本文是谭浩强老师c++程序设计(第三版)第八章的习题总结,主要涉及类的基础知识。
1. 请检查下面程序,找出其中的错误(先不要上机,在纸面上作人工检查),并改正之。然后上机调试,使之能正常运行。运行时从键盘输入时、分、秒的值,检查输出是否正确。
原程序:
#include <iostream>
using namespace std;
class Time
{ void set_time(void);
void show_time(void);
int hour;
int minute;
int sec;
};
Time t;
int main( )
{
set_time( );
show_time( );
return 0;
}
int set_time(void)
{
cin>>t.hour;
cin>>t.minute;
cin>>t.sec;
}
int show_time(void)
{
cout<<t.hour<<″:″<<t.minute<<″:″<<t.sec<<endl;
}
主要错误有:
(1) 类定义时没有声明函数和变量是public的,使类之外不可访问类的成员;
(2)类的成员函数定义时应用::指明函数作用域。
因此改法不唯一,此处修改第一种是将函数定义在类外,另一种是类中无函数,直接把函数作为全局函数,两种代码分别如下:
//设置时间程序
#include <iostream>
using namespace std;
class Time
{ public:
void set_time(void);
void show_time(void);
int hour;
int minute;
int sec;
};
Time t;
int main( )
{
t.set_time();
t.show_time( );
return 0;
}
void Time::set_time(void)
{
cin>>t.hour;
cin>>t.minute;
cin>>t.sec;
}
void Time::show_time(void)
{
cout<<t.hour<<" :"<<t.minute<<" :"<<t.sec<<endl;
}
#include <iostream>
using namespace std;
class Time
{public: //成员改为公用的
int hour;
int minute;
int sec;
};
Time t;
void set_time(void) //在main函数之前定义
{
cin>>t.hour;
cin>>t.minute;
cin>>t.sec;
}
void show_time(void) //在main函数之前定义
{
cout<<t.hour<<":"<<t.minute<<":"<<t.sec<<endl;
}
int main()
{set_time();
show_time();
return 0;
}
2. 改写例8.1程序,要求:
(1) 将数据成员改为私有的
(2) 将输入和输出的功能改为由成员函数实现;
(3) 在类体内定义成员函数。
//2. 改写例8.1程序,要求:
//(1) 将数据成员改为私有的
//(2) 将输入和输出的功能改为由成员函数实现;
//(3) 在类体内定义成员函数。
#include <iostream>
using namespace std;
class Time
{ public:
void set_time(void)
{
cin>>hour;
cin>>minute;
cin>>sec;
}
void show_time(void)
{
cout<<hour<<" :"<<minute<<" :"<<sec<<endl;
}
private:
int hour;
int minute;
int sec;
};
Time t;
int main( )
{
t.set_time();
t.show_time();
return 0;
}
3. 在第2题的基础上进行如下修改: 在类体内声明成员函数,而在类外定义成员函数。
这个函数同第一题的方法一,不再赘述。
4. 在第8.3.3节中分别给出了包含类定义的头文件student.h,包含成员函数定义的源文件student.cpp以及包含主函数的源文件main.cpp。请完善该程序,在类中增加一个对数据成员赋初值的成员函数set_value。上机调试并运行。
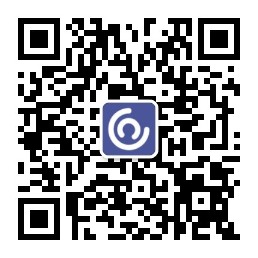
以下将依次写出所需的三个代码文件:
(1)头文件:(类的定义)
//头文件代码.h
//xt8-4.h(student.h)
class Student
{
public:
void display( );
void set_value();
private:
int num;
char name[20];
char sex ;
};
(2)成员函数定义:
//成员函数定义,student.cpp
//xt8-4-1.cpp(student.cpp)
#include <iostream>
using namespace std;
#include "8_4.h"
Student stu;
void Student::set_value(void)
{
cin>>stu.num;
cin>>stu.name;
cin>>stu.sex;
}
void Student::display(void)
{
cout<<"num:"<<stu.num<<endl;
cout<<"name:"<<stu.name<<endl;
cout<<"sex:"<<stu.sex<<endl;
}
(3)主函数:
//主函数
//xt8-4-1.cpp(main.cpp)
#include <iostream>
using namespace std;
#include "8_4.h"
int main()
{Student stud;
stud.set_value();
stud.display();
return 0;
}
5. 将例8.4 改写为一个多文件的程序:
(1) 将类定义放在头文件arraymax.h中;
(2) 将成员函数定义放在源文件arraymax.cpp中;
(3) 主函数放在源文件file1.cpp中。 请写出完整的程序,上机调试并运行。
就把第四题的三个代码放到不同文件即可。
6. 需要求3个长方柱的体积,请编写一个基于对象的程序。数据成员包括length(长)、width(宽)、 height(高)。要求用成员函数实现以下功能:
(1) 由键盘分别输入3个长方柱的长、宽、高;
(2) 计算长方柱的体积;
(3) 输出3个长方柱的体积。
请编程序,上机调试并运行。
#include <iostream>
using namespace std;
class Box
{
public:
void get_value();
float volume();
void display();
private:
float length;
float width;
float height;
};
void Box::get_value()
{
cout << "input lenght,width,height: ";
cin >> length;
cin >> width;
cin >> height;
}
float Box::volume()
{ return(length*width*height);}
void Box::display()
{ cout<<volume()<<endl;}
int main()
{
Box box1,box2,box3;
box1.get_value();
cout<<"volmue of bax1 is ";
box1.display();
box2.get_value();
cout<<"volmue of bax2 is ";
box2.display();
box3.get_value();
cout<<"volmue of bax3 is ";
box3.display();
return 0;
}