interrupt()
源码:public void interrupt() {
if (this != Thread.currentThread())
checkAccess(); //判断此线程是否为当前线程,不等于,则进行检查,此处有可能抛出SecurityException异常。
synchronized (blockerLock) {
Interruptible b = blocker;
if (b != null) {
interrupt0(); // Just to set the interrupt flag只是设置中断标志
b.interrupt(this);
return;
}
}
interrupt0();
}
这个方法的作用:其作用是中断此线程(此线程不一定是当前线程,而是指调用该方法的Thread实例所代表的线程),但实际上只是给线程设置一个中断标志,线程仍会继续运行。
interrupt()并不会终止处于“运行状态”的线程!它会将线程的中断标记设为true
若线程在阻塞状态时,调用了它的interrupt()方法,那么它的“中断状态”会被清除并且会收到一个InterruptedException异常,
isInterrupted():
源码:public boolean isInterrupted() {
return isInterrupted(false);//这句话表示:1:检查调用该方法的线程是否被中断 2:不清除中断状态
}
interrupted();
源码:public static boolean interrupted() {
return currentThread().isInterrupted(true); //这句话表示1:检查当前线程是否被中断,2:清除中断状态
}
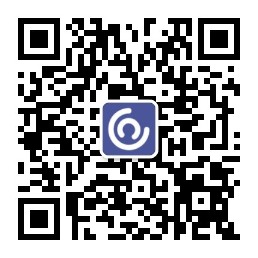
private native boolean isInterrupted(boolean ClearInterrupted);
ClearInterrupted:true代表清除中断状态 false 表示不清楚中断状态
终止处于“阻塞状态”的线程
我们通过“中断”方式interrupt()终止处于“阻塞状态”的线程。
为什么使用interrupt()终止处于阻塞状态的线程?:
1:首先阻塞状态是线程调用了sleep,join,wait方法,使线程进入阻塞或者等待
2:若线程在阻塞状态时,调用了它的interrupt()方法,那么它的“中断状态”会被清除并且会收到一个InterruptedException异常,
3:这样,我们如果捕获了InterruptedException异常, 并将InterruptedException放在适当的为止就能终止线程
终止处于“运行状态”的线程
我们通过“标记”方式isInterrupted()终止处于“运行状态”的线程。其中,包括“中断标记”和“额外添加标记”。
为什么可以使用isInterrupted()终止处于运行状态的线程,
1:isInterrupted()是判断线程的中断标记是不是为true
2:当线程处于运行状态,并且我们需要终止它时;可以调用线程的interrupt()方法,使用线程的中断标记为true,这个时候就做了个标记,
3:注意:interrupt()并不会终止处于“运行状态”的线程!它会将线程的中断标记设为true
4:我们在运行代码的时候
while (!isInterrupted()) {
// 执行任务...
}
//如果之前调用了interrupt()方法,这里的判断条件就为false,不会执行任务,这就做到了终止运行状态中的线程
综合线程处于“阻塞状态”和“运行状态”的终止方式,比较通用的终止线程的形式如下:
@Override
public void run() {
try {
// 1. isInterrupted()保证,只要中断标记为true就终止线程。
while (!isInterrupted()) {
// 执行任务...
}
} catch (InterruptedException ie) {
// 2. InterruptedException异常保证,当InterruptedException异常产生时,线程被终止。
}
}