1、let 允许重复赋值,但不允许重复声明变量
2、const 声明常量,不允许重复声明,也不允许重复赋值
3、字符串
(1)String.fromCharCode(编码),能让编码转成字符
示例,统计浏览器能支持多少个中文字符
let name = '';
for(let i=0x4e00;i<0x9fa5;i++) {
name += String.fromCharCode(i);
}
console.log(name.length);
(2)repeat接受一个参数,参数表示字符串重复的次数
let v = 'dqedw';
console.log(v.repeat(5));
//结果:dqedwdqedwdqedwdqedw
(3)includes,有点像indexOf,第一个参数表示字符,第二个参数表示从第几位开始查找,需要注意的是位置是从0开始。
let n = 'werewq';
console.log(n.includes('w'));//true
console.log(n.includes('w',4))//true
console.log(n.includes('w',5))//false
(4)startsWith 开始位置,只有一个参数的情况:是字符串的开头字符的话,就返回true,否则返回false。
如果有第二个参数(可选参数),就表示从第几位开始,找与第一个参数匹配的字符,找到就返回true,否则返回false。位置数字从0开始
let d ='salqwee00';
console.log(d.startsWith('s')); //true
console.log(d.startsWith('a')); //false
console.log(d.startsWith('a',1)) //true 位数从0开始
(5)endsWith 终止位置,只有一个参数的情况:第一个参数如果是字符串的最后一个字符的话,就返回true。
如果有第二个参数(可选参数),就表示从第几位开始,位置从1开始【不知官方的解释是否是这样,这只是笔者的试验后的见解】
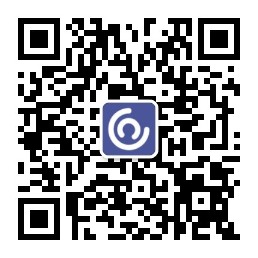
let d ='salqwee';
console.log(d.endsWith('e');//true
console.log(d.endsWith('e',7);//true
console.log(d.endsWith('e',8);//false
(6)es6中的字符串表示法不同于之前版本的,而是使用反单引号来表示字符串:``。如果要拼串,则使用${}模板来表示。
let a = `asd`;
let fc = 'qwwq${a+a}';
另外,反单引号还有一种奇特的用法,就是可以用来代替方法调用中的括号。
function show (e) {
alert(`hello`)
}
console.log(show``);
4、数组的find方法
es6提供了一个使用数组时较好用的方法find,find方法里面的参数是一个函数,函数一共支持三个参数,第一个参数表示数组元素,第二个参数表示数组的元素索引,第三个参数表示数组
[1,2,3,4,5].find(function(x,y,z){
console.log(x);//1,2,3,4,5
console.log(y);//0,1,2,3,4
console.log(z);//[1,2,3,4,5]
console.log(z[y]);//1,2,3,4,5
})
5、箭头函数
(1)es6中的箭头函数与之前版本的函数声明相同,所以它有一个缺点就是调用函数必须在声明的函数之后
x = x => x;
console.log(x);//返回 x => x;
//相当于
var x = function (x) {
return x;
}
console.log(x); //返回 f(x){return x};
(2)匿名函数
var x = 10;
(a => {
console.log(a)
})(x)
(3)扩展参数: x=5 用于:传递参数时,使用传递过来的参数值,不传递参数时,则用扩展的参数值
function show (x=5) {
console.log(x);
}
show()//5
show(1)//1
//使用箭头函数可以用匿名函数的方式
((x=5) => {
console.log(x);
})();//这种是没有传递参数的情况,返回5
((x => {
console.log(x);
}))(1);//1
6、扩展符[...]
作用:合并数组、函数参数中表示数组
(1)合并数组
var a = [1];
var b = [2];
var c = [3];
console.log([...a,...b,...c]);
//相当于
console.log(a.concat(b.concat(c)));
(2)函数参数中表示数组,以前版本的函数中,参数的arguments只是类数组,而在参数中用了扩展符后,则参数表示为数组
((...x) => {
console.log(x);
})(1,2,3,4);
//能够返回数组[1,2,3,4]
//如果没有...扩展符,则只会返回第一个参数
7、生成器函数 yield,get 、set
function* show() {
yield function () {
console.log(1);
}
yield function () {
console.log(2);
}
yield function () {
}
}
let p = show();
p.next().value();
p.next().value();
p.next().value();
//依次执行yield返回的函数
var s = {
set fun (x) {
console.log(x);
},
get fun () {
return {
get fun2 () {
return 'fun2';
}
}
}
}
s.fun = 1;//调用的是set方法
console.log(s.fun.fun2);//调用的是get方法