Priority queues are a type of container adapters, specifically designed such that the first element of the queue is the greatest of all elements in the queue and elements are in non decreasing order(hence we can see that each element of the queue has a priority{fixed order}). 优先队列是一种容器,队列的第一个元素是最大的,元素的顺序是非减顺序。
Methods of priority queue are: 优先队列的内置函数。
- priority_queue::empty() in C++ STL– empty() function returns whether the queue is empty.
- priority_queue::size() in C++ STL– size() function returns the size of the queue.
- priority_queue::top() in C++ STL– Returns a reference to the top most element of the queue
- priority_queue::push() in C++ STL– push(g) function adds the element ‘g’ at the end of the queue.
- priority_queue::pop() in C++ STL– pop() function deletes the first element of the queue.
- priority_queue::swap() in C++ STL– This function is used to swap the contents of one priority queue with another priority queue of same type and size.
- priority_queue::emplace() in C++ STL – This function is used to insert a new element into the priority queue container, the new element is added to the top of the priority queue.
- priority_queue value_type in C++ STL– Represents the type of object stored as an element in a priority_queue. It acts as a synonym for the template parameter.
Below is the C++ program to show the functionality of Priority Queue:
#include <iostream>
#include <queue>
using namespace std;
void showpq(priority_queue <int> gq)
{
priority_queue <int> g = gq;
while (!g.empty())
{
cout << '\t' << g.top();
g.pop();
}
cout << '\n';
}
int main ()
{
priority_queue <int> gquiz;
gquiz.push(10);
gquiz.push(30);
gquiz.push(20);
gquiz.push(5);
gquiz.push(1);
cout << "The priority queue gquiz is : ";
showpq(gquiz);
cout << "\ngquiz.size() : " << gquiz.size();
cout << "\ngquiz.top() : " << gquiz.top();
cout << "\ngquiz.pop() : ";
gquiz.pop();
showpq(gquiz);
return 0;
}
Output:
The priority queue gquiz is : 30 20 10 5 1
gquiz.size() : 5
gquiz.top() : 30
gquiz.pop() : 20 10 5 1
priority_queue::empty() and priority_queue::size() in C++ STL
Priority queues are a type of container adaptors, specifically designed such that the first element of the queue is the greatest of all elements in the queue.
priority_queue::empty() 判断队列是否为空。
empty() function is used to check if the priority queue container is empty or not.
Syntax :
pqueuename.empty()
Parameters :
No parameters are passed
Returns :
True, if priority queue is empty,
False, Otherwise
Examples:
Input : pqueue = 3, 2, 1
pqueue.empty();
Output : False
Input : pqueue
pqueue.empty();
Output : True
Errors and Exceptions
1. Shows error if a parameter is passed
2. Shows no exception throw guarantee.
// CPP program to illustrate
// Implementation of empty() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
priority_queue<int> pqueue;
pqueue.push(1);
// Priority Queue becomes 1
if (pqueue.empty()) {
cout << "True";
}
else {
cout << "False";
}
return 0;
}
Output:
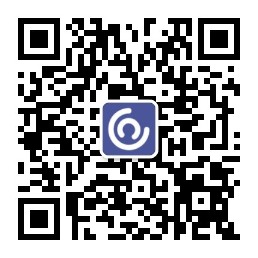
False
Application : Given a priority queue of integers, find the sum of the all the integers.
Input : 8, 6, 3, 2, 1
Output : 20
Algorithm
1. Check if the priority queue is empty, if not add the top element to a variable initialised as 0, and pop the top element.
2. Repeat this step until the priority queue is empty.
3. Print the final value of the variable.
// CPP program to illustrate
// Application of empty() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
int sum = 0;
priority_queue<int> pqueue;
pqueue.push(8);
pqueue.push(6);
pqueue.push(3);
pqueue.push(2);
pqueue.push(1);
// Queue becomes 8, 6, 3, 2, 1
while (!pqueue.empty()) {
sum = sum + pqueue.top();
pqueue.pop();
}
cout << sum;
return 0;
}
Output:
20
priority_queue::size() 返回队列的元素的个数。
size() function is used to return the size of the priority queue container or the number of elements in the container.
Syntax :
pqueuename.size()
Parameters :
No parameters are passed
Returns :
Number of elements in the container
Examples:
Input : pqueue = 3, 2, 1
pqueue.size();
Output : 3
Input : pqueue
pqueue.size();
Output : 0
Errors and Exceptions
1. Shows error if a parameter is passed.
2. Shows no exception throw guarantee
// CPP program to illustrate
// Implementation of size() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
int sum = 0;
priority_queue<int> pqueue;
pqueue.push(8);
pqueue.push(6);
pqueue.push(3);
pqueue.push(3);
pqueue.push(1);
// Priority Queue becomes 8, 6, 3, 2, 1
cout << pqueue.size();
return 0;
}
Output:
5
Application : Given a priority queue of integers, find the sum of the all the integers.
Input : 8, 6, 3, 2, 1
Output : 20
Algorithm
1. Check if the size of the priority queue is 0, if not add the top element to a variable initialised as 0, and pop the top element.
2. Repeat this step until the sizeof the priority queue becomes 0.
3. Print the final value of the variable.
// CPP program to illustrate
// Application of size() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
int sum = 0;
priority_queue<int> pqueue;
pqueue.push(8);
pqueue.push(6);
pqueue.push(3);
pqueue.push(2);
pqueue.push(1);
// Queue becomes 8, 6, 3, 2, 1
while (pqueue.size() > 0) {
sum = sum + pqueue.top();
pqueue.pop();
}
cout << sum;
return 0;
}
Output:
20
priority_queue::top() in C++ STL
Priority queues are a type of container adaptors, specifically designed such that the first element of the queue is the greatest of all elements in the queue.
priority_queue::top()
top() function is used to reference the top(or the largest) element of the priority queue. 获得队列的第一个元素,就是最大的元素。
Syntax :
pqueuename.top()
Parameters :
No value is needed to pass as the parameter.
Returns :
Direct reference to the top(or the largest)
element of the priority queue container.
Examples:
Input : pqueue.push(5);
pqueue.push(1);
pqueue.top();
Output : 5
Input : pqueue.push(5);
pqueue.push(1);
pqueue.push(7);
pqueue.top();
Output : 7
Errors and Exceptions
1. If the priority queue container is empty, it causes undefined behaviour
2. It has a no exception throw guarantee if the priority queue is not empty
// CPP program to illustrate
// Implementation of top() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
priority_queue<int> pqueue;
pqueue.push(5);
pqueue.push(1);
pqueue.push(7);
// Priority queue top
cout << pqueue.top();
return 0;
}
Output:
7
Application :
Given a priority queue of integers, find the number of prime and non prime numbers.
Input : 8, 6, 3, 2, 1
Output: Prime - 2
Non Prime - 3
Algorithm
1. Enter the size of the priority queue into a variable.
2. Check if the priority queue is empty, check if the top element is prime, if prime increments the prime counter, and pop the top element.
3. Repeat this step until the priority queue is empty.
4. Print the final value of the variable prime and nonprime(size – prime).
// CPP program to illustrate
// Application of top() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
int prime = 0, nonprime = 0, size;
priority_queue<int> pqueue;
pqueue.push(1);
pqueue.push(8);
pqueue.push(3);
pqueue.push(6);
pqueue.push(2);
size = pqueue.size();
// Priority queue becomes 1, 8, 3, 6, 2
while (!pqueue.empty()) {
for (int i = 2; i <= pqueue.top() / 2; ++i) {
if (pqueue.top() % i == 0) {
prime++;
break;
}
}
pqueue.pop();
}
cout << "Prime - " << prime << endl;
cout << "Non Prime - " << size - prime;
return 0;
}
Output:
Prime - 2
Non Prime - 3
priority_queue::push() and priority_queue::pop() in C++ STL
Priority queues are a type of container adaptors, specifically designed such that the first element of the queue is the greatest of all elements in the queue.
priority_queue::push()
push() function is used to insert an element in the priority queue. The element is added to the priority queue container and the size of the queue is increased by 1. Firstly, the element is added at the back and at the same time the elements of the priority queue reorder themselves according to priority. 在优先队列里插入元素,队列元素的个数加1,首先元素加在队列的尾部,同时优先队列重新排序。
Syntax :
pqueuename.push(value)
Parameters :
The value of the element to be inserted is passed as the parameter.
Result :
Adds an element of value same as that of
the parameter passed in the priority queue.
Examples:
Input : pqueue
myqueue.push(6);
Output : 6
Input : pqueue = 5, 2, 1
pqueue.push(3);
Output : 5, 3, 2, 1
Errors and Exceptions
1. Shows error if the value passed doesn’t match the priority queue type.
2. Shows no exception throw guarantee if the parameter doesn’t throw any exception.
// CPP program to illustrate
// Implementation of push() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
// Empty Queue
priority_queue<int> pqueue;
pqueue.push(3);
pqueue.push(5);
pqueue.push(1);
pqueue.push(2);
// Priority queue becomes 5, 3, 2, 1
// Printing content of queue
while (!pqueue.empty()) {
cout << ' ' << pqueue.top();
pqueue.pop();
}
}
Output:
5 3 2 1
priority_queue::pop()
pop() function is used to remove the top element of the priority queue.
Syntax :
pqueuename.pop()
Parameters :
No parameters are passed.
Result :
The top element of the priority
queue is removed.
Examples:
Input : pqueue = 3, 2, 1
myqueue.pop();
Output : 2, 1
Input : pqueue = 5, 3, 2, 1
pqueue.pop();
Output : 3, 2, 1
Errors and Exceptions
1. Shows error if a parameter is passed.
2. Shows no exception throw guarantee if the parameter doesn’t throw any exception.
// CPP program to illustrate
// Implementation of pop() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
// Empty Priority Queue
priority_queue<int> pqueue;
pqueue.push(0);
pqueue.push(1);
pqueue.push(2);
// queue becomes 2, 1, 0
pqueue.pop();
pqueue.pop();
// queue becomes 0
// Printing content of priority queue
while (!pqueue.empty()) {
cout << ' ' << pqueue.top();
pqueue.pop();
}
}
Output:
0
Application : push() and pop()
Given a number of integers, add them to the priority queue and find the size of the priority queue without using size function.
Input : 5, 13, 0, 9, 4
Output: 5
Algorithm
1. Push the given elements to the priority queue container one by one.
2. Keep popping the elements of priority queue until it becomes empty, and increment the counter variable.
3. Print the counter variable.
// CPP program to illustrate
// Application of push() and pop() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
int c = 0;
// Empty Priority Queue
priority_queue<int> pqueue;
pqueue.push(5);
pqueue.push(13);
pqueue.push(0);
pqueue.push(9);
pqueue.push(4);
// Priority queue becomes 13, 9, 5, 4, 0
// Counting number of elements in queue
while (!pqueue.empty()) {
pqueue.pop();
c++;
}
cout << c;
}
Output:
5
priority_queue::swap() in C++ STL
Priority queues are a type of container adaptors, specifically designed such that the first element of the queue is the greatest of all elements in the queue.
priority_queue::swap()
This function is used to swap the contents of one priority queue with another priority queue of same type and size.交换相同大小和类型的两个优先队列。
Syntax :
priorityqueuename1.swap(priorityqueuename2)
Parameters :
The name of the priority queue with which
the contents have to be swapped.
Result :
All the elements of the 2 priority queues are swapped.
Examples:
Input : mypqueue1 = {1, 2, 3, 4}
mypqueue2 = {3, 5, 7, 9}
mypqueue1.swap(mypqueue2);
Output : mypqueue1 = {9, 7, 5, 3}
mypqueue2 = {4, 3, 2, 1}
Input : mypqueue1 = {1, 3, 5, 7}
mypqueue2 = {2, 4, 6, 8}
mypqueue1.swap(mypqueue2);
Output : mypqueue1 = {8, 6, 4, 2}
mypqueue2 = {7, 5, 3, 1}
Note: In priority_queue container, the elements are printed
in reverse order because the top is printed first
then moving on to other elements.
Errors and Exceptions
1. It throws an error if the priority queues are not of the same type.
2. It throws an error if the priority queues are not of the same size.
2. It has a basic no exception throw guarantee otherwise.
// CPP program to illustrate
// Implementation of swap() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
// priority_queue container declaration
priority_queue<int> mypqueue1;
priority_queue<int> mypqueue2;
// pushing elements into the 1st priority queue
mypqueue1.push(1);
mypqueue1.push(2);
mypqueue1.push(3);
mypqueue1.push(4);
// pushing elements into the 2nd priority queue
mypqueue2.push(3);
mypqueue2.push(5);
mypqueue2.push(7);
mypqueue2.push(9);
// using swap() function to swap elements of priority queues
mypqueue1.swap(mypqueue2);
// printing the first priority queue
cout << "mypqueue1 = ";
while (!mypqueue1.empty()) {
cout << mypqueue1.top() << " ";
mypqueue1.pop();
}
// printing the second priority queue
cout << endl
<< "mypqueue2 = ";
while (!mypqueue2.empty()) {
cout << mypqueue2.top() << " ";
mypqueue2.pop();
}
return 0;
}
Output:
mypqueue1 = 9 7 5 3
mypqueue2 = 4 3 2 1
priority_queue::emplace() in C++ STL
Priority queues are a type of container adaptors, specifically designed such that the first element of the queue is the greatest of all elements in the queue.
priority_queue::emplace()
This function is used to insert a new element into the priority queue container, the new element is added to the top of the priority queue.
Syntax :
priorityqueuename.emplace(value)
Parameters :
The element to be inserted into the priority
queue is passed as the parameter.
Result :
The parameter is added to the
priority queue at the top position.
Examples:
Input : mypqueue{1, 2, 3, 4, 5};
mypqueue.emplace(6);
Output : mypqueue = 6, 5, 4, 3, 2, 1
Input : mypqueue{};
mypqueue.emplace(4);
Output : mypqueue = 4
Note: In priority_queue container, the elements are printed in reverse order because the top is printed first then moving on to other elements.
Errors and Exceptions
1. It has a strong exception guarantee, therefore, no changes are made if an exception is thrown.
2. Parameter should be of same type as that of the container, otherwise an error is thrown.
// INTEGER PRIORITY QUEUE
// CPP program to illustrate
// Implementation of emplace() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
priority_queue<int> mypqueue;
mypqueue.emplace(1);
mypqueue.emplace(2);
mypqueue.emplace(3);
mypqueue.emplace(4);
mypqueue.emplace(5);
mypqueue.emplace(6);
// queue becomes 1, 2, 3, 4, 5, 6
// printing the priority queue
cout << "mypqueue = ";
while (!mypqueue.empty()) {
cout << mypqueue.top() << " ";
mypqueue.pop();
}
return 0;
}
Output:
6 5 4 3 2 1
// CHARACTER PRIORITY QUEUE
// CPP program to illustrate
// Implementation of emplace() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
priority_queue<char> mypqueue;
mypqueue.emplace('A');
mypqueue.emplace('b');
mypqueue.emplace('C');
mypqueue.emplace('d');
mypqueue.emplace('E');
mypqueue.emplace('f');
// queue becomes A, b, C, d, E, f
// printing the priority queue
cout << "mypqueue = ";
while (!mypqueue.empty()) {
cout << mypqueue.top() << " ";
mypqueue.pop();
}
return 0;
}
Output:
f E d C b A
// STRING PRIORITY QUEUE
// CPP program to illustrate
// Implementation of emplace() function
#include <iostream>
#include <queue>
#include <string>
using namespace std;
int main()
{
priority_queue<string> mypqueue;
mypqueue.emplace("portal");
mypqueue.emplace("computer science");
mypqueue.emplace("is a");
mypqueue.emplace("GEEKSFORGEEKS");
// queue becomes portal, computer scince,
// is a, GEEKSFORGEEKS
// printing the priority queue
cout << "mypqueue = ";
while (!mypqueue.empty()) {
cout << mypqueue.top() << " ";
mypqueue.pop();
}
return 0;
}
Output:
GEEKSFORGEEKS is a computer science portal
Application :
Given a number of integers, add them to the priority queue using emplace() and find the size of the priority queue.
Input : 5, 13, 0, 9, 4
Output: 5
Algorithm
1. Insert the given elements to the priority queue container one by one using emplace().
2. Keep popping the elements of priority queue until it becomes empty, and increment the counter variable.
3. Print the counter variable.
// CPP program to illustrate
// Application of emplace() function
#include <iostream>
#include <queue>
using namespace std;
int main()
{
int c = 0;
// Empty Priority Queue
priority_queue<int> pqueue;
// inserting elements into priority_queue
pqueue.emplace(5);
pqueue.emplace(13);
pqueue.emplace(0);
pqueue.emplace(9);
pqueue.emplace(4);
// Priority queue becomes 13, 9, 5, 4, 0
// Counting number of elements in queue
while (!pqueue.empty()) {
pqueue.pop();
c++;
}
cout << c;
}
Output:
5
Time Complexity : O(1)
emplace() vs push()
When we use push(), we create an object and then insert it into the priority_queue. With emplace(), the object is constructed in-place and saves an unnecessary copy. Please see emplace vs insert in C++ STL for details. push是创建一个对象,然后插入到优先队列,emplace是直接在该位置创建,避免复制。
// C++ code to demonstrate difference between
// emplace and insert
#include<bits/stdc++.h>
using namespace std;
int main()
{
// declaring priority queue
priority_queue<pair<char, int>> pqueue;
// using emplace() to insert pair in-place
pqueue.emplace('a', 24);
// Below line would not compile
// pqueue.push('b', 25);
// using push() to insert pair
pqueue.push(make_pair('b', 25));
// printing the priority_queue
while (!pqueue.empty()) {
pair<char, int> p = pqueue.top();
cout << p.first << " "
<< p.second << endl;
pqueue.pop();
}
return 0;
}
Output :
b 25
a 24