在以前,开发者需要为每一个需要调度的任务编写一个
Cron
条目,这是很让人头疼的事。你的任务调度不在源码控制中,你必须使用SSH
登录到服务器然后添加这些Cron
条目。Laravel
命令调度器允许你平滑而又富有表现力地在Laravel
中定义命令调度,并且服务器上只需要一个Cron
条目即可。
任务调度又是我们俗称的“计划任务”
任务调度定义在app/Console/Kernel.php
文件的schedule
方法中,该方法中已经包含了一个示例。你可以自由地添加你需要的调度任务到Schedule
对象
1.开启调度
在linux环境中执行
* * * * * root php /var/www/laravel/artisan schedule:run
/var/www/laravel
为你的项目目录
该Cron
将会每分钟调用Laravel
命令调度,然后,Laravel
评估你的调度任务并运行到期的任务。
2.定义调度
2.1
在项目根目录中 下创建定时任务所需要进行的操作
创建命令:php artisan make:console Stat_Test
该操作会在app/Console/Commands
下生成一个Stat_Test.php
扫描二维码关注公众号,回复:
5428717 查看本文章
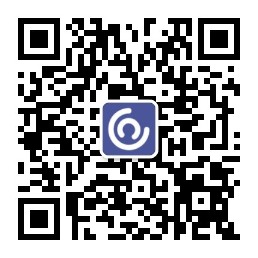
下面打开该文件 给大家展示一个简单而又完整的代码例子
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class Stat_Test extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'stat:test';
/**
* The console command description.
*
* @var string
*/
protected $description = 'stat:test';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
$this->addData();
}
// 小例子
public function addData(){
$time = time();
$rand = rand(1,1000);
$id = \DB::table('data_test')->insertGetId(['uuid'=>$time,'uuid'=>$rand]);
if($id){
\Log::info('定时/数据插入成功.',$id);
}else{
\Log::error('定时/数据插入失败.',$time);
}
}
}
值得注意的是 这个文件中的
$signature = 'stat:test'
这个签名在Kernel.php
中也要相应用到
下面也附上Kernel.php
的完整代码
<?php
namespace App\Console;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
class Kernel extends ConsoleKernel
{
/**
* The Artisan commands provided by your application.
*
* @var array
*/
protected $commands = [
\App\Console\Commands\Inspire::class,
'\App\Console\Commands\Stat_Test',
];
/**
* Define the application's command schedule.
*
* @param \Illuminate\Console\Scheduling\Schedule $schedule
* @return void
*/
protected function schedule(Schedule $schedule)
{
// 为测试方便 每分钟执行一次
$schedule->command('stat:test')->everyMinute();
}
}
再强调一次 $schedule->command('stat:test')
里面的stat:test必须和上面的签名 $signature = 'stat:test'
对应上
protected $commands = [
\App\Console\Commands\Inspire::class,
'\App\Console\Commands\Stat_Test',
];
也要把引入你的调度文件
// 每周星期六11:00运行一次...
$schedule->command('stat:test')->weekly()->saturdays()->at('11:00');
// 每周星期一:00运行一次...
$schedule->command('stat:test')->weekly()->->mondays()->at('01:00');
2.2 调度常用选项
当然,你可以分配多种调度到任务
->cron('* * * * *'); 在自定义Cron调度上运行任务
->everyMinute(); 每分钟运行一次任务
->everyFiveMinutes(); 每五分钟运行一次任务
->everyTenMinutes(); 每十分钟运行一次任务
->everyThirtyMinutes(); 每三十分钟运行一次任务
->hourly(); 每小时运行一次任务
->daily(); 每天凌晨零点运行任务
->dailyAt('13:00'); 每天13:00运行任务
->twiceDaily(1, 13); 每天1:00 & 13:00运行任务
->weekly(); 每周运行一次任务
->monthly(); 每月运行一次任务
下面是额外的调度约束列表:
->weekdays(); 只在工作日运行任务
->sundays(); 每个星期天运行任务
->mondays(); 每个星期一运行任务
->tuesdays(); 每个星期二运行任务
->wednesdays(); 每个星期三运行任务
->thursdays(); 每个星期四运行任务
->fridays(); 每个星期五运行任务
->saturdays(); 每个星期六运行任务
->when(Closure); 基于特定测试运行任务