原创转载请注明出处:http://agilestyle.iteye.com/blog/2361909
Question
Print all pairs of values a sorted array that sum up to a given value M
Example
Solution
1. Scan from both ends, calculate the sum
2. if(sum is equal to M), print and shrink the focus window
3. if(sum is less than M), shift left focus index by 1
4. if(sum is bigger than M), shift right focus index by 1
5. Stop the loop when left is larger or equal to right index
扫描二维码关注公众号,回复:
502184 查看本文章
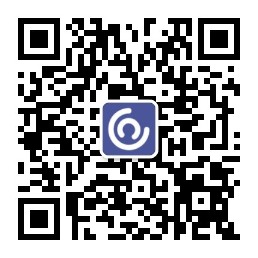
package org.fool.java.test; public class PrintAllPairsSumToM { public static void main(String[] args) { int[] sorted = {1, 2, 3, 4, 8, 9, 9, 10}; printAllPairs(sorted, 12); } private static void printAllPairs(int[] sorted, int m) { int left = 0; int right = sorted.length - 1; while (left < right) { int tempSum = sorted[left] + sorted[right]; if (tempSum == m) { // which means we find one pair System.out.println("Sum of {" + sorted[left] + ", " + sorted[right] + "} = " + m); // update the both index values left++; right--; } else if (tempSum > m) { // which means the temp sum is bigger than m right--; // shift right focus index by 1 } else { // which means the temp sum is smaller than m left++; // shift left focus index by 1 } } } }
Console Output
Reference
https://www.youtube.com/watch?v=l5orcTJlT54&list=PLlhDxqlV_-vkak9feCSrnjlrnzzzcopSG&index=39