在编写jsp时,不需要声明(创建)就可以直接使用的对象称为隐式对象
在jsp中,有九大隐式对象:
request | HttpServletRequest | 请求信息 |
response | HttpServletResponse | 响应信息 |
PageContext | PageContext | 显示此页面的上下文 |
session | HttpSession | 表示会话对象 |
out | JspWriter | 输出值 |
config | ServletConfig | jsp的配置信息 |
page | HttpJspPage | 如同Java中的this |
application | ServletContext | 表示此应用程序的上下文 |
exception | Throwable | 异常处理 |
(1)用来数据共享的对象:
pageContext:在本页共享数据
request:在同一次请求响应过程中共享数据
session:在同一个会话中共享数据(从浏览器到浏览器关闭之间默认为一次对话,如果浏览器关闭,session对象从浏览器内存中被清除)
application:在应用程序运行期间共享数据(服务器打开到服务器关闭之间默认为一次应用)
代码示例:
Index.jsp:
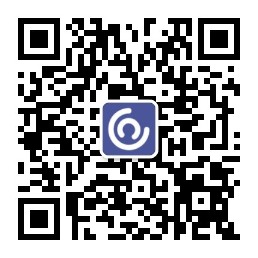
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title></head> <body><h2>这是index.jsp页面</h2> <% pageContext.setAttribute("pageContext", 1); request.setAttribute("request", 1); session.setAttribute("session", 1); application.setAttribute("application", 1); Object obj1=pageContext.getAttribute("pageContext"); Object obj2=request.getAttribute("request"); Object obj3=session.getAttribute("session"); Object obj4=application.getAttribute("application"); %> pageContext:<%=obj1 %><br/>; request:<%=obj2 %><br/>; session:<%=obj3 %><br/>; application:<%=obj4 %><br/>; </body> </html>
运行结果:
这是index.jsp页面
pageContext:1
; request:1
; session:1
; application:1
在index.jsp中加入如下代码:
<% request.getRequestDispatcher("index2.jsp").include(request,response); response.sendRedirect("index2.jsp");
index2.jsp代码:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <h2>这是index2.jsp页面</h2> <% //在转发的页面获取 Object obj1=pageContext.getAttribute("pageContext"); Object obj2=request.getAttribute("request"); Object obj3=session.getAttribute("session"); Object obj4=application.getAttribute("application"); %> pageContext:<%=obj1 %><br/> request:<%=obj2 %><br/> session:<%=obj3 %><br/> application:<%=obj4 %><br/> </body> </html>
这是在index.jsp中加入:request.getRequestDispatcher(“index2.jsp”).include(request,response)后运行结果:
这是index2.jsp页面
pageContext:null
request:1
session:1
application:1
这是index.jsp页面
pageContext:1
; request:1
; session:1
; application:1
这是在index.jsp中加入:response.sendRedirect(“index2.jsp”)后运行结果:
这是index2.jsp页面
pageContext:nullrequest:null
session:1
application:1
以上运行结果分析:
1,由于pageContext的作用域在本页面,所以只有在index.jsp本页面中其值为1,而在index2.jsp中,其值为空;
2,对于request,在index.jsp中加入include那行代码的时候,浏览器还是为同一个请求,所以request的值还是为1;而sendRedirect为重定向,即浏览器向服务器发出请求,服务器不是直接向浏览器返回index2.jsp这个页面(如果是的话,即为同一请求),而是让浏览器直接请求index2.jsp,所以此时不是同一个request,所以此时浏览器跳转到index2.jsp页面的时候,request为null;
3,对于session,只要是同一个浏览器,一个窗口或者多个窗口,均看做同一个会话,只有浏览器关闭,session对象从浏览器内存中被清空,session的值才为空;
例如关闭浏览器窗口,运行index2.jsp结果如下:
这是index2.jsp页面
pageContext:null
request:null
session:null
application:1
4,对于application,只要服务器未关闭,均为同一个应用,只有浏览器重启,application的值才会清空,如关闭服务器重启,再运行index2.jsp结果如下:
这是index2.jsp页面
pageContext:null
request:null
session:null
application:null
(2),与输入输出有关的对象:
out:向浏览器输出信息
request:包含请求信息
response:包含的响应信息
(3),和Servlet有关的对象:
page:指jsp页面本身
代码示例:
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <%//由于JSP本身就是一个Servlet HttpJspPage servlet = (HttpJspPage) page; String str = servlet.getServletInfo(); String str2=this.getServletInfo(); String str3 = this.getServletName(); %> <%=str%><br/> <%=str2%><br/> <%=str3%> </body> </html>
运行结果如下:
Jasper JSP 2.1 Engine
Jasper JSP 2.1 Engine
jsp
config:用来存储jsp配置信息的对象
index5.jsp代码如下:
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 这个是index5.jsp! <br/> <% String className = config.getInitParameter("className"); String url = config.getInitParameter("url"); String user = config.getInitParameter("user"); String pwd = config.getInitParameter("pwd");%> <%=className %> <br/> <%=url %> <br/> <%=user %> <br/> <%=pwd %> <br/> </body> </html>
在web.xml中配置以下信息;
<servlet> <servlet-name>aa</servlet-name> <jsp-file>/index5.jsp</jsp-file> <init-param> <param-name>className</param-name> <param-value>oracle.jdbc.driver.OracleDriver</param-value> </init-param> <init-param> <param-name>url</param-name> <param-value>jdbc:oracle:thin:@127.0.0.1:1521:ORCL</param-value> </init-param> <init-param> <param-name>user</param-name> <param-value>scott</param-value> </init-param> <init-param> <param-name>pwd</param-name> <param-value>1234</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>aa</servlet-name> <url-pattern>/aa</url-pattern> </servlet-mapping>
运行index5.jsp结果如下:
这个是index5.jsp!
oracle.jdbc.driver.OracleDriver
jdbc:oracle:thin:@127.0.0.1:1521:ORCL
scott
1234
(4),和异常处理有关的对象
Exception:用来处理异常的对象
定义错误页面 <%@ page isErrorPage="true" %>
如果页面需要处理异常 <%@ page errorPage="error.jsp"%>
代码示例:
index6.jsp代码如下:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page errorPage="error.jsp"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% int a = 1, b = 0; %> <%=a / b%> <a href="index7.jsp">这是一个超链接</a> </body> </html>
error.jsp代码如下:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page isErrorPage="true" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 你访问的页面飞走了!! <% String msg = exception.getMessage(); %> <%=msg %> </body> </html>
index6.jsp程序中设置了出错处理页面为 <%@ page errorPage=”error.jsp”%>,所以index6.jsp页面在运行过程中一旦出现异常,就会自动跳到error.jsp页面。在error.jsp中利用<%@ page isErrorPage=”true”%>将其设置为错误页面,可以在该页面中处理捕捉到的异常;由于index6.jsp中进行了除0操作,即为错误页面,所以运行index6.jsp时,页面无法显示,而跳转到了处理异常页面的error.jsp页面
运行结果如下:
你访问的页面飞走了!! / by zero