error msg:
HttpClient throws TaskCanceledException on timeout
HttpClient is throwing a TaskCanceledException on timeout in some circumstances. This is happening for us when the server is under heavy load. We were able to work around by increasing the default timeout. The following MSDN forum thread captures the essence of the experienced issue:
error code:
var c = new HttpClient(); try { c.Timeout = TimeSpan.FromMilliseconds(10); var x = await c.GetAsync("http://linqpad.net"); } catch(TaskCanceledException ex) { Console.WriteLine("should not see this type of exception!"); Console.WriteLine(ex.Message); }
Surely this should be throwing a WebException? This behaviour makes it difficult to tell the difference between timeout exceptions & legit cancellations!
1.
Did you ever thik about doing what I did below?
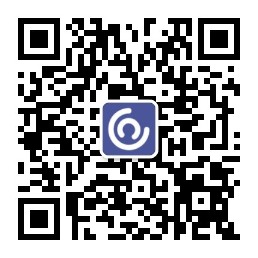
var c = new HttpClient();
try
{
c.Timeout = TimeSpan.FromMilliseconds(10);
}
catch(TaskCanceledException ex)
{
Console.WriteLine("should not see this type of exception!");
Console.WriteLine(ex.Message);
}
try
{
var x = await c.GetAsync("http://linqpad.net");
}
catch(TaskCanceledException ex)
{
Console.WriteLine("should not see this type of exception!");
Console.WriteLine(ex.Message);
}
2.
Hmm. I'm not sure if you understand the problem (or I don't understand your answer!)
I've moved the code where I set the timeout. The TaskCanceledException is thrown from the call to GetAsync.
var c = new HttpClient(); c.Timeout = TimeSpan.FromMilliseconds(10); try { var x = await c.GetAsync("http://linqpad.net"); } catch(TaskCanceledException ex) { Console.WriteLine("should not see this type of exception!?"); Console.WriteLine(ex.Message); }
I don't think this is a very good API. It shouldn't throw a TaskCanceledException unless the caller supplies a CancelationToken and calls Cancel! It should be a WebException.
3.
You code has four diffent Network layers where an error can occur
1) You first have to interface with a socket on the local computer.
2) A TCP connection has to complete from a socket with an IP address on your computer to an IP address on the server
3) On top of TCP and HTTP negotiation has to occur. You may need credentials (cookies, certifications, login) for this to complete.
4) Then the webpage has to run which sends data back to your computer.
Some of the above errors will actually return error strings, other errors may cause the application to hang causing a time out. Some errors will occur in less than 10msec. 10msec is one timer tick and the timer ticks on a computer don't run exactly at 10msec. If you are having an error that is occuring that quickly it is probably on yhour local host.
Try putting the URL into a webbrowser to see what errors you get with a webbrower. If the webbrowser works then you are mising an instruction in your code to use credentials. See this webpage
I'm not sure if you should be using the default credentials or using a specific proxy setting
4.
Hi Joel,
I'm sorry but I don't think you've understood the post. I know why the code throws an exception, it is because the HTTP request can't be serviced within the specified timeout period (if you increase the timeout the request is serviced). The post is about the type of exception that is being raised.
I'm suggesting that it shouldn't be a TaskCanceledException but a WebException!
Does that make sense?
5.
For me the point is that as good developers we check the msdn documentation for possible exceptions and catch only those. In this case we should actually be catching a WebException (http://msdn.microsoft.com/en-us/library/system.net.http.httpclient.timeout.aspx).
I guess what James is saying is that either the documentation is wrong or there is a bug. Unless, that is, theres some other documentation/rule/recommendation somewhere that recommends we warp all async calls in a try/catch(TaskCancelledException) and I doubt there is.
7.
Exactly! (Thanks Stelrad)
The caller should be provided with a detailed WebException.
*update* I've update the question replaced TimeoutException -> WebException
8.
Just to ram the point home... if you wanted to support cancellation and implement an interesting WebException handling routine (for example a retry policy), you might end up with the following abomination.
var c = new HttpClient(); c.Timeout = TimeSpan.FromMilliseconds(10); var cts = new CancellationTokenSource(); try { var x = await c.GetAsync("http://linqpad.net", cts.Token); } catch(WebException ex) { // handle web exception } catch(TaskCanceledException ex) { if(ex.CancellationToken == cts.Token) { // a real cancellation, triggered by the caller } else { // a web request timeout (possibly other things!?) } }
I find it hard to believe that this is by design.
9.
Whether the right design or not, by design OperationCanceledExceptions are thrown for timeouts (and TaskCanceledException is an OperationCanceledException).
10.
Our team found this unintuitive, but it does seem to be working as designed. Unfortunately, when we hit this, we wasted a bit of time trying to find a source of cancelation. Having to handle this scenario differently from task cancelation is pretty ugly (we created custom handler to manage this). This also seems to be an overuse of cancelation as a concept.
Thanks again for thee quick response.
11.
This is a bad design IMO. There's no way to tell if the request was actually canceled (i.e. the cancellation token passed to SendAsync
was canceled) or if the timeout was elapsed. In the latter case, it would make sense to retry, whereas in the former case it wouldn't. There's a TimeoutException
that would be perfect for this case, why not use it?
12.
We have several options what to do when timeout happens:
- Throw
TimeoutException
instead ofTaskCanceledException
.- Pro: Easy to distinguish timeout from explicit cancellation action at runtime
- Con: Technical breaking change - new type of exception is thrown.
- Throw
TaskCanceledException
with inner exception asTimeoutException
- Pro: Possible to distinguish timeout from explicit cancellation action at runtime. Compatible exception type.
- Open question: Is it ok to throw away original
TaskCanceledException
stack and throw a new one, while preservingInnerException
(asTimeoutException.InnerException
)? Or should we preserve and just wrap the originalTaskCanceledException
?- Either: new TaskCanceledException -> new TimeoutException -> original TaskCanceledException (with original InnerException which may be null)
- Or: new TaskCanceledException -> new TimeoutException -> original TaskCanceledException.InnerException (may be null)
- Throw
TaskCanceledException
with message mentioning timeout as the reason- Pro: Possible to distinguish timeout from explicit cancellation action from the message / logs
- Con: Cannot be distinguished at runtime, it is "debug only".
- Open question: Same as in [2] - should we wrap or replace the original TaskCanceledException (and it stack)
I am leaning towards option [2], with discarding original stack of original TaskCanceledException
.
@stephentoub @davidsh any thoughts?
BTW: The change should be fairly straightforward in HttpClient.SendAsync
where we set up the timeout:
CancellationTokenSource cts; bool disposeCts; bool hasTimeout = _timeout != s_infiniteTimeout; if (hasTimeout || cancellationToken.CanBeCanceled) { disposeCts = true; cts = CancellationTokenSource.CreateLinkedTokenSource(cancellationToken, _pendingRequestsCts.Token); if (hasTimeout) { cts.CancelAfter(_timeout); } } else { disposeCts = false; cts = _pendingRequestsCts; } // Initiate the send. Task<HttpResponseMessage> sendTask; try { sendTask = base.SendAsync(request, cts.Token); } catch { HandleFinishSendAsyncCleanup(cts, disposeCts); throw; }
.NET Framework also throws TaskCanceledException when you set HttpClient.Timeout
.