一:学习之路
从今天开始数据结构的学习,学习的课程是刘宇波老师的《玩转数据结构》,并通过CSDN记录自己的学习之路,加油哦!!!
二:实现内容:
1.Array类里面实现的众多数组的基本操作,包括增、删、查、改等方法;
2.main函数里面完成了对Array方法的测试,并通过println显示测试结果;
3.补充了动态数组以及泛型的方法,使得功能更加全面。
三:代码及注释
1.Array类:
package com.company;
public class Array<E> {
private E[] data;
private int size;
// 构造函数,传入数组的容量capacity构造Array
public Array(int capacity){
data = (E[]) new Object [capacity];
size = 0;
}
// 无参数的构造函数,默认数组的容量capacity=10
public Array(){
this(10);
}
// 获取数组的容量
public int getCapacity(){
return data.length;
}
// 获取数组中的元素个数
public int getSize(){
return size;
}
// 返回数组是否为空
public boolean isEmpty(){
return size == 0;
}
// 向所有元素后添加一个新元素
public void addLast(E e){
add(size, e);
}
// 在所有元素前添加一个新元素
public void addFirst(E e){
add(0, e);
}
// 在index索引的位置插入一个新元素e
public void add(int index, E e){
if (size==data.length)
resize(data.length*2);
if(index < 0 || index > size)
throw new IllegalArgumentException("Add failed. Require index >= 0 and index <= size.");
for(int i = size - 1; i >= index ; i --)
data[i + 1] = data[i];
data[index] = e;
size ++;
}
// 获取index索引位置的元素
public E get(int index){
if(index < 0 || index >= size)
throw new IllegalArgumentException("Get failed. Index is illegal.");
return data[index];
}
// 修改index索引位置的元素为e
public void set(int index, E e){
if(index < 0 || index >= size)
throw new IllegalArgumentException("Set failed. Index is illegal.");
data[index] = e;
}
// 查找数组中是否有元素e
public boolean judge(int e){
for (E array:data){
if (array.equals(e))return true;
}
return false;
}
// 查找数组中元素e所在的索引,如果不存在元素e,则返回-1
public int find(E e){
for (int i=0;i<size;i++){
if (data[i].equals(e))return i;
}
return -1;
}
// 从数组中删除index位置的元素, 返回删除的元素
public E delete(int index){
E element=data[index];
if(index < 0 || index >= size) throw new IllegalArgumentException("Set failed. Index is illegal.");
for (int i=index;i<size-1;i++){
data[i]=data[i+1];
}
size--;
if (size<=getCapacity()/2)
resize(getCapacity()/2);
return element;
}
// 从数组中删除第一个元素, 返回删除的元素
public E deleteFirst(){
return delete(0);
}
// 从数组中删除最后一个元素, 返回删除的元素
public E deleteLast(){
return delete(size-1);
}
// 从数组中删除元素e
public String deleteElement(E e){
int i=find(e);
delete(i);
return new String(e+"has been delete");
}
//实现动态数组
public void resize(int num){
if( num < size) throw new IllegalArgumentException("Resize failed. num is illegal.");
E[]redata=(E[]) new Object[num];
for (int i=0;i<size;i++){
redata[i]=data[i];
}
data=redata;
}
@Override
public String toString(){
StringBuilder res = new StringBuilder();
res.append(String.format("Array: size = %d , capacity = %d\n", size, data.length));
res.append('[');
for(int i = 0 ; i < size ; i ++){
res.append(data[i]);
if(i != size - 1)
res.append(", ");
}
res.append(']');
return res.toString();
}
}
2.main函数:
①实现动态数组等多功能
package com.company;
public class Main {
public static void main(String[] args) {
Array<Integer> arr = new Array<>();
for(int i = 0 ; i < 10 ; i ++)
arr.addLast(i);
System.out.println(arr);
arr.add(1, 100);
System.out.println(arr);
arr.addFirst(-1);
System.out.println(arr);
arr.remove(2);
System.out.println(arr);
arr.removeElement(4);
System.out.println(arr);
arr.removeFirst();
System.out.println(arr);
for(int i = 0 ; i < 4 ; i ++){
arr.removeFirst();
System.out.println(arr);
}
}
}
result:
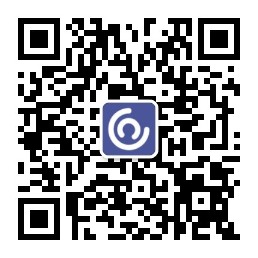
Array: size = 10 , capacity = 10
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Array: size = 11 , capacity = 20
[0, 100, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Array: size = 12 , capacity = 20
[-1, 0, 100, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Array: size = 11 , capacity = 20
[-1, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Array: size = 10 , capacity = 20
[-1, 0, 1, 2, 3, 5, 6, 7, 8, 9]
Array: size = 9 , capacity = 20
[0, 1, 2, 3, 5, 6, 7, 8, 9]
Array: size = 8 , capacity = 20
[1, 2, 3, 5, 6, 7, 8, 9]
Array: size = 7 , capacity = 20
[2, 3, 5, 6, 7, 8, 9]
Array: size = 6 , capacity = 20
[3, 5, 6, 7, 8, 9]
Array: size = 5 , capacity = 10
[5, 6, 7, 8, 9]
②实现Student的泛型操作
代码:
Student类:
public class Student {
private String name;
private int scores;
Student (String name ,int scores){
this.name=name;
this.scores=scores;
}
@Override
public String toString(){
return String.format("%s的成绩是:%d",name,scores);
}
}
main:
package IMUHERO;
public class main {
public static void main(String[] args) {
Array<Student>student=new Array<>();
student.addLast(new Student("xiaoming",100));
student.addLast(new Student("xiaoli",90));
student.addFirst(new Student("xiaoxiao",80));
System.out.println(student);
Array<String>str=new Array<>();
str.addLast("one");
str.addLast("two");
str.addLast("three");
System.out.println(str);
}
}
result:
Array: size = 3 , capacity = 10
[xiaoxiao的成绩是:80, xiaoming的成绩是:100, xiaoli的成绩是:90]
Array: size = 3 , capacity = 10
[one, two, three]