EnumValue是一种特殊类型,它与所有枚举实例相统一。HAXE标准库使用它为所有枚举实例提供特定的操作,并且可以在API需要枚举实例而不是特定实例的情况下相应地用于用户代码中。
enum Color {
Red;
Green;
Blue;
Rgb(r:Int, g:Int, b:Int);
}
class Main {
static public function main() {
//var ec:EnumValue = Red; // valid
var ec = Red;
trace(ec);
var en:Enum<Color> = Color; // valid
trace(en);
// Error: Color should be Enum<Color>
//var x:Enum<Color> = Red;
}
}
枚举由enum关键字表示,并包含一个或多个枚举构造函数。枚举构造函数可以包含任意数量的构造函数参数。
// Describes a type of item that can be rewarded
enum ItemType {
Key;
Sword(name:String, attack:Int);
Shield(name:String, defense:Int);
}
// Describes a type of reward that can be given
enum RewardType {
Gold(value:Int);
Experience(value:Int);
Item(type:ItemType);
}
有两种方式创建enum
1、构造法
var gold = RewardType.Gold(123);
var experience = RewardType.Experience(456);
var item = RewardType.Item(ItemType.Key);
2、enum tool法
这个前提是using haxe.EnumTools
// Creates Sword item type with name Slashy and strength 100
var createdByName = ItemType.createByName("Sword", ["Slashy", 100]);
// Creates Key item type, as it is the first constructor specified
var createdByIndex = ItemType.createByIndex(0);
-----------------------------------
enum使用案例
package;
using haxe.EnumTools;
/**
* ...
* @author hw
*/
enum ItemType
{
Key;
Sword(name:String, attack:Int);
Shield(name:String, defense:Int);
}
// Describes a type of reward that can be given
enum RewardType
{
Gold(value:Int);
Experience(value:Int);
Item(type:ItemType);
}
class Main
{
static function main()
{
var reward= RewardType.Item(ItemType.Sword("Slashy", 100));
switch (reward)
{
case RewardType.Gold(value):
trace('I got $value gold!');
case RewardType.Experience(value):
trace('I got $value experience!');
case RewardType.Item(type):
switch (type)
{
case ItemType.Key:
trace('I got a key!');
case ItemType.Sword(name, attack):
trace('I got "$name", a sword with $attack attack!');
case ItemType.Shield(name, defense):
trace('I got "$name", a shield with $defense defense!');
}
}
// Gets enum name, including path
var enumName = ItemType.getName();
trace(enumName);//输出的'ItemType'
// Gets array of constructor names for provided enum
var enumConstructorNames = RewardType.getConstructors();// 官方教程可能比较老,用的是
//getNames(),3.4.7版本后应该没有这个方法了
trace(enumConstructorNames);//输出的是RewardType中的三个成员,是数组
}
}
案例改自官方教程。我看了一下源码,官方的教程的版本不对(可能是教程版本比较老,我现在所使用的是3.4.7),首先已经没有getNames的方法,取代的是getConstructors()
其次,也不能 using haxe.EnumValueTools; 因为这个现在是内部类无法被外部引用。
--------------------------------------
EnumValueTools(改成内部类)
扫描二维码关注公众号,回复:
5518422 查看本文章
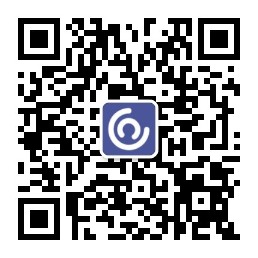
using haxe.EnumTools;
/**
* ...
* @author hw
*/enum ItemType
{
Key;
Sword(name:String, attack:Int);
Shield(name:String, defense:Int);
}
// Describes a type of reward that can be given
enum RewardType
{
Gold(value:Int);
Experience(value:Int);
Item(type:ItemType);
}
class Main
{
static function main()
{
var item = ItemType.Shield("Shieldy", 100);
var constructorName = item.getName();
trace(constructorName);
// Gets enum instance constructor index
var constructorIndex = item.getIndex();
trace(constructorIndex);
// Gets enum instance constructor arguments
var constructorArguments = item.getParameters();
trace(constructorArguments);
var otherItem = ItemType.Sword("Slashy", 100);
// Compares two enum instances recursively
if (item.equals(otherItem)) trace("Items are equal!");
// Matches enum instance against pattern
if (otherItem.match(ItemType.Shield(_, _))) trace("Other item is a shield!");
}
}