版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/maotianyi941005/article/details/85136166
347. Top K Frequent Elements(Medium)
Given a non-empty array of integers, return the k most frequent elements.
Example 1:
Input: nums = [1,1,1,2,2,3], k = 2 Output: [1,2]
Example 2:
Input: nums = [1], k = 1 Output: [1]
Note:
- You may assume k is always valid, 1 ≤ k ≤ number of unique elements.
- Your algorithm's time complexity must be better than O(n log n), where n is the array's size.
求频率最高的k个数字,时间复杂度要优于 O(n log n),
第一个想到先计算每个数字的频率-》collections.Counter
然后根据频率排序取最后k个的数字
做这道题卡在如何对字典排序,参考了《
(补充了一种直接使用count.most_common(n)的方法,)count.most_common(n)返回list,对count按照计数排序返回数量最多的n个,返回的list的格式是[(key1 , val1 ) , (key2,val2),...,(keyn,valn)]
class Solution:
def topKFrequent(self, nums, k):
"""
:type nums: List[int]
:type k: int
:rtype: List[int]
"""
count = collections.Counter(nums)
k_freq = count.most_common(k)
return [k[0] for k in k_freq]
下面两种都是把dict先排序
扫描二维码关注公众号,回复:
4798258 查看本文章
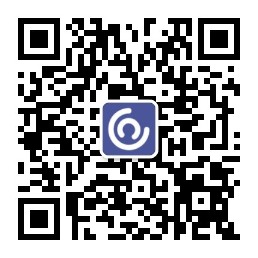
class Solution:
def topKFrequent(self, nums, k):
"""
:type nums: List[int]
:type k: int
:rtype: List[int]
"""
count = collections.Counter(nums)
l = [[val,key] for key,val in count.items()]
l.sort()
freq = [v[1] for v in l]
return freq[-k:]
还有一种利用sorted()里的key参数根据dict的val排序,然后利用odereddict顺序不变的特性直接取末k个key返回
class Solution:
def topKFrequent(self, nums, k):
"""
:type nums: List[int]
:type k: int
:rtype: List[int]
"""
count = collections.Counter(nums)
freq = collections.OrderedDict(sorted(count.items(),key=lambda t:t[1])) #sort according to val
freq = list(freq.keys())
return freq[-k:]