单链表是链表中结构最简单的。一个单链表的节点(Node)分为两个部分,第一个部
分(data)保存或者显示关于节点的信息,另一个部分存储下一个节点的地址。最后一个
节点存储地址的部分指向空值。
单向链表只可向一个方向遍历,一般查找一个节点的时候需要从第一个节点开始每次访
问下一个节点,一直访问到需要的位置。而插入一个节点,对于单向链表,我们只提供在
链表头插入,只需要将当前插入的节点设置为头节点,next指向原头节点即可。删除一个
节点,我们将该节点的上一个节点的next指向该节点的下一个节点。
节点
public class Node{
int data;
Node next;
boolean delectFlag = false ;//delectFlag为删除标记 false--存在 true--已删除
public Node(int data){
this.data=data;
}
}
添加元素
/**
*添加元素
*/
public void add(int data){
//LinkedList
Node newNode = new Node(data);
if (this.head == null){
this.head = newNode ;
}
else {
Node temp = head;
while(temp.next!=null){
temp = temp.next;
}
temp.next =newNode;
}
this.size++;
}
我的测试代码
package com.finatext;
import java.util.*;
public class LinkedList {
int size =0;
Node head = null;
/**
* 节点
*/
public class Node{
int data;
Node next;
boolean delectFlag = false ;//delectFlag为删除标记 false--存在 true--已删除
public Node(int data){
this.data=data;
}
}
/**
*添加元素
*/
public void add(int data){
//LinkedList
Node newNode = new Node(data);
if (this.head == null){
this.head = newNode ;
}
else {
Node temp = head;
while(temp.next!=null){
temp = temp.next;
}
temp.next =newNode;
}
this.size++;
}
/**
*修改指定位置的值
*/
public void alter(int data,int index){
int i=0;
Node preNode = head;
while(preNode!=null){
if(i==index){
preNode.data=data;
}
preNode = preNode.next;
i++;
}
}
/**
*查看指定位置的值
*/
public void check(int index){
Node newNode = head;
int i=0;
while(newNode!=null){
if (i==index){
System.out.println(newNode.data);
}
i++;
newNode = newNode.next;
}
}
/**
*删除指定位置的值
*/
public void delete(int index){
if (index==1){
head = head.next;
}
Node newNode = head;
Node curNode = newNode.next;
int i =1;
while (curNode!=null){
if(i==index){
newNode.next = curNode.next;
this.size--;
}
newNode = newNode.next;
curNode = curNode.next;
i++;
}
}
/**
* 链表长度
* @return
*/
public int length(){
return this.size ;
}
/**
*用删除标记删除
*/
public void delect1(int str[]){
Node preNode = this.head ;
while(preNode!=null){
for (int i=0;i<str.length;i++) {
if (preNode.data == str[i]) {
preNode.delectFlag = true;
this.size--;
}
}
preNode = preNode.next ;
}
}
public void delect2(int str[]){
Node preNode = this.head ;
while(preNode!=null){
for (int i=0;i<str.length;i++) {
if (preNode.data == str[i]) {
preNode.delectFlag = true;
this.size--;
}
}
preNode = preNode.next ;
}
}
/**
* 显示链表的值
*/
public void display(){
Node node = head;
while(node!=null ){
if (!node.delectFlag){
System.out.print(node.data+" ");
}
node = node.next;
}
System.out.println();
}
public void reversal(){
Node newNode = head;
Node preNode = null;
while(newNode!=null){
Node nextNode = newNode.next;
newNode.next = preNode;
preNode = newNode;
newNode = nextNode;
}
head = preNode;
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
LinkedList lists = new LinkedList();
int n = in.nextInt();
int str[] = new int[20];
int arr[] = new int[20];
int mar[] = new int[20];
for (int i=0;i<n;i++){
str[i] = in.nextInt();
lists.add(str[i]);
}
/* int m = in.nextInt();
for (int i=0;i<m;i++){
arr[i] = in.nextInt();
}
int p =in.nextInt();
for (int i=0;i<p;i++){
mar[i] = in.nextInt();
}*/
//lists.delect1(arr);
//lists.delect2(mar);
lists.display();
System.out.println("链表长度:"+lists.length());
lists.alter(15,3);
System.out.print("修改后的链表:");
lists.display();
System.out.print("执行删除后的链表:");
lists.delete(2);//删除位置2的值
lists.display();//输出节点
}
}
测试结果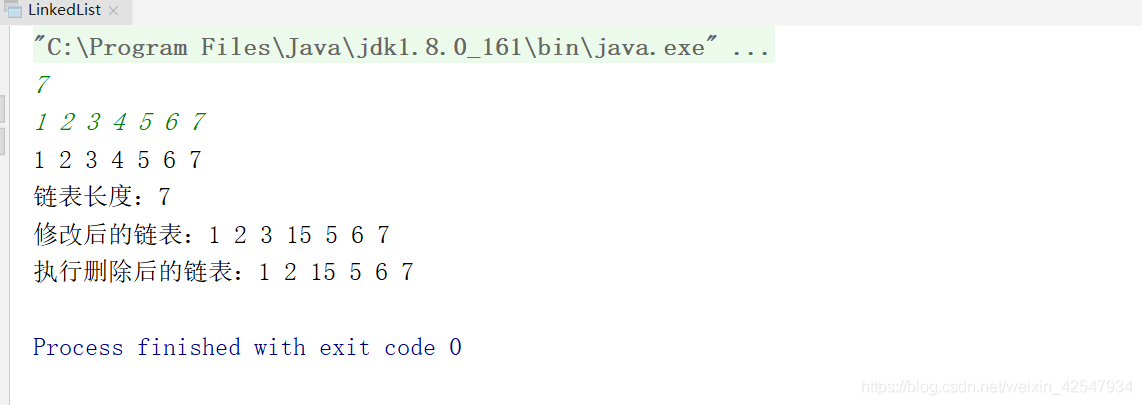
以上是我自己的运行结果,读者课根据自己的实际情况举一反三