类:就是将一类事物共有的属性和能力进行封装,类是抽象的,具体的事物 还需要对类进行实例化。
简单示例:共有的 年龄,体重等等
class Dog {
public://修饰符,判断该类的某些变量和方法是否能被外部访问
int age;
int wight;
void getwigth() {
cout << "dog:" << wight << endl;
}
void getage() {
cout << "dog:" << age << endl;
}
};
//对类进行实例化的两种方式
//第一种:直接拿到类,对类中的变量和方法进行操作,
//直接通过类名点来进行操作(自动分配内存空间,系统自动回收内存)。
Dog dg;
dg.age = 5;
dg.wight = 50;
dg.getage();
dg.getwigth();
//第二种:通过new来创建类(给类分配内存空间)
//对类中变量和方法 通过->来进行操作,
//注:通过new创建的对象,使用后要手动delete,并且质为NULL
Dog *d = new Dog();
if (d == NULL) {
//申请内存失败
return 0;
}
d->age = 10;
d->wight = 60;
d->getage();
d->getwigth();
delete d;
d == NULL;
String:
使用时一定要将string的头文件放进去。
#include "string"
注:在c++中两字符串拼接,“hello”+"world"这种是不行的。下面几种是可以的
string name("hello");
cout << name << endl;
cout << name + "world" << endl;
cout << "hai" + name + "world" << endl;
内存分区:
栈区:int x=10;
堆区:int *p = new int[10];
全局区:存储全局变量和静态变量;
常量区:string str = "hello";
扫描二维码关注公众号,回复:
5107059 查看本文章
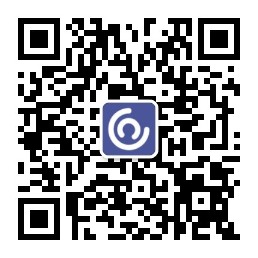
代码区:存储逻辑代码的二进制;
对象数组(大量数据):
堆中实例化的数组需要手动销毁释放内存,在栈中实例化的数组,系统自动回收内存
例:实现一个长度为三的数组,并循环打印
class Coordinates {
public:
Coordinates();
~Coordinates();
public:
int m_ix;
int m_iy;
};
Coordinates::Coordinates() {
cout << "coordinate" << endl;
}
Coordinates::~Coordinates() {
cout << "~coordinate" << endl;
}
//对象数组
//栈中实例化
Coordinates coor[3];//执行了构造函数
//给第一个赋值
coor[0].m_ix = 10;
coor[0].m_iy = 20;
//堆中实例化
Coordinates *p = new Coordinates[3];//执行了构造函数
//两种赋值方式,第一个元素
p->m_ix = 30;
p[0].m_iy = 40;
p++;//指向第二个元素
p->m_ix = 31;
p[0].m_iy = 41;
p++;
p->m_ix = 32;
p[0].m_iy = 42;
for (int i = 0; i < 3; i++)
{
cout << "coor_x:" << coor[i].m_ix << endl;
cout << "coor_y:" << coor[i].m_iy << endl;
}
for (int j = 0; j < 3; j++)
{
cout << "p_x:" << p->m_ix << endl;
cout << "p_y:" << p->m_iy << endl;
p--;
}
p++;//前面进行循环打印,对p进行了--操作,在下面进行内存释放需要将p指针 指向数组内存位置
//堆中实例化的数组需要手动销毁释放内存,在栈中实例化的数组,系统自动回收内存
//释放内存,执行了析构函数(~Coordinates())
delete []p;
p = NULL;
对象成员:
示例:线(实体类),点(实体类),两点连接成线,实体类嵌套,看两实体类的创建和销毁的执行步骤(查看对象成员和对象自己两个对象之间创建和销毁的执行流程)
线:
#pragma once
#include "Coordinate.h"
class Line
{
public:
Line(int x1,int y1,int x2,int y2);
~Line();
public:
void setCoordA(int x1,int y1);
void setCoordB(int x2,int y2);
Coordinate getCoordA();
Coordinate getCoordB();
void printInfo();
private:
Coordinate m_coordA;
Coordinate m_coordB;
};
#include "stdafx.h"
#include "Line.h"
#include "iostream"
using namespace std;
//线
Line::Line(int x1,int y1,int x2,int y2):m_coordA(x1,y1),m_coordB(x2,y2)
{
cout << "line" << endl;
}
Line::~Line()
{
cout << "~line" << endl;
}
void Line::setCoordA(int x1,int y1) {
m_coordA.setX(x1);
m_coordA.setY(y1);
}
void Line::setCoordB(int x2,int y2) {
m_coordB.setX(x2);
m_coordB.setY(y2);
}
Coordinate Line::getCoordA() {
return m_coordA;
}
Coordinate Line::getCoordB() {
return m_coordB;
}
void Line::printInfo() {
cout << "(" <<m_coordA.getX()<<","<<m_coordA.getY()<< ")" << endl;
cout << "(" <<m_coordB.getX() << "," << m_coordB.getY() << ")" << endl;
}
点:
#pragma once
class Coordinate
{
public:
Coordinate(int x, int y);
~Coordinate();
public:
void setX(int x);
void setY(int y);
int getX();
int getY();
private:
int m_ix;
int m_iy;
};
#include "stdafx.h"
#include "Coordinate.h"
#include "iostream"
using namespace std;
//点
Coordinate::Coordinate(int x, int y)
{
cout << "Coordinate" << endl;
m_ix = x;
m_iy = y;
}
Coordinate::~Coordinate()
{
cout << "~coordinate" << endl;
}
void Coordinate::setX(int x) {
m_ix = x;
}
void Coordinate::setY(int y) {
m_iy = y;
}
int Coordinate::getX() {
return m_ix;
}
int Coordinate::getY() {
return m_iy;
}
调用:
Line *p = new Line(2,5,8,0);
if (p == NULL) {}
p->printInfo();
delete p;
p = NULL;
打印结果:
深拷贝、浅拷贝
浅拷贝:就是将值直接考过去的赋值方式(不改变值对应的内存地址,这种拷贝方式在多个值拷贝后,释放内存时会报错(多次释放到同一内存空间));
Array::Array(int count)
{
m_iCount = count;
m_pArr = new int[count];
}
Array::Array(const Array&arr) {
m_iCount = arr.m_iCount;
m_pArr = arr.m_pArr;
}
深拷贝:重新分配一个内存空间,赋值到新分配的内存空间
Array::Array(int count)
{
m_iCount = count;
m_pArr = new int[count];
}
Array::Array(const Array&arr) {
m_iCount = arr.m_iCount;
m_pArr = new int[m_iCount];//深拷贝,分配内存
for (int i = 0; i < m_iCount; i++)
{
m_pArr[i] = arr.m_pArr[i];
}
}
对象指针
就是将一个对象用指针的方式来进行操作
//两种方式都可以,Coorinate是自定义的一个对象
Coordinate *p1 = new Coordinate;
Coordinate *p2 = new Coordinate();
//两种赋值方式都可以
p1->m_ix = 10;
p1->m_iy = 20;
(*p2).m_ix = 40;
(*p2).m_iy = 60;
//两种取值方式
cout << p1->m_ix + p2->m_ix << endl;
cout << (*p1).m_iy + (*p2).m_iy << endl;
//在堆中申请内存 记得释放
delete p1;
p1 = NULL;
delete p2;
p2 = NULL;
对象成员指针:就是在对象中嵌套的对象(嵌套的对象通过指针的形式在堆中分配内存),对象成员指针需要注意,实例化时需要通过new来进行操作,在析构函数中就分配的内存进行回收。
this指针:本质-》所在对象的地址
同java的this一样;主要目的是为了区分同名变量是否属于该类的(例如:初始化赋值时,两个变量是一样的就需要用this指针来进行区分);