滤波器
cv2.filter2D(src, ddepth, kernel[, dst[, anchor[, delta[, borderType]]]]) dst
- src – input image.
- dst – output image of the same size and the same number of channels as src.
- ddepth –
desired depth of the destination image; if it is negative, it will be the same as src.depth(); the followin
– src.depth() = CV_8U, ddepth = -1/CV_16S/CV_32F/CV_64F
– src.depth() = CV_16U/CV_16S, ddepth = -1/CV_32F/CV_64F
– src.depth() = CV_32F, ddepth = -1/CV_32F/CV_64F
– src.depth() = CV_64F, ddepth = -1/CV_64F
when ddepth=-1, the output image will have the same depth as the source. - kernel – convolution kernel (or rather a correlation kernel), a single-channel floating point matrix; if you want to apply different kernels to different channels, split the image into separate color planes using split() and process them individually.
- anchor – anchor of the kernel that indicates the relative position of a filtered point within the kernel; the anchor should lie within the kernel; default value (-1,-1) means that the anchor is at the kernel center.
- delta – optional value added to the filtered pixels before storing them in dst.
- borderType – pixel extrapolation method (see borderInterpolate() for details).
将一个图片与一个核做卷积
归一化盒子滤波器
cv2.blur(src, ksize[, dst[, anchor[, borderType]]]) dst
- src – input image; it can have any number of channels, which are processed independently, but the depth should be CV_8U, CV_16U, CV_16S, CV_32F or CV_64F.
- dst – output image of the same size and type as src.
- ksize – blurring kernel size.
- anchor – anchor point; default value Point(-1,-1) means that the anchor is at the kernel center.
- borderType – border mode used to extrapolate pixels outside of the image.
- The function smoothes an image using the kernel:
盒子滤波器
cv2.boxFilter(src, ddepth, ksize[, dst[, anchor[, normalize[, borderType]]]]) dst
- src – input image.
- dst – output image of the same size and type as src.
- ddepth – the output image depth (-1 to use src.depth()).
- ksize – blurring kernel size.
- anchor – anchor point; default value Point(-1,-1) means that the anchor is at the kernel center.
- normalize – flag, specifying whether the kernel is normalized by its area or not.
- borderType – border mode used to extrapolate pixels outside of the image.
- The function smoothes an image using the kernel:
where
高斯滤波器
标准的高斯核函数进行滤波。不考虑像素是否具有相同的灰度值,也不考虑像素是否位于边缘,结果则趋向于模糊边缘,有时不受欢迎的。
cv2.GaussianBlur(src, ksize, sigmaX[, dst[, sigmaY[, borderType]]])
dst
- src – input image; the image can have any number of channels, which are processed independently, but the depth should be CV_8U, CV_16U, CV_16S, CV_32F or CV_64F.
- dst – output image of the same size and type as src.
- ksize – Gaussian kernel size. ksize.width and ksize.height can differ but they both must be positive and odd. Or, they can be zero’s and then they are computed from sigma* .
- sigmaX – Gaussian kernel standard deviation in X direction.
- sigmaY – Gaussian kernel standard deviation in Y direction; if sigmaY is zero, it is set to be equal to sigmaX, if both sigmas are zeros, they are computed from ksize.width and ksize.height , respectively (see getGaussianKernel() for details); to fully control the result regardless of possible future modifications of all this semantics, it is recommended to specify all of ksize, sigmaX, and sigmaY.
- borderType – pixel extrapolation method (see borderInterpolate() for details).
*如果想要创建一个高斯核,你可以用cv2.getGaussianKernel( kszie , sigma [,ktype])函数.
中值滤波
中心元素的像素值可能是原始图像中不存在的值,而中指滤波器则是原图像的值,有效的减小了噪声,对于椒盐噪声。核大小必须是奇数值。
非线性滤波:Blurs an image using
cv2.medianBlur(src, ksize[, dst])
dst
- src – input 1-, 3-, or 4-channel image; when ksize is 3 or 5, the image depth should be CV_8U, CV_16U, or CV_32F, for larger aperture sizes, it can only be CV_8U.
- dst – destination array of the same size and type as src.
- ksize – aperture linear size; it must be odd and greater than 1, for example: 3, 5, 7 …
双边滤波
非线性滤波:是结合图像的空间邻近度和像素值相似度的一种折中处理,同时考虑空域信息和灰度相似性,达到保边去噪的目的。双边滤波器的好处是可以做边缘保存(edge preserving),一般过去用的维纳滤波或者高斯滤波去降噪,都会较明显地模糊边缘,对于高频细节的保护效果并不明显。
原理:通过一个颜色差范围和像素距离范围来确定权重,进而影响中心像素的像素值。对于空间域(spatial domain )和像素范围域(range domain)来分别介绍。
- 空间域:距离越远,权重越小。
- 值域范围域:像素与中心差别越大,权重越小。弱化高斯模糊,在边缘区域像素值差别较大的区域。
which was defined for, and is highly effective at noise removal while preserving edges.But the operation is slower compared to other filters.
cv2.bilateralFilter(src, d, sigmaColor, sigmaSpace[, dst[, borderType]]) dst
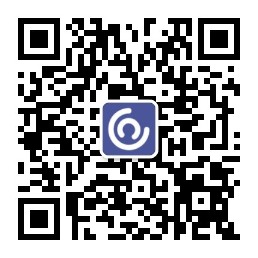
- src – Source 8-bit or floating-point, 1-channel or 3-channel image.
- dst – Destination image of the same size and type as src .
- d – Diameter of each pixel neighborhood that is used during filtering. If it is non-positive, it is computed from sigmaSpace .
- sigmaColor – Filter sigma in the color space. A larger value of the parameter means that farther colors within the pixel neighborhood (see sigmaSpace ) will be mixed together, resulting in larger areas of semi-equal color.定义多大的颜色差来起作用。
- sigmaSpace – Filter sigma in the coordinate space. A larger value of the parameter means that farther pixels will influence each other as long as their colors are close enough (see sigmaColor ). When d>0 , it specifies the neighborhood size regardless of sigmaSpace . Otherwise, d is proportional to sigmaSpace .定义多大的距离内的像素起作用。