += String.format("^FO%d,%d^XG0000%01X%01X,%d,%d^FS\n", x, y, qcode, wcode, mx, my);
- begin += String.format("~DG0000%02X%02X,00072,003,\n", qcode, wcode);
- qcode = (qcode + 128 - 32) & 0x00ff;
- wcode = (wcode + 128 - 32) & 0x00ff;
- int offset = ((int) qcode - 16) * 94 * 72 + ((int) wcode - 1) * 72;
- for (int j = 0; j < 72; j += 3) {
- qcode = (int) dotFont[j + offset] & 0x00ff;
- wcode = (int) dotFont[j + offset + 1] & 0x00ff;
- int qcode1 = (int) dotFont[j + offset + 2] & 0x00ff;
- begin += String.format("%02X%02X%02X\n", qcode, wcode, qcode1);
- }
- x = x + ms * mx;
- off = off + 2;
- } catch (Exception e) {
- e.printStackTrace();
- //替换成X号
- setChar("X", x, y, eh, ew);
- x = x + es;//注意间距更改为英文字符间距
- off = off + 2;
- }
- } else if (((int) ch[off] & 0x00FF) < 0xA0) {//英文字符
- setChar(String.format("%c", ch[off]), x, y, eh, ew);
- x = x + es;
- off++;
- }
- }
- }
- /**
- * 英文字符串(包含数字)
- * @param str 英文字符串
- * @param x x坐标
- * @param y y坐标
- * @param h 高度
- * @param w 宽度
- */
- public void setChar(String str, int x, int y, int h, int w) {
- content += "^FO" + x + "," + y + "^A0," + h + "," + w + "^FD" + str + "^FS";
- }
- /**
- * 英文字符(包含数字)顺时针旋转90度
- * @param str 英文字符串
- * @param x x坐标
- * @param y y坐标
- * @param h 高度
- * @param w 宽度
- */
- public void setCharR(String str, int x, int y, int h, int w) {
- content += "^FO" + x + "," + y + "^A0R," + h + "," + w + "^FD" + str + "^FS";
- }
- /**
- * 获取完整的ZPL
- * @return
- */
- public String getZpl() {
- return begin + content + end;
- }
- /**
- * 重置ZPL指令,当需要打印多张纸的时候需要调用。
- */
- public void resetZpl() {
- begin = "^XA" + darkness + width;
- end = "^XZ";
- content = "";
- }
- /**
- * 打印
- * @param zpl 完整的ZPL
- */
- public boolean print(String zpl){
- if(printService==null){
- message = "打印出错:没有找到打印机["+printerURI+"]";
- System.out.println("打印出错:没有找到打印机["+printerURI+"]");
- return false;
- }
- DocPrintJob job = printService.createPrintJob();
- byte[] by = zpl.getBytes();
- DocFlavor flavor = DocFlavor.BYTE_ARRAY.AUTOSENSE;
- Doc doc = new SimpleDoc(by, flavor, null);
- try {
- job.print(doc, null);
- message = "已打印";
- System.out.println("已打印");
- return true;
- } catch (PrintException e) {
- message = "打印出错:"+e.getMessage();
- System.out.println("打印出错:"+e.getMessage());
- e.printStackTrace();
- return false;
- }
- }
- public String getMessage(){
- return message;
- }
- /**
- * 字符串转byte[]
- * @param s
- * @return
- */
- private byte[] str2bytes(String s) {
- if (null == s || "".equals(s)) {
- return null;
- }
- byte[] abytes = null;
- try {
- abytes = s.getBytes("gb2312");
- } catch (UnsupportedEncodingException ex) {
- ex.printStackTrace();
- }
- return abytes;
- }
- }
测试main方法如下
扫描二维码关注公众号,回复:
576684 查看本文章
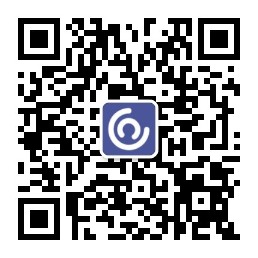
- public class ZplPrinterTest {
- public static void main(String[] args) {
- ZplPrinter p = new ZplPrinter("\\\\10.10.13.235\\ZDesigner 105SLPlus-300dpi ZPL");
- // ZplPrinter p = new ZplPrinter("\\\\10.10.13.224\\ZDesigner GT800 (ZPL)");
- // printBarcode(p);
- // p.resetZpl();//清除
- // printPicking300DPI(p);
- // p.resetZpl();//清除
- // printPicking203DPI(p);
- // p.resetZpl();//清除
- printRestoking(p);
- }
- private static boolean printBarcode(ZplPrinter p){
- //1.打印单个条码
- String bar0 = "131ZA010101";//条码内容
- // String bar0Zpl = "^FO110,110^BY6,3.0,280^BCN,,Y,N,N^FD${data}^FS";//条码样式模板
- String bar0Zpl = "^FO100,50^AAN,100,110^BY6,3.0,280^BCN,,N,N,N^FD${data}^FS";//条码样式模板
- p.setBarcode(bar0, bar0Zpl);
- p.setChar(bar0,200,380,140,110);
- p.setText("上", 880, 380, 60, 60, 30, 4, 4, 24);
- String zpl = p.getZpl();
- System.out.println(zpl);
- boolean result = p.print(zpl);//打印
- return result;
- }
- private static boolean printPicking300DPI(ZplPrinter p){
- //左边的条码
- String bar1 = "07";
- p.setChar(bar1, 190, 130, 60, 60);
- String bar1Zpl = "^FO100,200^BY8,3.0,240^BCR,,N,N,N^FD${data}^FS";//条码样式模板
- p.setBarcode(bar1,bar1Zpl);
- //下边的条码
- String bar2 = "123459999900188ABCDE";//20位
- String bar2Paper = "^FO380,600^BY3,3.0,100^BCN,,Y,N,N^FD${data}^FS";//条码样式模板
- p.setBarcode(bar2,bar2Paper);
- p.setText("国药控股湖南有限公司", 380, 40, 60, 60, 30, 2, 2, 24);
- p.setChar("CSS0BPKPPR", 380, 100, 60, 60);
- p.setText("09件",940, 80, 60, 60, 30, 2, 2, 24);
- p.setText("补", 1100, 80, 60, 60, 30, 2, 2, 24);
- p.setText("公司自配送 公路", 380, 180, 80, 80, 30, 3, 3, 24);
- p.setChar("03231151",940, 187, 40, 40);
- p.setChar("2015-10-10",940, 227, 30, 30);
- p.setText("湖南六谷大药房连锁有限公司", 380, 260, 60, 60, 30, 2, 2, 24);
- p.setText(":长沙市:开福区:捞刀河镇中岭村258号:", 380, 320, 60, 60, 30, 2, 2, 22);
- p.setText("多SKU", 800, 485, 60, 60, 30, 2, 2, 24);
- p.setText("库位:49", 380, 420, 56, 56, 30, 2, 2, 24);
- p.setText("品类:感冒胶囊", 380, 485, 56, 56, 30, 2, 2, 24);
- p.setText("批号:", 380, 550, 56, 56, 30, 2, 2, 24);
- p.setChar("78787878788", 500, 560, 40, 40);
- String zpl = p.getZpl();
- System.out.println(zpl);
- boolean result = p.print(zpl);
- return result;
- }
- private static boolean printPicking203DPI(ZplPrinter p){
- //左边的条码
- String bar1 = "07";
- p.setChar(bar1, 126, 86, 40, 40);
- String bar1Zpl = "^FO66,133^BY5,3.0,160^BCR,,N,N,N^FD${data}^FS";//条码样式模板
- p.setBarcode(bar1,bar1Zpl);
- //下边的条码
- String bar2 = "00000999990018822969";//20位
- String bar2Paper = "^FO253,400^BY2,3.0,66^BCN,,Y,N,N^FD${data}^FS";//条码样式模板
- p.setBarcode(bar2,bar2Paper);
- p.setText("国药控股湖南有限公司", 253, 26, 40, 40, 20, 1, 1, 24);
- p.setChar("CSS0BPKPPR", 253, 66, 20, 20);
- p.setText("09件",626, 53, 40, 40, 20, 1, 1, 24);
- p.setText("补", 733, 53, 40, 40, 20, 1, 1, 24);
- p.setText("公司自配送 公路", 253, 120, 53, 53, 20, 1, 1, 24);
- p.setChar("03231151",626, 124, 26, 26);
- p.setChar("2015-10-10",626, 151, 20, 20);
- p.setText("湖南六谷大药房连锁有限公司", 253, 173, 40, 40, 20, 1, 1, 24);
- p.setText("长沙市开福区捞刀河镇中岭村258号", 253, 213, 30, 30, 20, 1, 1, 22);
- p.setText("多SKU", 533, 323, 40, 40, 20, 1, 1, 24);
- p.setText("库位:49", 253, 280, 37, 37, 20, 1, 1, 24);
- p.setText("品类:感冒胶囊", 253, 323, 37, 37, 20, 1, 1, 24);
- p.setText("批号:", 253, 366, 37, 37, 20, 1, 1, 24);
- p.setChar("78787878788", 333, 373, 26, 26);
- String zpl = p.getZpl();
- System.out.println(zpl);
- boolean result = p.print(zpl);
- return result;
- }
- private static boolean printRestoking(ZplPrinter p){
- //上方的条码
- String bar1 = "1434455567773344abcd";
- String bar1Zpl = "^FO85,70^BY4,3.0,140^BCN,,Y,N,N^FD${data}^FS";//条码样式模板
- p.setBarcode(bar1,bar1Zpl);
- //源货位
- p.setText("来源:131ZA010101", 100, 320, 60, 60, 30, 2, 2, 24);
- //目标货位
- p.setText("目的:131ZA010102",640, 320, 60, 60, 30, 2, 2, 24);
- //货品编号
- p.setText("货品编号:YF10001", 100, 440, 60, 60, 30, 2, 2, 24);
- //件装数
- p.setText("补货数量:200", 640, 440, 60, 60, 30, 2, 2, 24);
- //品名
- p.setText("复方一支黄花:", 100, 580, 60, 60, 30, 2, 2, 24);
- String zpl = p.getZpl();
- System.out.println(zpl);
- boolean result = p.print(zpl);
- return result;
- }
- }