需要配置hbase-site.xml:
<property>
<name>hbase.cluster.distributed</name>
<value>true</value>
</property>
<property>
<name>hbase.master</name>
<value>主机名(zhz100):60000</value>
</property>
<property>
<name>hbase.zookeeper.property.dataDir</name>
<value>/usr/java/zookeeper3.4.10/temp</value>
</property>
<property>
<name>hbase.zookeeper.quorum</name>
<value>主机1(zhz100),主机2,主机3</value>
</property>
<property>
<name>hbase.zookeeper.property.clientPort</name>
<value>2181</value>
</property>
配置application.yml:
server:
port: 8080
hbase:
conf:
confMaps:
‘hbase.zookeeper.quorum’ : ‘IP地址1:2181,IP地址2:2181’
pom.xml 引入的依赖:
org.apache.hbase
hbase-client
1.2.0
org.slf4j
slf4j-log4j12
org.springframework.data
spring-data-hadoop
2.5.0.RELEASE
org.apache.hadoop
hadoop-hdfs
2.5.1
org.springframework.data
spring-data-hadoop-core
2.4.0.RELEASE
org.apache.hbase
hbase
1.2.1
pom
HbaseDemo 对hbase数据库进行操作:
public class HbaseDemo{
private static Configuration conf = HBaseConfiguration.create();
private static Admin admin;
static {
conf.set(“hbase.rootdir”, “hdfs://node1:9000/hbase”);
// 设置Zookeeper,直接设置IP地址
conf.set(“hbase.zookeeper.quorum”, “主机1(zhz100),主机2,主机3”);
try {
Connection connection = ConnectionFactory.createConnection(conf);
admin = connection.getAdmin();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 创建表,可以同时创建多个列簇
*
* @param tableName
* @param columnFamily
*/
public static void createTable(String tableName, String... columnFamily) {
TableName tableNameObj = TableName.valueOf(tableName);
try {
if (admin.tableExists(tableNameObj)) {
System.out.println("Table : " + tableName + " already exists !");
} else {
HTableDescriptor td = new HTableDescriptor(tableNameObj);
int len = columnFamily.length;
for (int i = 0; i < len; i++) {
HColumnDescriptor family = new HColumnDescriptor(columnFamily[i]);
td.addFamily(family);
}
admin.createTable(td);
System.out.println(tableName + " 表创建成功!");
}
} catch (Exception e) {
e.printStackTrace();
System.out.println(tableName + " 表创建失败!");
}
}
/*
* 创建表:cross_history 在测试类中测试:Demo2ApplicationTests
* */
@Test
public void testCreateTable() {
HbaseDemo.createTable(“dr_ps_20181112”, “trademark” , “dr_type” , “service_id” }
/**
* 删除表
*
*/
public void delTable(String tableName) {
TableName tableNameObj = TableName.valueOf(tableName);
try {
if (this.admin.tableExists(tableNameObj)) {
admin.disableTable(tableNameObj);
admin.deleteTable(tableNameObj);
System.out.println(tableName + " 表删除成功!");
} else {
System.out.println(tableName + " 表不存在!");
}
} catch (Exception e) {
e.printStackTrace();
System.out.println(tableName + " 表删除失败!");
}
}
/*
*插入数据
* */
public static void insertRecord(String tableName, String rowKey, String columnFamily, String qualifier, String value) {
try {
Connection connection = ConnectionFactory.createConnection(conf);
Table table = connection.getTable(TableName.valueOf(tableName));
Put put = new Put(rowKey.getBytes());
put.addColumn(Bytes.toBytes(columnFamily), Bytes.toBytes(qualifier), Bytes.toBytes(value));
table.put(put);
table.close();
connection.close();
System.out.println(tableName + " 表插入数据成功!");
} catch (Exception e) {
e.printStackTrace();
System.out.println(tableName + " 表插入数据失败!");
}
}
/*
* 根据KEYROW删除表中的数据
* */
public static void deleteRecord(String tableName, String rowKey) {
try {
Connection connection = ConnectionFactory.createConnection(conf);
Table table = connection.getTable(TableName.valueOf(tableName));
Delete del = new Delete(rowKey.getBytes());
table.delete(del);
System.out.println(tableName + " 表删除数据成功!");
} catch (Exception e) {
e.printStackTrace();
System.out.println(tableName + " 表删除数据失败!");
}
}
/*
* 根据表名字获取所有数据
* */
public static List getAll(String tableName) {
try {
Connection connection = ConnectionFactory.createConnection(conf);
Table table = connection.getTable(TableName.valueOf(tableName));
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
List list = new ArrayList();
for (Result r : scanner) {
list.add®;
}
scanner.close();
System.out.println(tableName + " 表获取所有记录成功!");
return list;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
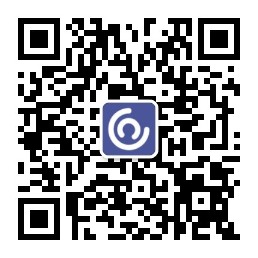
// 删除表
public static void deleteTable(String tableName) {
try {
Connection connection = ConnectionFactory.createConnection(conf);
Admin admin = connection.getAdmin();
TableName table = TableName.valueOf(tableName);
admin.disableTable(table);//使表失效
admin.deleteTable(table);//删除表
System.out.println("delete table " + tableName + " ok.");
} catch (IOException e) {
e.printStackTrace();
}
}
注*此为项目中部分代码,出现问题可以联系我[email protected],大家共同探讨。