6.3 二维数组的声明和引用
声明:数据类型 标识符[常量表达式1][常量表达式2];int a[3][4];
表示a为整型二维数组,其中第一维有3个下标(0~2),第二维有4个下标(0~3),数组元素12个,可以用来存放3行4列的整型数据表格。可以理解为:
a[0]——a 00 a01 a02 a03
a[1]——a10 a11 a12 a13
a[2]——a20 a21 a22 a23存储顺序是按行存储a00 a01 a02 a03 a10 a11 a12 a13 a20 a21 a22 a23
引用的时候下标不可越界,例如b[1][5]=a[2][3]/2,不可以写成a[3][4],否则发生错误。
二维数组的初始化
将所有数据写在一个{}内,按顺序赋值,例如:static int a[3][4]={1,2,3,4,5,6,7,8,9,10,11,12};
分行给二维数组赋初值,例如:static int a[3][4]={{1,2,3,4},{5,6,7,8},{9,10,11,12}};
可以对部分元素赋初值,例如:static int a[3][4]={{1},{0,6},{0,0,11}};
数组作为函数参数
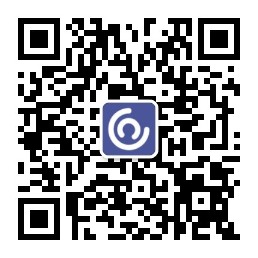
数组元素作为实参,与单个变量一样。
数组名作为参数,形实参数都应是数组名,类型要一样,传送的是数组首地址。对形参数组的改变会直接影响到实参数组。
#include <iostream> using namespace std; void RowSum(int A[][4], int nrow) //计算二维数组A每行元素的值的和,nrow是行数 { for (int i = 0; i < nrow; i++) { for(int j = 1; j < 4; j++) A[i][0] += A[i][j]; } } int main() //主函数 { int Table[3][4] = {{1,2,3,4},{2,3,4,5},{3,4,5,6}}; //声明并初始化数组 for (int i = 0; i < 3; i++) //输出数组元素 { for (int j = 0; j < 4; j++) cout << Table[i][j] << " "; cout << endl; } RowSum(Table,3); //调用子函数,计算各行和 for (i = 0; i < 3; i++) //输出计算结果 { cout << "Sum of row " << i << " is " <<Table[i][0]<< endl; } }
运行结果:
1 2 3 4
2 3 4 5
3 4 5 6
Sum of row 0 is 10
Sum of row 1 is 14
Sum of row 2 is 18
10 14 18
6.3 对象数组
声明:类名 数组名[元素个数];
访问方法:通过下标访问 数组名[下标].成员名
初始化:数组中每一个元素对象被创建时,系统都会调用类构造函数初始化该对象。
通过初始化列表赋值:point A[2]={point(1,2),point(3,4)};
如果没有为数组显示指定初始值,数组元素使用默认值初始化(调用默认构造函数)
//Point.h #if !defined(_POINT_H) #define _POINT_H class Point { public: Point(); Point(int xx,int yy); ~Point(); void Move(int x,int y); int GetX() {return X;} int GetY() {return Y;} private: int X,Y; }; #endif //Point.cpp #include<iostream> using namespace std; #include "Point.h" Point::Point() { X=Y=0; cout<<"Default Constructor called."<<endl; } Point::Point(int xx,int yy) { X=xx; Y=yy; cout<< "Constructor called."<<endl; } Point ::~Point() { cout<<"Destructor called."<<endl; } void Point ::Move(int x,int y) { X=x; Y=y; } #include<iostream> #include "Point.h" using namespace std; int main() { cout<<"Entering main..."<<endl; Point A[2]; for(int i=0;i<2;i++) A[i].Move(i+10,i+20); cout<<"Exiting main..."<<endl; return 0; }