变量、数据类型
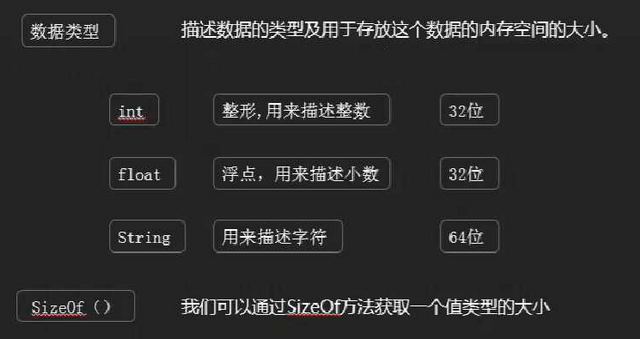
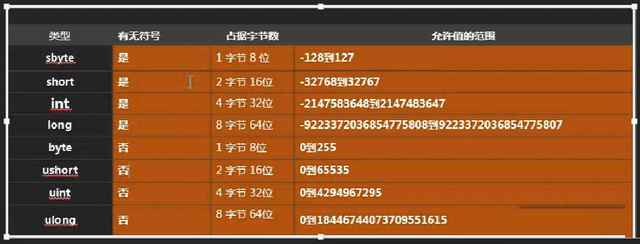
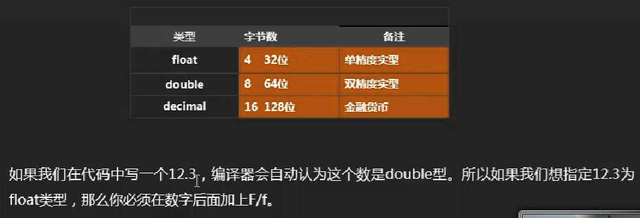
给变量赋值,如果想指定float,在数字后面加上F/f;如果想指定decimal,在数字后面加上m。
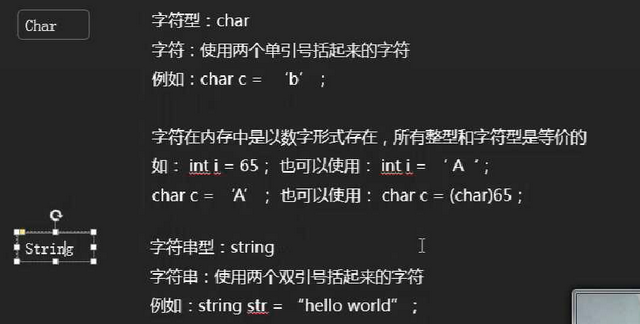
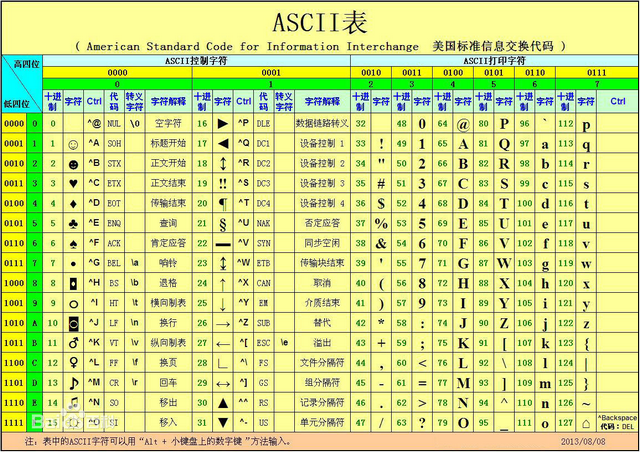
0-9:48-57
A-Z:65-90
a-z:97-122
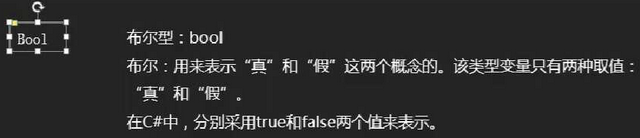
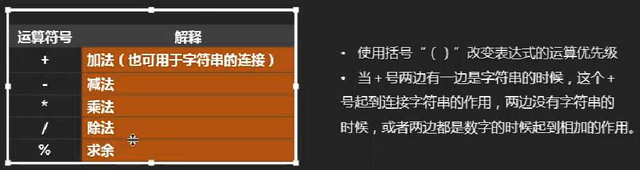

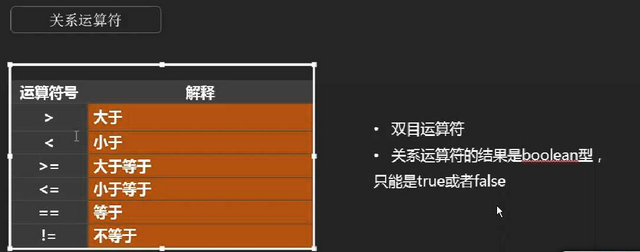
字符串格式化输出
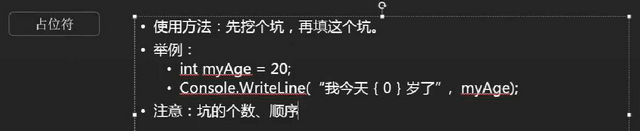
我们在使用Console.writeLine时,可以使用占位符格式化字符串
在字符串中使用{}包裹一个下标,来表示后面的变量
下标不能超过变量个数-1
数据转换
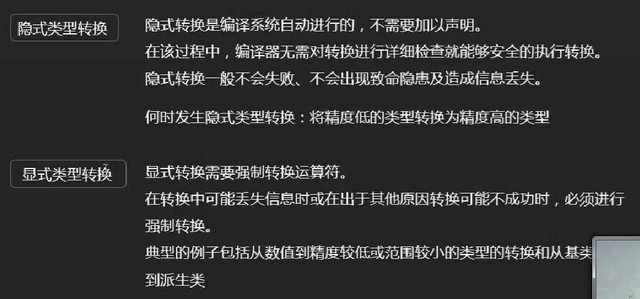
隐式类型转换,造成的信息丢失,不容易排查
显示类型转换,造成的信息丢失,会报错且容易排查
显示类型转换的典型例子:高精度转换为低精度,父类与派生类之间的转换
convert转换,用于基本类型到基本类型之间的转换
Parse转换,用于字符串到特定类型之间的转换,功能更强大,可转IP地址
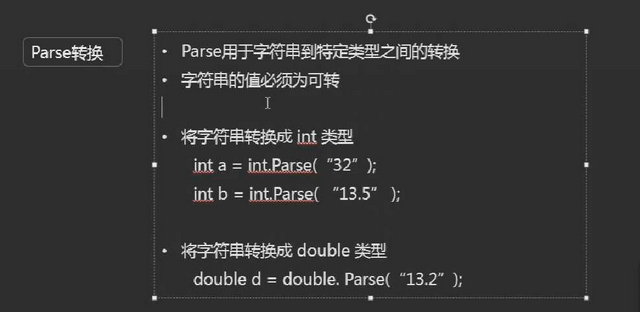
强制转换符,用于数值类型、派生关系之间的转换
char字符类型也是数值类型,ASC码表
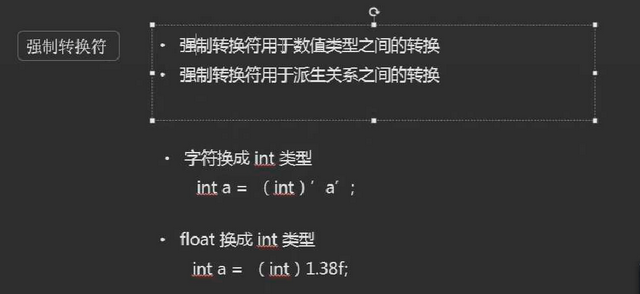
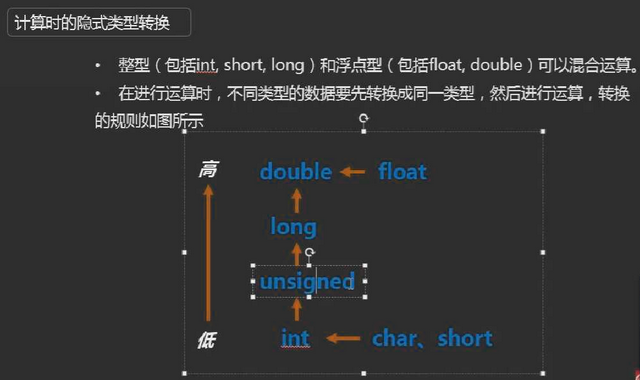
using System.Text; using System.Threading.Tasks; namespace w1d3_namespace { class Program { static void Main(string[] args) { #region 基本数据类型 //基本类型 //整型 int num = 10; long longNum = 10; Console.WriteLine(longNum); //小数型 float floatNum = 10.0f; double doubleNum = 10.0; decimal decimalNum = 10.0m; //字符 char cha = 'a'; string str = "abc";//引用类型,是一种特殊的引用类型 //布尔 bool isTrue = true; bool isFalse = false; Console.WriteLine("sizeof(int)=" + sizeof(int)); Console.WriteLine("sizeof(long)=" + sizeof(long)); Console.WriteLine("sizeof(float)=" + sizeof(float)); Console.WriteLine("sizeof(double)=" + sizeof(double)); Console.WriteLine("sizeof(decimal)=" + sizeof(decimal)); Console.WriteLine("sizeof(char)=" + sizeof(char)); //Console.WriteLine(sizeof(string));不能打出字符串,因为是引用类型 Console.WriteLine("sizeof(bool)=" + sizeof(bool)); #endregion #region 基本数据类型练习 double num1 = 36.6; double num2 = 36.6f; Console.WriteLine("num1=" + num1); Console.WriteLine("num2=" + num2); Console.WriteLine("请输入你的年龄"); string myYear; myYear = Console.ReadLine(); Console.WriteLine("十年后你的年龄" + (10 + myYear)); //小明的数学考试成绩是80,语文考试成绩是78,英文的考试成绩是98,请用变量描述 //数据 //小明 变量 数据类型 字符串 变量名 name //数学考试成绩 变量 字符串 变量名mathSorce //语文考试成绩 变量 字符串 变量名chineseSorce //英文考试成绩 变量 字符串 变量名englishSorce Console.WriteLine("请输入你的名字"); string name = Console.ReadLine(); Console.WriteLine("请输入你的数学考试成绩"); string mathSorce = Console.ReadLine(); Console.WriteLine("请输入你的语文考试成绩"); string chineseSorce = Console.ReadLine(); Console.WriteLine("请输入你的英文考试成绩"); string englishSorce = Console.ReadLine(); Console.WriteLine(name + "数学考试成绩:" + mathSorce + ",语文考试成绩:" + chineseSorce + ",英文考试成绩:" + englishSorce); //字符类型 int i1 = 65; int i2 = 'A'; char c1 = 'A'; char c2 = (char)65; Console.WriteLine("i1=" + i1); Console.WriteLine("i2=" + i2); Console.WriteLine("c1=" + c1); Console.WriteLine("c2=" + c2); Console.WriteLine("依次输入学生姓名"); Console.WriteLine("C#语言成绩"); string cSharp = Console.ReadLine(); int cSharp1 = Convert.ToInt16(cSharp);//强制类型转换 Console.WriteLine("Unity的成绩"); string unity = Console.ReadLine(); int unity1 = Convert.ToInt16(unity); Console.WriteLine("你的总分是" + (cSharp1 + unity1)); #endregion #region 运算符 //运算符 //用来处理数据的一种逻辑 #endregion #region 算数运算符 //+,-,*,/,% //用于数值类型的算术运算 // + 可以用于字符串的连接, - 不可以 int math = 80; int english = 78; int chinese = 90; int sum = math + english + chinese; Console.WriteLine("总分是" + sum); Console.WriteLine("数学比英语多" + (math - english));//减号不可以连接字符串,要用括号括起来先计算 Console.WriteLine("四五得" + (4 * 5));//建议所有的运算,全部都用括号括起来,不要记忆运算符的优先级,用括号 Console.WriteLine("二十除以六" + (20 / 6));//如果你需要得到一个高精度的结果,请使用高精度数据参与运算 Console.WriteLine("二十除以六" + (20f / 6f));//float Console.WriteLine("二十除以六" + (20.0 / 6.0));//double Console.WriteLine("二十余六" + (20 % 6));//求余用整数 #endregion #region 算数运算符的练习 //string guestName; //string guestYear; //Console.WriteLine("请输入你的名字"); //string guestName = Console.ReadLine(); // Console.WriteLine("请输入你的年龄"); //string guestYear = Console.ReadLine(); //Console.WriteLine(guestName + "+" + guestYear + "岁了"); Console.WriteLine("请输入你的姓名"); string guestName = Console.ReadLine(); Console.WriteLine("请输入你的年龄"); string guestYear = Console.ReadLine(); Console.WriteLine("请输入你的邮箱"); string guestMail = Console.ReadLine(); Console.WriteLine("请输入你的家庭住址"); string guestAdress = Console.ReadLine(); Console.WriteLine("请输入你的期望工资"); string guestManey = Console.ReadLine(); Console.WriteLine(guestName + "," + guestYear + "," + guestMail + "," + guestAdress + "," + guestManey); #endregion #region 赋值运算符 //=,+=,-=,*=,/= //= 是将右边的值(数据)赋值给左边的变量,类型必须一致 //+= int age = 18; age = age + 10; Console.WriteLine(age); age += 10;//数据自身参与运算,且只有一个运算 Console.WriteLine(age); int age1; age1 = (int)Convert.ToDouble(age) / 10; Console.WriteLine(age); age /= 10; Console.WriteLine(age); age = age % 10; Console.WriteLine(age); age %= 10; Console.WriteLine(age); #endregion #region 自增减运算符 //++ -- int age = 0; age = age + 1; age += 1; //++ 相当于变量自身做加一的运算 //++ 相当于变量自身做减一的运算 //当自增减运算符作为前缀时,先运算,再将结果交给使用者 //当自增减运算符作为后缀时,先将数据交给使用者,后运算 //如果你想回避前后缀问题,使用自增减运算符时,就只运算,不参与使用 age = 0; Console.WriteLine(++age);//age++;先运算后加减 //运算结果是1,打印出来是1 age = 0; Console.WriteLine(age++);//++age;先加减后运算 //运算结果是1,打印出来是0 #endregion #region 关系运算符 双目运算符 //>,<,==,!=,>=,<= //用于数值类型之间的关系比较 //用于处理左右两边数据的关系 //如果关系达成,返回一个布尔值为true值 //如果关系不达成,返回一个布尔值为false值 bool result = true; result = 10 > 20; Console.WriteLine(result); #endregion #region 运算符练习 //小李有20个苹果,小王有10个苹果,他们想和对方交换,请实现 int xiaoLi = 20; int xiaoWang = 10; int exchange = 0; Console.WriteLine("小李的苹果有" + xiaoLi + ",小王的苹果有" + xiaoWang); //如果我们要交换两个变量的数据 //先将一方的数据保存到一个临时变量 exchange = xiaoLi; xiaoLi = xiaoWang; xiaoWang = exchange; Console.WriteLine("交换后"); //Console.WriteLine("小李的苹果有" + xiaoLi + ",小王的苹果有" + xiaoWang); Console.WriteLine("小李的苹果有{0},小王的苹果有{1}", xiaoLi, xiaoWang); //计算任意三门成绩的总分、平均分 Console.WriteLine("请输入第一门的成绩"); string score1 = Console.ReadLine(); double score11 = Convert.ToDouble(score1); Console.WriteLine("请输入第二门的成绩"); string score2 = Console.ReadLine(); double score22 = Convert.ToDouble(score2); Console.WriteLine("请输入第三门的成绩"); string score3 = Console.ReadLine(); double score33 = Convert.ToDouble(score3); double sum = score11 + score22 + score33; double aver = sum / 3; //Console.WriteLine("总分是" + sum + ",平均分是" + aver); //我们在使用Console.writeLine时,可以使用占位符格式化字符串 //在字符串中使用{}包裹一个下标,来表示后面的变量 //下标不能超过变量个数-1 Console.WriteLine("总分是{0},平均分是{1}", sum, aver);//字符串格式化输出 //计算一个半径为5的圆的面积和周长 //float r = 5; //float PI = 3.1415926f; //float perimeter = 2 * r * PI; //float area = PI * r * r; Console.WriteLine("请输入半径"); string radius = Console.ReadLine(); double r = Convert.ToDouble(radius); double PI = 3.1415926f; double perimeter = 2 * r * PI; double area = PI * r * r; //Console.WriteLine("圆的面积是" + area + "圆的周长是" + perimeter); Console.WriteLine("圆的面积是{0},圆的周长是{1}", area, perimeter); //某商店T恤的价格为285元/件,裤子的价格为720元/条,小李在该店买了2件T恤和3条裤子,请计算小李该付多少钱?打3.8折后呢? Console.WriteLine("T恤的价格为285元/件,裤子的价格为720元/条"); Console.WriteLine("请输入T恤件数"); string tShirt = Console.ReadLine(); double tShirt1 = Convert.ToDouble(tShirt); Console.WriteLine("请输入裤子件数"); string pants = Console.ReadLine(); double pants1 = Convert.ToDouble(pants); double price = tShirt1 * 285 + pants1 * 720; double discount = tShirt1 * 285 + pants1 * 720; //Console.WriteLine("原价为" + price + ",打折为" + discount); Console.WriteLine("原价是{0},打折是{1}", price, discount); #endregion #region 字符串格式化输出 //我们在使用Console.writeLine时,可以使用占位符格式化字符串 //在字符串中使用{ } //包裹一个下标,来表示后面的变量 //下标不能超过变量个数 - 1 #endregion #region 数据类型转换 //隐式转换 //系统默认发生的,将低精度向高精度的类型转换 //如果你需要结果是高精度,数据源尽量保持高精度 //显式转换 //我们调用一些指令对数据进行转换 #endregion #region Convert //Convert(显式转换) //用于所有基本类型之间的转换 //用户输入姓名,语文,数学,英语的成绩,最后在控制台显示:XXX,你的总成绩为XX分,平均分是XX分 Console.WriteLine("请输入您的姓名"); string name = Console.ReadLine(); Console.WriteLine("请输入您的语文成绩"); string inputChinese = Console.ReadLine(); float chinese = Convert.ToSingle(inputChinese);//float的显式转换用single表示 Console.WriteLine("请输入您的数学成绩"); float math = Convert.ToSingle(Console.ReadLine()); Console.WriteLine("请输入您的英语成绩"); float english = Convert.ToSingle(Console.ReadLine()); float sum = chinese + math + english; float aver = sum / 3; Console.WriteLine("您的总分是{0},平均分是{1}", sum, aver); Console.WriteLine(1.1 + 2.2 + 3.3); Console.WriteLine(1.1 + 2.2 + 3.3f);//隐式转换数据丢失 Console.WriteLine(1.1 + 2.2 + Convert.ToSingle(3.3f));//显式转换数据丢失 int age1 = Convert.ToInt16('A'); Console.WriteLine(age1); //int age2 = Convert.ToInt16("AAA");//Convert的值必须可转 //Console.WriteLine(age2); Console.WriteLine(111 + 111 + "");// Console.WriteLine("" + 111 + 111);//字符串转换,如果加号左边是字符,默认将右边的类型转成字符串 #endregion #region Parse转换 //将字符串转换成一个特定类型 //由特定类型调用 //提示用户输入一个数字,接收并计算这个数字的1.5倍 Console.WriteLine("请输入一个整数"); int num1 = int.Parse(Console.ReadLine()); Console.WriteLine(num1 * 1.5f); Console.WriteLine("请输入一个数字"); float num2 = float.Parse(Console.ReadLine()); Console.WriteLine(num2 * 1.5f); Console.WriteLine("请输入一个数字"); double num3 = double.Parse(Console.ReadLine()); Console.WriteLine(num3 * 1.5f); Console.WriteLine("请输入一个数字"); decimal num4 = decimal.Parse(Console.ReadLine()); Console.WriteLine(num4 * 1.5m); #endregion #region 强制转换符 //()把需要转到的类型填在括号里,把需要转换的数据放在括号后面 //用于数值类型(整型,浮点型,char)之间的转换 //用于派生(继承)关系之间的转换 int a = (int)'a'; Console.WriteLine(a); int b = (int)1.35f; Console.WriteLine(b);//强制转换,精度丢失 //将一个char转换成float float c = (float)'c'; Console.WriteLine(c); // float d = (int)'d'; Console.WriteLine(d); #endregion } } }