一、概述
在用户模块,对于用户密码的保护,通常都会进行加密。我们通常对密码进行加密,然后存放在数据库中,在用户进行登录的时候,将其输入的密码进行加密然后与数据库中存放的密文进行比较,以验证用户密码是否正确。
目前,MD5和BCrypt比较流行。相对来说,BCrypt比MD5更安全,但加密更慢。
二、使用BCrypt
首先,可以在官网中取得源代码:http://www.mindrot.org/projects/jBCrypt/
然后通过Ant进行编译。编译之后得到jbcrypt.jar。也可以不需要进行编译,而直接使用源码中的BCrypt.java文件(本身仅一个文件)。
下面是官网的一个Demo。
public class TestUtil {
public static void main(String[] args) {
String password = "testPassword";
// Hash a password for the first time
String hashpw = BCrypt.hashpw(password, BCrypt.gensalt());
// gensalt's log_rounds parameter determines the complexity
// the work factor is 2**log_rounds, and the default is 10
String hashpw2 = BCrypt.hashpw(password, BCrypt.gensalt(12));
System.out.println("salt:" + BCrypt.gensalt());
System.out.println("salt2:" + BCrypt.gensalt(12));
System.out.println("hashpw:" + hashpw);
System.out.println("hashpw2:" + hashpw2);
String inputStr = "testPassword";
// Check that an unencrypted password matches one that has
// previously been hashed
if (BCrypt.checkpw(inputStr, hashpw2)) {
System.out.println("It matches");
}else {
System.out.println("It does not match");
}
}
}
输出:
salt:$2y$10$JOQKt1QIX7bzmNDy243ecO
salt:$2y$10$9lQoSayj8x4w9YiAZ89WWO
salt2:$2y$15$lQLZ.V5OZghFwNY7xgNbdO
hashpw:$2y$10$.7MmgjfztxerjqQcm1WFteAnU8ZMVlzHwH0mMqLpIKN3xfOpHyOS6
hashpw2:$2y$12$VC0sy8WXO5oGLl9S/P6ekOQSRJ2nduwUSAP1rtJKh4fila3jgRUyq
It matches
可以看到,在对密码进行加密和验证的时候是很方便的。
在这个例子中:
BCrypt.hashpw(password, BCrypt.gensalt())
是核心。通过调用BCrypt类的静态方法hashpw对password进行加密。第二个参数就是我们平时所说的加盐,gensalt()
里面的参数可要可不要,默认是10,当然我们可以自己随意设定一个大于0小于30的值。
这里可以看一下源码:
gensalt
方法,有一个无参的和一个有参的方法,无参的方法里的默认值是10,
/**
* Generate a salt for use with the BCrypt.hashpw() method
* @param log_rounds the log2 of the number of rounds of
* hashing to apply - the work factor therefore increases as
* 2**log_rounds.
* @return an encoded salt value
*/
public static String gensalt(int log_rounds) {
return gensalt(log_rounds, new SecureRandom());
}
/**
* Generate a salt for use with the BCrypt.hashpw() method,
* selecting a reasonable default for the number of hashing
* rounds to apply
* @return an encoded salt value
*/
public static String gensalt() {
return gensalt(GENSALT_DEFAULT_LOG2_ROUNDS);
}
/**
* Generate a salt for use with the BCrypt.hashpw() method
* @param log_rounds the log2 of the number of rounds of
* hashing to apply - the work factor therefore increases as
* 2**log_rounds.
* @param random an instance of SecureRandom to use
* @return an encoded salt value
*/
public static String gensalt(int log_rounds, SecureRandom random) {
StringBuffer rs = new StringBuffer();
byte rnd[] = new byte[BCRYPT_SALT_LEN];
random.nextBytes(rnd);
rs.append("$2y$");
if (log_rounds < 10)
rs.append("0");
if (log_rounds > 30) {
throw new IllegalArgumentException(
"log_rounds exceeds maximum (30)");
}
rs.append(Integer.toString(log_rounds));
rs.append("$");
rs.append(encode_base64(rnd, rnd.length));
return rs.toString();
}
通过查看源码,可以看到,加密的密码的前几位是固定的,包含了$2y$
和我们输入的加密次数,后面的部分是随机的。
然后我们再来看一下验证密码的方法:
BCrypt.checkpw(candidate, hashed)
该方法就是对用户后来输入的密码进行比较。如果能够匹配,返回true。
三、加盐
如果两个人或多个人的密码相同,加密后保存会得到相同的结果。破一个就可以破一片的密码。如果名为A的用户可以查看数据库,那么他可以观察到自己的密码和别人的密码加密后的结果都是一样,那么,别人用的和自己就是同一个密码,这样,就可以利用别人的身份登录了。
其实只要稍微混淆一下就能防范住了,这在加密术语中称为“加盐”。具体来说就是在原有材料(用户自定义密码)中加入其它成分(一般是用户自有且不变的因素),以此来增加系统复杂度。当这种盐和用户密码相结合后,再通过摘要处理,就能得到隐蔽性更强的摘要值。
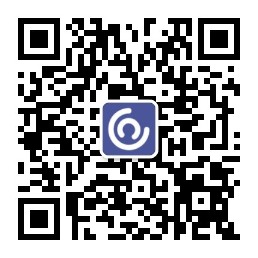
而使用BCrypt工具,就可以帮我们解决盐的随机性问题,从输出结果可以看出,即使获取盐时BCrypt.gensalt()
都是使用的默认值,但是生成的盐还是不一样的。
使用这个工具,就可以解决一行代码加密,一行代码验证密码是否正确。