我们手机安装很多应用时,分屏摆放,不同屏的应用切换时的效果我们使用得多,如何实现呢?转载自:
(1)主界面代码SwitchViewDemoActivity.java:
package com.example.switchview; import android.app.Activity; import android.os.Bundle; import android.view.Gravity; import android.view.View; import android.view.View.OnClickListener; import android.widget.ImageView; import android.widget.LinearLayout; import android.widget.LinearLayout.LayoutParams; public class SwitchViewDemoActivity extends Activity implements OnViewChangeListener, OnClickListener { private MyScrollLayout mScrollLayout; private ImageView[] mImageViews; private int mViewCount; private int mCurSel; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); init(); } private void init() { mScrollLayout = (MyScrollLayout) findViewById(R.id.ScrollLayout); LinearLayout linearLayout = (LinearLayout) findViewById(R.id.llayout); //如果不想写死在布局文件里也可以动态添加,这里动态添加一个作为示例 // 动态添加一个layout控件 LinearLayout layout = new LinearLayout(this); layout.setLayoutParams(new LayoutParams(LayoutParams.FILL_PARENT,LayoutParams.FILL_PARENT)); layout.setBackgroundResource(R.drawable.mm_1); mScrollLayout.addView(layout); // 动态添加一个imageView控件 ImageView imageView = new ImageView(this); LayoutParams lp = new LayoutParams(LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT); lp.gravity = Gravity.CENTER; imageView.setLayoutParams(lp); imageView.setPadding(15, 15, 15, 15); imageView.setImageResource(R.drawable.white_dot); linearLayout.addView(imageView); mViewCount = mScrollLayout.getChildCount(); mImageViews = new ImageView[mViewCount]; for (int i = 0; i < mViewCount; i++) { mImageViews[i] = (ImageView) linearLayout.getChildAt(i); mImageViews[i].setEnabled(true); mImageViews[i].setOnClickListener(this); mImageViews[i].setTag(i); } mCurSel = 0; mImageViews[mCurSel].setEnabled(false); mScrollLayout.SetOnViewChangeListener(this); } private void setCurPoint(int index) { if (index < 0 || index > mViewCount - 1 || mCurSel == index) { return; } mImageViews[mCurSel].setImageResource(R.drawable.white_dot); mImageViews[index].setImageResource(R.drawable.green_dot); mImageViews[mCurSel].setEnabled(true); mImageViews[index].setEnabled(false); mCurSel = index; } @Override public void OnViewChange(int view) { setCurPoint(view); } @Override public void onClick(View v) { int pos = (Integer) (v.getTag()); setCurPoint(pos); mScrollLayout.snapToScreen(pos); } }
(2) 主滑动自定义控件MyScrollLayout.java:
package com.example.switchview; import android.content.Context; import android.util.AttributeSet; import android.util.Log; import android.view.MotionEvent; import android.view.VelocityTracker; import android.view.View; import android.view.ViewGroup; import android.widget.Scroller; /** * 一组图片滑动主控件 * * @author chenwenbiao * @date 2014-6-7 下午3:55:12 * @version V1.0 */ public class MyScrollLayout extends ViewGroup { private VelocityTracker mVelocityTracker; // 用于判断甩动手势 private static final int SNAP_VELOCITY = 600; private Scroller mScroller; // 滑动控制器 private int mCurScreen; private int mDefaultScreen = 0; private float mLastMotionX; // private int mTouchSlop; private OnViewChangeListener mOnViewChangeListener; public MyScrollLayout(Context context) { super(context); // TODO Auto-generated constructor stub init(context); } public MyScrollLayout(Context context, AttributeSet attrs) { super(context, attrs); // TODO Auto-generated constructor stub init(context); } public MyScrollLayout(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); // TODO Auto-generated constructor stub init(context); } private void init(Context context) { mCurScreen = mDefaultScreen; // mTouchSlop = // ViewConfiguration.get(getContext()).getScaledTouchSlop(); mScroller = new Scroller(context); } @Override protected void onLayout(boolean changed, int l, int t, int r, int b) { // TODO Auto-generated method stub if (changed) { int childLeft = 0; final int childCount = getChildCount(); for (int i = 0; i < childCount; i++) { final View childView = getChildAt(i); if (childView.getVisibility() != View.GONE) { final int childWidth = childView.getMeasuredWidth(); childView.layout(childLeft, 0, childLeft + childWidth, childView.getMeasuredHeight()); childLeft += childWidth; } } } } @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { // TODO Auto-generated method stub super.onMeasure(widthMeasureSpec, heightMeasureSpec); final int width = MeasureSpec.getSize(widthMeasureSpec); final int widthMode = MeasureSpec.getMode(widthMeasureSpec); final int count = getChildCount(); for (int i = 0; i < count; i++) { getChildAt(i).measure(widthMeasureSpec, heightMeasureSpec); } scrollTo(mCurScreen * width, 0); } public void snapToDestination() { final int screenWidth = getWidth(); final int destScreen = (getScrollX() + screenWidth / 2) / screenWidth; snapToScreen(destScreen); } public void snapToScreen(int whichScreen) { // get the valid layout page whichScreen = Math.max(0, Math.min(whichScreen, getChildCount() - 1)); if (getScrollX() != (whichScreen * getWidth())) { final int delta = whichScreen * getWidth() - getScrollX(); mScroller.startScroll(getScrollX(), 0, delta, 0, Math.abs(delta) * 2); mCurScreen = whichScreen; invalidate(); // Redraw the layout if (mOnViewChangeListener != null) { mOnViewChangeListener.OnViewChange(mCurScreen); } } } @Override public void computeScroll() { // TODO Auto-generated method stub if (mScroller.computeScrollOffset()) { scrollTo(mScroller.getCurrX(), mScroller.getCurrY()); postInvalidate(); } } @Override public boolean onTouchEvent(MotionEvent event) { // TODO Auto-generated method stub final int action = event.getAction(); final float x = event.getX(); final float y = event.getY(); switch (action) { case MotionEvent.ACTION_DOWN: Log.i("", "onTouchEvent ACTION_DOWN"); if (mVelocityTracker == null) { mVelocityTracker = VelocityTracker.obtain(); mVelocityTracker.addMovement(event); } if (!mScroller.isFinished()) { mScroller.abortAnimation(); } mLastMotionX = x; break; case MotionEvent.ACTION_MOVE: int deltaX = (int) (mLastMotionX - x); if (IsCanMove(deltaX)) { if (mVelocityTracker != null) { mVelocityTracker.addMovement(event); } mLastMotionX = x; scrollBy(deltaX, 0); } break; case MotionEvent.ACTION_UP: int velocityX = 0; if (mVelocityTracker != null) { mVelocityTracker.addMovement(event); mVelocityTracker.computeCurrentVelocity(1000); velocityX = (int) mVelocityTracker.getXVelocity(); } if (velocityX > SNAP_VELOCITY && mCurScreen > 0) { // Fling enough to move left snapToScreen(mCurScreen - 1); } else if (velocityX < -SNAP_VELOCITY && mCurScreen < getChildCount() - 1) { // Fling enough to move right snapToScreen(mCurScreen + 1); } else { snapToDestination(); } if (mVelocityTracker != null) { mVelocityTracker.recycle(); mVelocityTracker = null; } // mTouchState = TOUCH_STATE_REST; break; } return true; } private boolean IsCanMove(int deltaX) { if (getScrollX() <= 0 && deltaX < 0) { return false; } if (getScrollX() >= (getChildCount() - 1) * getWidth() && deltaX > 0) { return false; } return true; } public void SetOnViewChangeListener(OnViewChangeListener listener) { mOnViewChangeListener = listener; } }
(3)接口OnViewChangeListener:
package com.example.switchview; public interface OnViewChangeListener { public void OnViewChange(int view); }
(4)布局文件activity_main.xml:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" > <com.example.switchview.MyScrollLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/ScrollLayout" android:layout_width="fill_parent" android:layout_height="fill_parent" > <LinearLayout android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/mm_1" > </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/mm_2" > </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/mm_3" > </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/mm_4" > </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/mm_5" > </LinearLayout> </com.example.switchview.MyScrollLayout> <LinearLayout android:id="@+id/llayout" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true" android:layout_marginBottom="24.0dip" android:orientation="horizontal" > <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/green_dot" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/white_dot" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/white_dot" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/white_dot" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/white_dot" /> </LinearLayout> </RelativeLayout>
扫描二维码关注公众号,回复:
571626 查看本文章
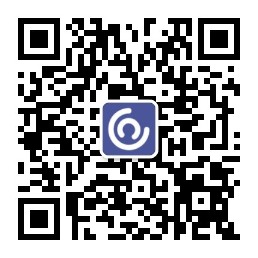
效果图:
切换的都是图片,这里可能会使用到就是点,也上传了.