一、ADO.NET简介
ADO.NET可以看作是C#语言访问数据库的一种方式。编程语言编写的程序需要数据库的支持,那么怎样才能让他们建立连接呢?当然是ADO.NET
二、ADO.NET 整体流程
1)写连接字符串
2)写连接对象
3)写sql语句
4)写操作SQL语句的对象SqlCommand
5)打开数据库
6)最后写执行操作对象的方法:ExecuteNonQuery(),executescalar(),ExecuteReader()
其中还有写小步骤,具体在案例里面显示。(第四步和第五步可以互换)
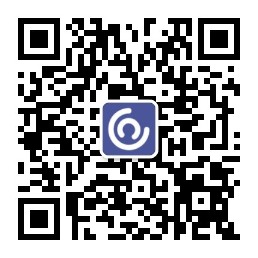
三、连接字符串
如果想了解详细的:https://www.cnblogs.com/shuibi/p/6566127.html
SQL SEVER
标准安全连接:
Data Source=.;Initial Catalog=myDataBase;User Id=myUsername;Password=myPassword;或者
Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;Trusted_Connection=False;
可信连接:
Data Source=192.168.0.4;Initial Catalog=MyDataBase;Integrated Security=True;或者
Server=ServerAddress;Database=MyDataBase;Trusted_Connection=True;
Access连接字符串
Provider=Microsoft.Jet.OLEDB.4.0;Data Source=C:\myDatabase.mdb;User Id=admin;Password=;
MySQL连接字符串
Server=myServerAddress;Database=myDatabase;Uid=myUsername;Pwd=myPassword;
DB2连接字符串
Server=myAddress:myPortNumber;Database=myDatabase;UID=myUsername;PWD=myPassword;
Oracle连接字符串
Data Source=TORCL;User Id=myUsername;Password=myPassword;
注意:如果你实在不知道怎么写,也可以通过VS来获取连接字符串
工具 >>>连接到数据库
提前准备好数据库,后面使用: --创建数据库 create database ExampleInfo --创建学生表 create table StudentTable( Sid int not null primary key identity, Sname varchar(50), Sage datetime, Ssex nvarchar(10) ) insert into StudentTable(Sname,Sage,Ssex) values ('王昭君',18,'女'),('貂蝉',18,'女'),('韩信',18,'男'), ('李白',20,'男'),('蔡文姬',19,'女'),('后裔',19,'男'),('伽罗',19,'女') --创建课程表 create table CourseTable( Cid int not null primary key identity, Cname varchar(50), Tid int ) insert into CourseTable(Cname,Tid) values ('语文',1),('数学',3),('英语',5), ('物理',4),('化学',6),('生物',5),('地理',2) --创建教师表 create table TeacherTable( Tid int not null primary key identity, Tname varchar(50) ) insert into TeacherTable(Tname) values ('诸葛亮'),('黄总'),('老夫子'),('墨子'),('女娲'),('伏羲') --创建一张学生课程表 create table SCTable( Sid int, Cid int, Score int, foreign Key (Sid) REFERENCES StudentTable(Sid), foreign Key (Cid) REFERENCES CourseTable(Cid) ) insert into SCTable (Sid,Cid,Score) values (1,1,80),(1,2,89),(1,3,88),(1,4,87),(1,5,78), (1,6,48),(1,7,87),(2,1,55),(2,2,77),(2,4,99),(2,5,89),(2,6,15),(3,1,88),(3,2,77),(3,3,78),(3,4,75),(3,5,67), (3,6,89),(3,7,88),(4,1,78),(4,4,98),(4,5,89),(4,6,78),(4,7,79),(5,1,77),(5,2,85),(5,3,82),(5,5,76), (5,6,95),(6,1,94),(6,4,48),(7,1,58),(7,2,88),(7,4,75), (7,6,84),(7,7,99)
四、连接对象Connection
主要是用于连接到数据库的
由于后面需要
Connection常用属性:
属性 | 说明 |
ConncetionString | 获取或设置用于打开数据库的字符串 |
ConnectioTimeout | 获取在尝试建立连接时终止尝试并生成错误之前所等待的时间 |
DataBase | 获取当前数据库,或者连接打开后要使用的数据名称 |
DataSource | 获取哟啊连接的数据库服务器的名称 |
State | 数据库连接状态 |
Connection常用方法:
方法 | 说明 |
Open | 打开数据库连接 |
Close | 关闭数据库连接 |
Dispose | 释放Connection使用的所有资源 |
这是一个使用模板 //编写连接字符串 String constr = "Data Source = .;Initial Catalog = ExampleInfo;Integrated Security = True" //创建连接对象 using(SqlConnection conn = new Sqlnnection(Constr)){ // 当在某个代码段中使用了类的实例,而希望无论因为什么原因,只要离开了这个代码段就自动调用这个类实例的Dispose
//要达到这样的目的,用try...catch来捕捉异常也是可以的,但用using也很方便。 //写Sql语句 //创建command对象 //打开数据 //执行 }
五、Command
Command有四种:SqlCommand,OleDbCommand,OdbcCommand,OracleComman
具体看使用的是什么数据库。
Command对象常用的属性
属性 | 说明 |
CommandType | 获取要执行命令的类型 |
CommandText | 获取或者设置要对数据源执行的SQL语句或存储过程或表名 |
Conncetion | 获取或设置这个Command使用的Connection对象的名称 |
Parameters | 获取Command对象需要使用的参数集合 |
Transaction | 获取或设置将在其中执行的SqlTransaction |
这是一个使用模板
//编写连接字符串 String constr = "Data Source = .;Initial Catalog = ExampleInfo;Integrated Security = True" //创建连接对象 using(SqlConnection conn = new Sqlnnection(Constr)){ //写Sql语句 string sql = "select * from StudentTable"; using(SqlCommand cmd = new Command(sql,conn)){ //打开数据 conn.Open(); //执行
.... } }
Command对象常用的方法:
(一)ExecuteNonQuery
用于执行非select语句,比如增删改,它会返回影响的行数
1.向数据库中的StudentTable表中插入数据
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.SqlClient; namespace commandExecuteNonQuery { class Program { static void Main(string[] args) { //创建连接字符串 string constr = "Data Source =.;Initial Catalog = ExampleInfo;Integrated Security = True"; //创建连接对象 using(SqlConnection conn = new SqlConnection(constr)){ //床架sql语句 string sql = "insert into StudentTable (Sname,Sage,Ssex) values ('露娜',16,'女')"; //创建command对象 using (SqlCommand cmd = new SqlCommand(sql, conn)) { //打开数据库 conn.Open(); //执行数据语句的command方法 int x = cmd.ExecuteNonQuery(); //返回的是受影响的行数 if (x > 0) { Console.WriteLine("插入成功"); }else{ Console.WriteLine("插入失败"); } } } } } }
(二)ExecuteScalar
通常用来执行SELECT查询命令,返回第一行第一列值,所以都是用来执行带有Count() 或者是Sum()函数的数据库SQL语句
1.返回数据库表StudentTable的记录条数
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.SqlClient; namespace commandExecuteNonQuery { class Program { static void Main(string[] args) { //创建连接字符串 string constr = "Data Source =.;Initial Catalog = ExampleInfo;Integrated Security = True"; //创建连接对象 using (SqlConnection conn = new SqlConnection(constr)) { //床架sql语句 string sql = "select Count(*) from StudentTable"; //创建command对象 using (SqlCommand cmd = new SqlCommand(sql, conn)) { //打开数据库 conn.Open(); //执行数据语句的command方法 object x = cmd.ExecuteScalar(); Console.WriteLine(x); } } } } }
(三)ExecuteReader
由于ExecuteReader通常是和DataReader一起使用的,所以我就放到DataReader一起说
五、DataReader
是一个简单的数据集
DataReader对象的常用属性:
属性 | 说明 |
Connection | 获取与DataReader关联的Conncetion对象 |
HasRows | 判断数据库中是否有数据 |
FieldCount | 获取当前行的列数 |
IsClosed | 是否已关闭DataReader实例,是一个bool值 |
Item | 获取指定列的以本机的格式表示的值 |
DataReader对象的常用方法:
方法 | 说明 |
ISDBNull | 获取一个值,判断是否是空值 |
Read | 使DataReader对象指向下一条记录 |
NextResult | 使数据读取器前进到下一个结果 |
Close | 关闭DataReader |
Get | 用来读取数据集的当前行的某一列的数据 |
这是一个使用模板
//连接字符串 string constr = "data source =.;Initial catalog = StudentInfo;Integrated Security = True"; //创建连接对象 using (SqlConnection conn = new SqlConnection(str2)) { //sql语句 string sql = "select * from StudentTable"; //创建Command对象 using (SqlCommand comm = new SqlCommand(sql, conn)) {//打开数据库 conn.Open(); //创建DataReader对象 using (SqlDataReader reader = comm.ExecuteReader()) { if (reader.HasRows) //如果有数据,一条一条去读数据 { //reader.Read() 向后移动一条数据(因为默认指向-1,就是开始时候不指向任何数据),有数据就会返回true while (reader.Read()) { .......... } } } } }
//小案例:查询返回表StudentTable using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.SqlClient; namespace commandExecuteNonQuery { class Program { static void Main(string[] args) { //创建连接字符串 string constr = "Data Source =.;Initial Catalog = ExampleInfo;Integrated Security = True"; //创建连接对象 using (SqlConnection conn = new SqlConnection(constr)) { //床架sql语句 string sql = "select * from StudentTable"; //创建command对象 using (SqlCommand cmd = new SqlCommand(sql, conn)) { //打开数据库 conn.Open(); //执行数据语句ExecuteReader()的方法 SqlDataReader reader = cmd.ExecuteReader(); if (reader.HasRows) { while (reader.Read()) {
Console.Write(reader.GetInt32(0) + " ");
Console.Write(reader.GetString(1) + " ");
Console.Write(reader.GetInt32(2) + " ");
Console.Write(reader.GetString(3) + " ");
Console.WriteLine();
} } } } } } }
六、DataAdapter
DataAdapter表示一组 SQL 命令和一个数据库连接,它们用于填充 DataSet和更新数据源
DataAdapter的属性:
属性 | 说明 |
SelectCommand | 引用从数据源中检索行的Command对象 |
InsertCommand | 引用将插入的行从DataSet写入数据源的Command对象 |
UpdateCommand | 引用将修改的行从DataSet写入数据源的Command对象 |
DeleteCommand | 引用从数据源中删除行的Command对象 |
DataAdapter的方法:
方法 | 说明 |
Fill | 使用SqlDataAdapter(或OleDbDataAdapter)的这个方法,从数据源增加或刷新行,并将这些行放到DataSet表中。Fill方法调用SelectCommand属性所指定的SELECT语句 |
Update | 使用DataAdapter对象的这个方法,将DataSet表的更改传送到相应的数据源中。该方法为DataSet的DataTable中每一指定的行调用相应的INSERT、UPDATE或DELETE命令 |
案例、编写一个小程序,创建一个窗体应用程序,然后在窗体应用程序中创建拖“DataGridView”和‘Button’控件。实现点击‘button’控件就会在“DataGridView”中显示
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.Data.SqlClient; namespace DataAdpter { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { //创建连接字符串 string constr = "Data Source =.;Initial Catalog = ExampleInfo;Integrated Security = True"; //创建连接对象 using (SqlConnection conn = new SqlConnection(constr)) { //床架sql语句 string sql = "select * from StudentTable"; //创建command对象 using (SqlCommand cmd = new SqlCommand(sql, conn)) {
//创建一个DataTable对象 DataTable ds = new DataTable(); using (SqlDataAdapter adapter = new SqlDataAdapter(sql, constr)) { //打开数据库 conn.Open(); adapter.Fill(ds); }
//绑定dataGridView控件
this.dataGridView1.DataSource = ds; } } } } }
//上述的代码中Datable可以改成DataSet
private void button1_Click_1(object sender, EventArgs e) { //创建连接字符串 string constr = "Data Source =.;Initial Catalog = ExampleInfo;Integrated Security = True"; //创建连接对象 using (SqlConnection conn = new SqlConnection(constr)) { //床架sql语句 string sql = "select * from StudentTable"; //创建command对象 using (SqlCommand cmd = new SqlCommand(sql, conn)) { DataSet ds = new DataSet(); using (SqlDataAdapter adapter = new SqlDataAdapter(sql, constr)) { //打开数据库 conn.Open(); adapter.Fill(ds); } this.dataGridView1.DataSource = ds.Tables[0]; } } }
六、封装SqlHelper
为什么要封装成SqlHelper?
如果不封装的话,我们每写一次查询就要写一次连接字符串,连接对象等等,如果一个程序当中有n个,就要写n此。那么不如将其封装起来,可以直接调用。我们只用提供sql语句和参数就可以了
封装第一步:创建一个项目:窗体应用程序(由于我会直接在这里写案例,所以就直接创建窗体),名称:LoginInfo
封装第二步:如下图
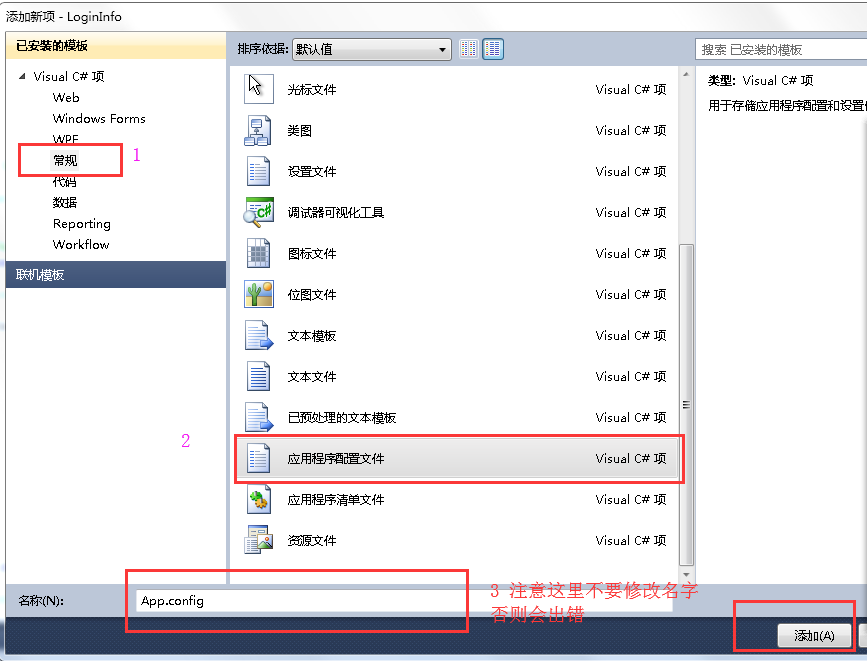
封装第二步骤,在App.config中添加如下代码

懒人这里复制(还是要多多手打哦):
<configuration> <connectionStrings> <add name="mssqlsrever" connectionString="Data Source =.;Initial Catalog =ExampleInfo;Integrated Security = True" /> </connectionStrings>
封装第三步:创建SqlHelper类
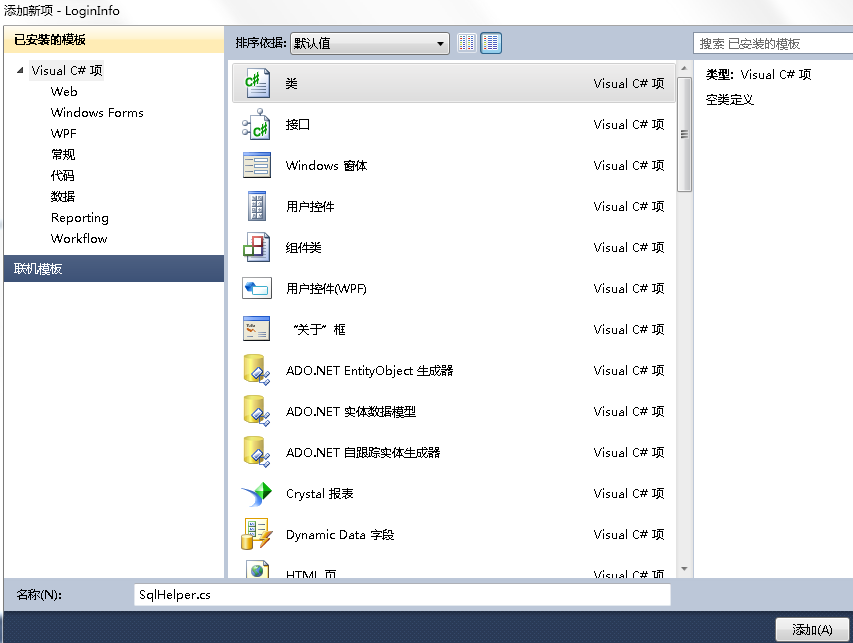
封装第五步:上代码
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Configuration; using System.Data.SqlClient; using System.Data; namespace LoginInfo { public class SqlHelper {//定义一个链接字符串 //readOnly 修饰的变量,只能在初始话的时候赋值,以及在构造函数中赋值 //读取配置文件中的链接字符串 private static readonly string constr = ConfigurationManager.ConnectionStrings["mssqlsrever"].ConnectionString; //执行增删改的方法 ExecuteNonQuery params SqlParameter[] paras 可能存在sql语句中带有参数,那么需要把参数给加进去 public static int ExecuteNonQuery(string sql, params SqlParameter[] paras) { using (SqlConnection conn = new SqlConnection(constr)) { using (SqlCommand cmd = new SqlCommand(sql, conn)) { if (paras != null) { cmd.Parameters.AddRange(paras); } conn.Open(); return cmd.ExecuteNonQuery(); } } } //执行查询,返回单个值方法 ExecuteScalar public static object ExecuteScalar(string sql, params SqlParameter[] paras) { using (SqlConnection conn = new SqlConnection(constr)) { using (SqlCommand comm = new SqlCommand(sql, conn)) { if (paras != null) { comm.Parameters.AddRange(paras); } conn.Open(); return comm.ExecuteScalar(); } } } //执行查询 返回多行多列的方法 ExecuteReader public static SqlDataReader ExecuteReader(string sql, params SqlParameter[] paras) { using (SqlConnection conn = new SqlConnection(constr)) { using (SqlCommand comm = new SqlCommand(sql, conn)) { if (paras != null) { comm.Parameters.AddRange(paras); } try { conn.Open(); return comm.ExecuteReader(System.Data.CommandBehavior.CloseConnection); } catch { conn.Close(); conn.Dispose(); throw; } } } } //执行查询 返回DataTable ExecuteDataTable public static DataTable ExecuteDataTable(string sql, params SqlParameter[] paras) { DataTable dt = new DataTable(); using (SqlDataAdapter adapter = new SqlDataAdapter(sql, constr)) { if (paras != null) { adapter.SelectCommand.Parameters.AddRange(paras); } adapter.Fill(dt); } return dt; } } }
到这里这个封装的SqlHelper类就好了。然后我会在这里写一个简单的小案例。
七、综合小案例
将第六步做完之后,我们直接使用这个封装好的SqlHelper
(一)在form1 中拖拉控件,达到以下效果
(二)编写程序,就是增删改查,这里我直接放代码
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.Data.SqlClient; namespace LoginInfo { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { //进入窗体之后就加载数据 LoadDate(); } private void LoadDate() { String sql = "select * from StudentTable"; this.dataGridView1.DataSource= SqlHelper.ExecuteDataTable(sql); } private void button1_Click(object sender, EventArgs e) { string Sname = textBox1.Text.Trim(); //Trim() 移除多余的空格 string Sage = textBox2.Text.Trim(); string Ssex = textBox3.Text.Trim(); string sql = "insert into StudentTable(Sname,Sage,Ssex) values (@Sname,@Sage,@Ssex)"; //这里是创建参数,更加的安全,防止不法人员注入sql语句,造成错误 SqlParameter[] paras = new SqlParameter[] { new SqlParameter("@Sname",SqlDbType.NVarChar,50){Value = Sname}, new SqlParameter("@Sage",SqlDbType.NVarChar,50){Value = Sage}, new SqlParameter("@Ssex",SqlDbType.NVarChar,50){Value = Ssex} }; //因为执行的是插入操作,所以调用ExecuteNonQuery int x = SqlHelper.ExecuteNonQuery(sql,paras); if (x > 0) { MessageBox.Show("新增成功"); //成功的同时,重新加载表格中的数据 LoadDate(); } else { MessageBox.Show("新增失败"); } } private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e) { //行点击事件,点击之后,行会吧数据给修改那一栏 DataGridViewRow current = dataGridView1.CurrentRow; textBox6.Text = current.Cells["Sname"].Value.ToString(); textBox5.Text = current.Cells["Sage"].Value.ToString(); textBox4.Text = current.Cells["Ssex"].Value.ToString(); label8.Text = current.Cells["Sid"].Value.ToString(); } private void button2_Click(object sender, EventArgs e) { string Sname = textBox6.Text; string Sage = textBox5.Text; string Ssex = textBox4.Text; string Sid = label8.Text; string sql = "update StudentTable set Sname =@Sname ,Sage = @Sage,Ssex = @Ssex where Sid = @Sid"; SqlParameter[] paras = new SqlParameter[]{ new SqlParameter("@Sname",SqlDbType.NVarChar,50){Value = Sname}, new SqlParameter("@Sage",SqlDbType.NVarChar,50){Value = Sage}, new SqlParameter("@Ssex",SqlDbType.NVarChar,50){Value = Ssex}, new SqlParameter("@Sid",SqlDbType.Int){Value = Sid} }; int x = SqlHelper.ExecuteNonQuery(sql,paras); if (x > 0) { MessageBox.Show("修改成功"); LoadDate(); } else { MessageBox.Show("修改失败"); } } private void button3_Click(object sender, EventArgs e) { string Sid = label8.Text; string sql = "delete from StudentTable where Sid = @Sid"; SqlParameter[] paras = new SqlParameter[]{ new SqlParameter("@Sid",SqlDbType.Int){Value = Sid} }; int x = SqlHelper.ExecuteNonQuery(sql, paras); if (x > 0) { MessageBox.Show("删除成功"); LoadDate(); } else { MessageBox.Show("删除失败"); } } } }
注意:
由于DataGridView还需要设置一些东西:
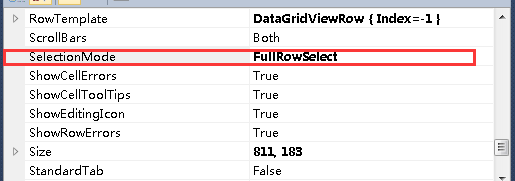
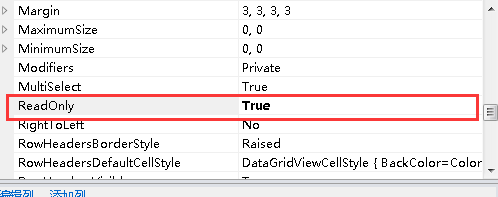
后续可能还会有补充,由于我是初学,所以有很多东西,不够详细,希望谅解