路由:
路由定义了如何响应客户端请求以及响应结束点。
路由是URI的组成部分,包含http请求方法,和一个或多个处理方法。路由的结构为“app.METHOD(path,[callback...],callback)"。”app“是一个express实例,”METHOD“为http请求方法,”path“是服务路径,”callback“路径匹配时执行的方法。
下面是一个非常基础的路由示例:
var express = require('express') var app = express() // respond with "hello world" when a GET request is made to the homepage app.get('/', function(req, res) { res.send('hello world') })
路由方法:
路由方法衍生于HTTP方法,是express实例的属性。
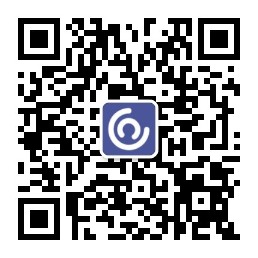
下面是关于路由的一个例子,定义了应用根路径的GET和POST方法:
// GET method route app.get('/', function (req, res) { res.send('GET request to the homepage') }) // POST method route app.post('/', function (req, res) { res.send('POST request to the homepage') })
Express提供了一下路由方法,相当于HTTP方法的:get,post,put,head,delete,options,trace,copy,lock,mkcol,move,purge,propfind,proppatch,unlock,report,mkactivity,checkout,merge,m-search,notify,subscribe,unsubscribe,patch,search,connect。
使用方括号([ ])记法,可以通过javascript变量名调用路由方法,例如: app.['m-search']('/',function...)
这里有个一个特殊的路由方法”app.all()",不衍生于任何HTTP方法。它被用于为所有请求方法的路径加载中间件。
例如,下面的例子中,使用GET,POST,PUT,DELETE或“http module”模块提供的其他HTTP请求方法访问“/secret”路径时,将运行处理方法。
app.all('/secret', function (req, res, next) { console.log('Accessing the secret section ...') next() // pass control to the next handler })
路由路径:
路由路径,结合路由方法,定义了请求的端点以及哪一类请求可以访问。路径可以是字符串,字符串模式或者正则表达式。
备注:Express使用“path-to-regexp”来匹配路径,关于路径的详细信息可以参考它的文档。“Express Route Tester”是测试Express路由的处理工具。
注意:查询字符串不是路由路径的一部分。
下面是字符串路由路径的例子:
// 匹配根“/”路径 app.get('/', function (req, res) { res.send('root') }) // 匹配“/about”路径 app.get('/about', function (req, res) { res.send('about') }) // 匹配"/random.text"路径 app.get('/random.text', function (req, res) { res.send('random.text') })
下面是字符串模式路由路径的例子:
// will match acd and abcd app.get('/ab?cd', function(req, res) { res.send('ab?cd') }) // will match abcd, abbcd, abbbcd, and so on app.get('/ab+cd', function(req, res) { res.send('ab+cd') }) // will match abcd, abxcd, abRABDOMcd, ab123cd, and so on app.get('/ab*cd', function(req, res) { res.send('ab*cd') }) // will match /abe and /abcde app.get('/ab(cd)?e', function(req, res) { res.send('ab(cd)?e') })
备注:字符?,+,*和()是Express中正则表达式的子集.连字符(-)和点号(.)基于字符串路径逐字解释.
下面是正则表达式路由路径的例子:
// will match anything with an a in the route name: app.get(/a/, function(req, res) { res.send('/a/') }) // will match butterfly, dragonfly; but not butterflyman, dragonfly man, and so on app.get(/.*fly$/, function(req, res) { res.send('/.*fly$/') })
路由处理方法:
你可以针对一个请求提供多个回调函数,它们的行为就像中间件.这里有一个特殊情况: 当回调函数调用"next('route')"时,就会忽略剩余的回调函数.你可以利用这个机制给路径添加先决条件;满足条件则执行后续的路径,不满足条件则继续执行当前路径.
路由处理可以是单个的函数,也可以是函数数组,或者两者结合使用.下面是相关的例子:
使用单个函数处理:
app.get('/example/a', function (req, res) { res.send('Hello from A!') })
使用多个函数处理(确保指定了'next'对象):
app.get('/example/b', function (req, res, next) { console.log('response will be sent by the next function ...') next() }, function (req, res) { res.send('Hello from B!') })
使用函数数组处理:
var cb0 = function (req, res, next) { console.log('CB0') next() } var cb1 = function (req, res, next) { console.log('CB1') next() } var cb2 = function (req, res) { res.send('Hello from C!') } app.get('/example/c', [cb0, cb1, cb2])
使用函数数组和独立的函数组合处理:
var cb0 = function (req, res, next) { console.log('CB0') next() } var cb1 = function (req, res, next) { console.log('CB1') next() } app.get('/example/d', [cb0, cb1], function (req, res, next) { console.log('response will be sent by the next function ...') next() }, function (req, res) { res.send('Hello from D!') })
响应方法:
下表中响应对象(res)的方法,用于响应客户端的请求并结束请求响应周期.如果在路由处理函数中没有调用任何响应方法,那么客户端的请求将被挂起.
res.download() | 提示文件被下载 |
res.end() | 结束响应过程 |
res.json() | 发送JSON响应 |
res.jsonp() | 发送支持JSONP的JSON响应 |
res.redirect() | 重定向请求 |
res.render() | 渲染视图模板 |
res.send() | 发送各种类型的响应 |
res.sendFile() | 将指定文件作为字节流响应 |
res.sendStatus() | 设置响应状态码,并发送其字符串表示为响应体。 |
app.route()
可以用app.route()创建链式路由处理。由于路径在一个位置指定,它有助于创建模块化路线,减少冗余和错别字。有关路由的详细信息,请参阅路由文档。
下面是使用app.route()定义的链式路由处理的例子:
app.route('/book') .get(function(req, res) { res.send('Get a random book'); }) .post(function(req, res) { res.send('Add a book'); }) .put(function(req, res) { res.send('Update the book'); })
express.Router
express.Router类可用于创建模块化可安装的路由处理程序。一个Router实例是一个完整的中间件和路由系统;因为这个原因,它通常被称为“小应用程序”。
下面的示例演示创建一个路由模块,并加载中间件,定义路径,并将它安装在主应用的一个路径上。
在应用文件夹中创建一个birds.js的文件,并添加如下内容:
var express = require('express'); var router = express.Router(); // middleware specific to this router router.use(function timeLog(req, res, next) { console.log('Time: ', Date.now()); next(); }) // define the home page route router.get('/', function(req, res) { res.send('Birds home page'); }) // define the about route router.get('/about', function(req, res) { res.send('About birds'); }) module.exports = router;
然后在应用中加载这个路由模块:
var birds = require('./birds'); ... app.use('/birds', birds);
现在该应用将能够处理"/birds"和"/birds/about"路径上的请求,以及调用特别为路由配置的中间件timeLog。
以上内容翻译自Express官网文档,原文参考Express Routing