1.效果展示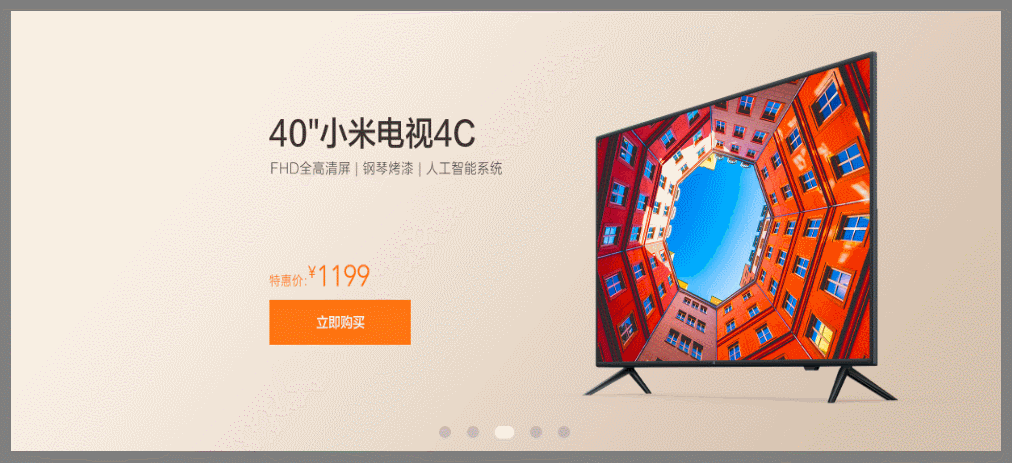
2.html代码
<div class="wrap">
<div class="carousel">
<!--左箭头-->
<div class="arrow arrow-l"><</div>
<!--右箭头-->
<div class="arrow arrow-r">></div>
<!--轮播图-->
<div class="imgs">
<img src="images/xiaomi4.jpg" alt="" class="active">
<img src="images/xiaomi5.jpg" alt="">
<img src="images/xiaomi6.jpg" alt="">
<img src="images/xiaomi7.jpg" alt="">
<img src="images/xiaomi8.jpg" alt="">
</div>
<!--圆点-->
<div class="circle">
<ul>
<li class="active"></li>
<li></li>
<li></li>
<li></li>
<li></li>
</ul>
</div>
</div>
</div>
3.css代码
/*CSS初始化*/
* {
margin: 0;
padding: 0;
}
body {
background-color: #808080;
}
ul {
list-style: none; /* 去除ul前面的小点 */
}
/*轮播图模块*/
.carousel {
width: 990px;
height: 440px;
margin: 100px auto;
/*border: 1px solid #ddd;*/
border-radius: 10px;
position: relative;
}
/*轮播图大小*/
.carousel img {
display: none;
width: 990px;
height: 440px;
cursor: pointer;
}
/*显示轮播图*/
img.active {
display: block;
}
/*轮播标识模块*/
.circle ul {
width: 150px;
height: 20px;
position: absolute;
bottom: 10px;
left: 50%;
margin-left: -75px;
}
/*轮播小点*/
li {
width: 12px;
height: 12px;
border-radius: 50%;
margin: 5px 8px;
float: left;
background: rgba(125, 125, 151, .3);
cursor: pointer;
}
/*激活小点*/
li.active {
width: 19px;
border-radius: 10px;
background-color: #fff;
}
/*箭头*/
.arrow {
position: absolute;
top: 50%;
margin-top: -30px;
z-index: 99;
display: none;
width: 30px;
height: 60px;
font-size: 30px;
font-weight: bold;
line-height: 60px;
text-align: center;
color: #fff;
background-color: rgba(0, 0, 0, .1);
cursor: pointer;
}
/*左箭头*/
.arrow-l {
left: 0;
}
/*右箭头*/
.arrow-r {
right: 0;
}
/*箭头悬浮*/
.arrow:hover {
background-color: rgba(0, 0, 0, .7);
}
4.JS代码(需要引入jquery)
$(function () {
// 定时器变量
var plays;
// 启动滚动定时器
function playRoll() {
plays = setInterval(function () {
var idx = $('li').index($('li.active')) + 1;
var n = $('li').length - 1;
if (idx > n) {
idx = 0;
}
$('li:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
$('img:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
}, 3000)
}
// 清除定时器
function stopRoll() {
clearInterval(plays);
}
//默认滚动
playRoll();
// 点击圆点
$('li').click(function () {
var idx = parseInt($('li').index(this));
$('li:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
$('img:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
})
// 进入轮播图区域,显示箭头,清除定时器
$('.carousel').stop().mouseenter(function () {
$('.arrow').fadeIn();
stopRoll();
})
// 离开轮播图区域,隐藏箭头,开启定时器
$('.carousel').stop().mouseleave(function () {
$('.arrow').fadeOut();
playRoll();
})
// 点击左箭头
$('.arrow-l').click(function () {
var n = $('li').length - 1;
var idx = $('li').index($('li.active')) - 1;
if (idx < 0) {
idx = n;
}
$('li:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
$('img:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
})
// 点击右箭头
$('.arrow-r').click(function () {
var idx = $('li').index($('li.active')) + 1;
var n = $('li').length - 1;
if (idx > n) {
idx = 0;
}
$('li:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
$('img:eq(' + idx + ')').addClass('active').siblings().removeClass('active');
})
})
5.实现原理
- 先让图片轮播起来。写一个 playRoll函数里面加定时器,获取当前显示图片的index,使图片的index加1,当index+1大于最大值时,设置index等于0.这样轮播图就动起来了。
- 轮播图动起来基本就是只显示一张图片隐藏其他的图片。使用display:none来隐藏图片,通过添加active类来显示图片 。
- 图片下面的按钮。主要就是使下面的按钮与上面的图片一一对应。然后点击下面的按钮显示对应的图片。
- 左右的上一张和下一张按钮。点击左边的上一张按钮图片向前显示,实现原理就是使 index - 1。点击左边的下一张按钮图片向后显示,实现原理就是使 index + 1,注意判断是否为第一张或者最后一张图片。
- 当鼠标放在轮播图区域时停止轮播,实现原理就是清除定时器,使用stopRoll()方法,离开开始轮播就是加上定时器,使用playRoll()方法。