309. Best Time to Buy and Sell Stock with Cooldown
Description:
Say you have an array for which the element is the price of a given stock on day i.
Design an algorithm to find the maximum profit. You may complete as many transactions as you like (ie, buy one and sell one share of the stock multiple times) with the following restrictions:
- You may not engage in multiple transactions at the same time (ie, you must sell the stock before you buy again).
- After you sell your stock, you cannot buy stock on next day. (ie, cooldown 1 day)
Example:
Input: [1,2,3,0,2] Output: 3 Explanation: transactions = [buy, sell, cooldown, buy, sell]
解题思路:
(1)动态规划解法:
这道题可以用动态规划思路来解决。这道题的难点在于如何写出状态转移方程。下面我们来分析一下题目:
在每一次股票操作之后,都可能出现三种状态:休息(rest)、持有(hold)和已卖出(sold)。下图是这三种状态的状态机。
1)对于rest,有两种情况:一种是,上一个时间再休息,现在接着休息。另一种是,上一个时间是卖出,那么现在必须是休息状态。
2)对于hold,有两种情况:一种是,上一个时间是休息,现在可以买入股票,变成持有状态。另一种是,上一个时间是持有,现在可以暂停交易进入休息,依然变成持有状态。
3)对于sold,只能是把持有的股票卖出,变成已卖出状态。
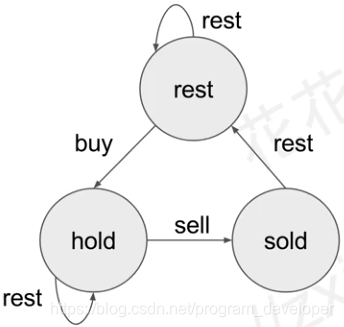
根据以上分析写出,状态转移方程:
带入例子中,验证状态转移方程是否正确:
已经AC的代码:
class Solution:
def maxProfit(self, prices):
"""
:type prices: List[int]
:type: int
"""
if len(prices) <= 1:
return 0
hold = [0] * len(prices)
sold = [0] * len(prices)
rest = [0] * len(prices)
hold[0] = -prices[0]
sold[0] = -float("inf")
for i in range(1,len(prices)):
hold[i] = max(hold[i - 1], rest[i - 1] - prices[i])
sold[i] = hold[i - 1] + prices[i]
rest[i] = max(rest[i - 1], sold[i - 1])
return max(sold[-1], rest[-1])
solution = Solution()
Input = [1, 2, 3, 0, 2]
print(solution.maxProfit(Input))
Reference:
【1】花花酱 LeetCode 309. Best Time to Buy and Sell Stock with Cooldown(视频)
【2】【LeetCode】309. Best Time to Buy and Sell Stock with Cooldown