一、模块化设计 点击此处返回总目录 二、举例
一、模块化设计 以后的程序会逐渐复杂,为了体现程序的层次关系,增加程序的可复用性,大多数的程序要使用模块化的设计思想了。 我们一起来梳理一下。 forward.py //描述前向传播过程。前向传播就是搭建网络,设计网络结构。 backward.py //描述反向传播的过程。反向传播的目的是训练网络,优化网络参数。
扫描二维码关注公众号,回复:
5506795 查看本文章
//forward.py
def forward(x, regularizer): #forward()函数完成网络结构的设计。给出从输入到输出的数据通路。两个参数:输入x, w= 正则化权重regularizer. b= y= return y def get_weight(shape, regularizer): w=tf.Variable() tf.add_to_collection('losses',tf.contrib.layers.l2_regularizer(regularizer)(w)) #把每个w的正则化损失加到总损失losses中 return w def get_bias(shape): #参数是b的形状,也就是某层中b的个数。 b=tf.Variable() return b |
//backward.py
def backward(): x=tf.placeholder() y_=tf.placeholder() y=forward.forward(x,REGULARIZER) global_step = tf.Variable(0,trainable=False) loss = tf.reduce_mean(tf.square(y-y_)) #如果用均方误差损失,使用这一句。 #如果用叉熵损失,可以替换为下面两句。 ce = tf.nn.sparse_softmax_cross_entropy_with_logits(logits=y, labels=tf.argmax(y_,1)) loss = tf.reduce_mean(ce) #如果包含正则化,还要加上所有w的正则化 loss = loss + tf.add_n(tf.get_collection('losses')) #如果使用指数学习率,则加上一下代码 learning_rate = tf.train.exponential_decay( LEARNING_RATE_BASE, global_step, LEARNING_RATE_STEP, LEARNING_RATE_DECAY, staircase = True ) train_step = tf.train.GradientDescentOptimizer(learning_rate).minimizer(loss, global_step=global_step) #如果需要滑动平均,则加上以下代码 ema=tf.train.ExponentialMovingAverage(MOVING_AVERAGE_DECAY, global_step) ema_op = ema.apply(tf.trainable_variables()) with tf.control_dependencies([train_step, ema_op]): train_op =tf.no_op(name='train') with tf.Session() sess: init_op = tf.global_variables_initializer() sess.run(init_op) for i in range(STEPS): sess.run(train_step,feed_dict={x: ,y_: }) if i % 轮数==0: print() if __name__ = '__main__': backward() |
二、举例 例题:把正则化那一节的代码改成使用模块化设计方法实现一下,程序使用正则化以提高泛化性、使用指数衰减学习率加快优化的效率。 分为三个模块: generateds.py #生成数据集模块 forward.py #前向传播 backward.py #反向传播 //generateds.py
#coding:utf-8 #0导入模块,生成模拟数据集 import numpy as np import matplotlib.pyplot as plt seed = 2 def generateds(): rdm=np.random.RandomState(seed) X = rdm.randn(300,2) #样本点 Y_ = [int(x1*x1 + x2*x2 <2) for (x1,x2) in X] #标签 Y_c = [['red' if y else 'blue'] for y in Y_] #根据标签赋颜色 X= np.vstack(X).reshape(-1,2) #对X整理形状 Y_ = np.vstack(Y_).reshape(-1,1) return X,Y_,Y_c ''' plt.scatter(X[:,0],X[:,1],c=np.squeeze(Y_c)) plt.show() ''' |
//forward.py
#coding:utf-8 import tensorflow as tf def get_weight(shape, regularizer): w=tf.Variable(tf.random_normal(shape),dtype=tf.float32) tf.add_to_collection('losses',tf.contrib.layers.l2_regularizer(regularizer)(w)) return w def get_bias(shape): b = tf.Variable(tf.constant(0.01,shape=shape)) return b def forward(x,regularizer): #在forward中设计前向传播过程 w1=get_weight([2,11],regularizer) #w1为2行11列。注意shape是列表的形式给出的。正则化权重为0.01 b1=get_bias([11]) y1=tf.nn.relu(tf.matmul(x,w1)+b1) w2=get_weight([11,1],regularizer) b2=get_bias([1]) y=tf.matmul(y1,w2)+b2 #输出层不过激活函数 return y |
backward.py
#coding:utf-8 import tensorflow as tf import numpy as np import matplotlib.pyplot as plt import generateds #导入另外两个模块 import forward STEPS = 40000 BATCH_SIZE = 30 #定义一次喂入的数据为30个 LEARNING_RATE_BASE = 0.001 LEARNING_RATE_DECAY = 0.999 REGULARIZER = 0.01 def backward(): x=tf.placeholder(tf.float32,shape=(None,2)) y_=tf.placeholder(tf.float32,shape=(None,1)) X,Y_,Y_c = generateds.generateds() y=forward.forward(x,REGULARIZER) global_step = tf.Variable(0,trainable=False) learning_rate = tf.train.exponential_decay( LEARNING_RATE_BASE, global_step, 300/BATCH_SIZE, LEARNING_RATE_DECAY, staircase=True) #定义损失函数 loss_mse = tf.reduce_mean(tf.square(y-y_)) #均方误差损失函数 loss_total = loss_mse + tf.add_n(tf.get_collection('losses')) #均方误差损失函数,加上每一个正则化w的损失 train_step = tf.train.AdamOptimizer(learning_rate).minimize(loss_total) #包含正则化 with tf.Session() as sess: init_op = tf.global_variables_initializer() sess.run(init_op) for i in range(STEPS): start = (i*BATCH_SIZE)%300 end= start + BATCH_SIZE sess.run(train_step,feed_dict={x:X[start:end],y_:Y_[start:end]}) if i% 2000 ==0: loss_v = sess.run(loss_total,feed_dict={x:X,y_:Y_}) print("after %d steps,loss is %f" % (i,loss_v)) xx,yy=np.mgrid[-3:3:0.01,-3:3:0.01] grid = np.c_[xx.ravel(),yy.ravel()] probs = sess.run(y,feed_dict={x:grid}) probs = probs.reshape(xx.shape) plt.scatter(X[:,0],X[:,1],c=np.squeeze(Y_c)) plt.contour(xx,yy,probs,levels=[.5]) #给所有值为0.5的点上色 plt.show() if __name__=='__main__': backward() |
运行结果: 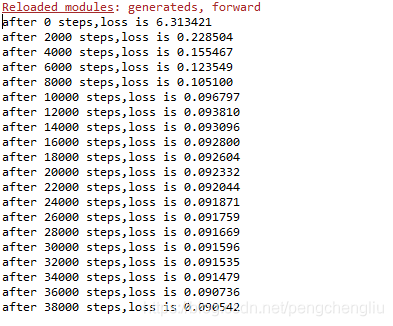 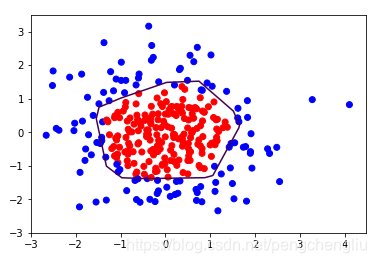 |