原题
Given two words (beginWord and endWord), and a dictionary’s word list, find the length of shortest transformation sequence from beginWord to endWord, such that:
Only one letter can be changed at a time.
Each transformed word must exist in the word list. Note that beginWord is not a transformed word.
Note:
Return 0 if there is no such transformation sequence.
All words have the same length.
All words contain only lowercase alphabetic characters.
You may assume no duplicates in the word list.
You may assume beginWord and endWord are non-empty and are not the same.
Example 1:
Input:
beginWord = “hit”,
endWord = “cog”,
wordList = [“hot”,“dot”,“dog”,“lot”,“log”,“cog”]
Output: 5
Explanation: As one shortest transformation is “hit” -> “hot” -> “dot” -> “dog” -> “cog”,
return its length 5.
Example 2:
Input:
beginWord = “hit”
endWord = “cog”
wordList = [“hot”,“dot”,“dog”,“lot”,“log”]
Output: 0
Explanation: The endWord “cog” is not in wordList, therefore no possible transformation.
解法
集合+ BFS.
首先将wordList转化为集合, 尽量减少时间复杂度, 然后按层遍历, 每加一层, 长度加1. 此题的难点是找出只改变一个字母的单词, 我们使用穷举法查找, 对每一个单词的每一个字母, 换成26个字母的其中一个, 检查新单词是否在wordSet中, 如果在, 放入队列中. 此时需要从队列中去掉新单词, 以减少遍历次数和重复值.
Time: O(n*26**l), n为单词数, l为单词长度.
Space: O(n)
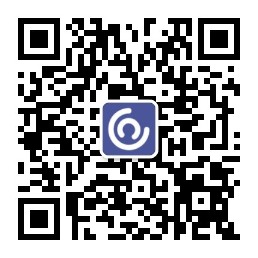
代码
class Solution(object):
def ladderLength(self, beginWord, endWord, wordList):
"""
:type beginWord: str
:type endWord: str
:type wordList: List[str]
:rtype: int
"""
# base case
if endWord not in wordList: return 0
wordSet = set(wordList)
q = [(beginWord, 1)]
while q:
word, length = q.pop(0)
if word == endWord:
return length
for i in range(len(word)):
for char in string.ascii_lowercase:
trans = word[:i] + char + word[i+1:]
if trans in wordSet:
wordSet.remove(trans)
q.append((trans, length+1))
return 0