1. 创建数据库
--创建文件对象表
CREATE TABLE sys_file (
id bigint NOT NULL identity(1,1),
name nvarchar(100),--文件名称
size nvarchar(100),--文件大小
type int DEFAULT NULL ,-- '文件类型',
url varchar(200) DEFAULT NULL ,-- 'URL地址',
create_date datetime DEFAULT NULL--'创建时间',
PRIMARY KEY (id)
)
2. 创建文件对象
package com.lifp.common.domain;
import java.io.Serializable;
import java.util.Date;
public class FileDO implements Serializable {
private static final long serialVersionUID = 1L;
//
private Long id;
// 文件类型
private Integer type;
// URL地址
private String url;
// 创建时间
private Date createDate;
//文件名称
private String name;
//文件大小
private String size;
public FileDO() {
super();
}
public FileDO(String name,String size, Integer type, String url, Date createDate) {
super();
this.name=name;
this.size=size;
this.type = type;
this.url = url;
this.createDate = createDate;
}
/**
* 设置:
*/
public void setId(Long id) {
this.id = id;
}
/**
* 获取:
*/
public Long getId() {
return id;
}
/**
* 设置:文件类型
*/
public void setType(Integer type) {
this.type = type;
}
/**
* 获取:文件类型
*/
public Integer getType() {
return type;
}
/**
* 设置:URL地址
*/
public void setUrl(String url) {
this.url = url;
}
/**
* 获取:URL地址
*/
public String getUrl() {
return url;
}
/**
* 设置:创建时间
*/
public void setCreateDate(Date createDate) {
this.createDate = createDate;
}
/**
* 获取:创建时间
*/
public Date getCreateDate() {
return createDate;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSize() {
return size;
}
public void setSize(String size) {
this.size = size;
}
@Override
public String toString() {
return "FileDO{" +
"id=" + id +
", name=" + name +
", size=" + size +
", type=" + type +
", url='" + url + '\'' +
", createDate=" + createDate +
'}';
}
}
3. Dao
package com.lifp.common.dao;
import java.util.List;
import java.util.Map;
import org.apache.ibatis.annotations.Mapper;
import com.lifp.common.domain.FileDO;
@Mapper
public interface FileDao {
FileDO get(Long id);
List<FileDO> list(Map<String,Object> map);
int count(Map<String,Object> map);
int save(FileDO file);
int update(FileDO file);
int remove(Long id);
int batchRemove(Long[] ids);
}
4. Mapper
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.lifp.common.dao.FileDao">
<select id="get" resultType="com.lifp.common.domain.FileDO">
select id,name,size,type,url,create_date from sys_file where name LIKE '%'+#{name}+'%'
</select>
<select id="list" resultType="com.lifp.common.domain.FileDO">
select id,name,size,type,url,create_date from sys_file
<where>
<if test="id != null and id != ''"> and id = #{id} </if>
<if test="name != null and name != ''"> and name LIKE '%'+#{name}+'%' </if>
<if test="type != null and type != ''"> and type = #{type} </if>
<if test="url != null and url != ''"> and url = #{url} </if>
<if test="createDate != null and createDate != ''"> and create_date = #{createDate} </if>
</where>
<choose>
<when test="sort != null and sort.trim() != ''">
order by ${sort} ${order}
</when>
<otherwise>
order by id desc
</otherwise>
</choose>
<!-- <if test="offset != null and limit != null"> -->
<!-- limit #{offset}, #{limit} -->
<!-- </if> -->
</select>
<select id="count" resultType="int">
select count(*) from sys_file
<where>
<if test="id != null and id != ''"> and id = #{id} </if>
<if test="name != null and name != ''"> and name LIKE '%'+#{name}+'%' </if>
<if test="type != null and type != ''"> and type = #{type} </if>
<if test="url != null and url != ''"> and url = #{url} </if>
<if test="createDate != null and createDate != ''"> and create_date = #{createDate} </if>
</where>
</select>
<insert id="save" parameterType="com.lifp.common.domain.FileDO" useGeneratedKeys="true" keyProperty="id">
insert into sys_file
(
name,
size,
type,
url,
create_date
)
values
(
#{name},
#{size},
#{type},
#{url},
#{createDate}
)
</insert>
<update id="update" parameterType="com.lifp.common.domain.FileDO">
update sys_file
<set>
<if test="name != null">name = #{name}, </if>
<if test="size != null">size = #{size}, </if>
<if test="type != null">type = #{type}, </if>
<if test="url != null">url = #{url}, </if>
<if test="createDate != null">create_date = #{createDate}</if>
</set>
where id = #{id}
</update>
<delete id="remove">
delete from sys_file where id = #{value}
</delete>
<delete id="batchRemove">
delete from sys_file where id in
<foreach item="id" collection="array" open="(" separator="," close=")">
#{id}
</foreach>
</delete>
</mapper>
5. mabatis配置 application.xml
server.port=8081
demo.uploadPath=C:/var/uploaded_files/
spring.datasource.driver=com.microsoft.sqlserver.jdbc.SQLServerDriver
spring.datasource.url=jdbc:sqlserver://127.0.0.1:1433;DatabaseName=LiangShanHeroes
spring.datasource.username=lifangping
spring.datasource.password=123
#\u5B9A\u4E49\u521D\u59CB\u8FDE\u63A5\u6570
spring.datasource.initialSize=0
#\u5B9A\u4E49\u6700\u5927\u8FDE\u63A5\u6570
spring.datasource.maxActive=20
#\u5B9A\u4E49\u6700\u5927\u7A7A\u95F2
spring.datasource.maxIdle=20
#\u5B9A\u4E49\u6700\u5C0F\u7A7A\u95F2
spring.datasource.minIdle=1
#\u5B9A\u4E49\u6700\u957F\u7B49\u5F85\u65F6\u95F4
spring.datasource.maxWait=60000
#注意:mybatis配置文件
mybatis.config-locations=classpath:mybatis/mybatis-config.xml
mybatis.mapper-locations: classpath:mapper/*.xml
#注意:一定要对应mapper映射xml文件的所在路径
mybatis.type-aliases-package: com.lifp.model
# 注意:对应实体类的路径
#开启开启驼峰命名转换
mybatis.configuration.mapUnderscoreToCamelCase=true
#打印sql日志
logging.level.com.lifp.common.dao=debug
6. FileService
package com.lifp.common.service;
import java.util.List;
import java.util.Map;
import com.lifp.common.domain.FileDO;
public interface FileService {
FileDO get(Long id);
List<FileDO> list(Map<String, Object> map);
int count(Map<String, Object> map);
int save(FileDO sysFile);
int update(FileDO sysFile);
int remove(Long id);
int batchRemove(Long[] ids);
/**
* 判断一个文件是否存在
* @param url FileDO中存的路径
* @return
*/
Boolean isExist(String url);
}
7. FileServiceImpl
package com.lifp.common.service.impl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.io.File;
import java.util.List;
import java.util.Map;
import org.springframework.util.Assert;
import org.springframework.util.StringUtils;
import com.lifp.common.config.DemoConfig;
import com.lifp.common.dao.FileDao;
import com.lifp.common.domain.FileDO;
import com.lifp.common.service.FileService;
@Service
public class FileServiceImpl implements FileService {
@Autowired
private FileDao fileMapper;
@Autowired
private DemoConfig demoConfig;
@Override
public FileDO get(Long id){
return fileMapper.get(id);
}
@Override
public List<FileDO> list(Map<String, Object> map){
return fileMapper.list(map);
}
@Override
public int count(Map<String, Object> map){
return fileMapper.count(map);
}
@Override
public int save(FileDO sysFile){
return fileMapper.save(sysFile);
}
@Override
public int update(FileDO sysFile){
return fileMapper.update(sysFile);
}
@Override
public int remove(Long id){
return fileMapper.remove(id);
}
@Override
public int batchRemove(Long[] ids){
return fileMapper.batchRemove(ids);
}
@Override
public Boolean isExist(String url) {
Boolean isExist = false;
if (!StringUtils.isEmpty(url)) {
String filePath = url.replace("/files/", "");
filePath = demoConfig.getUploadPath() + filePath;
File file = new File(filePath);
if (file.exists()) {
isExist = true;
}
}
return isExist;
}
}
9. FileController
@ResponseBody
@PostMapping("/upload")
R upload(@RequestParam("file") MultipartFile file, HttpServletRequest request) {
String oriFileName = FileUtil.originalName(file.getOriginalFilename());
String size = FileUtil.getSize(file.getSize());
String fileName = FileUtil.renameToUUID(oriFileName);
FileDO sysFile = new FileDO(oriFileName, size, FileType.fileType(fileName), "/files/" + fileName, new Date());
try {
FileUtil.uploadFile(file.getBytes(), demoConfig.getUploadPath(), fileName);
} catch (Exception e) {
return R.error();
}
if (fileService.save(sysFile) > 0) {
return R.ok().put("fileName", sysFile.getUrl());
}
return R.error();
}
@ResponseBody
@GetMapping("/download")
String download(@RequestParam("path") String path, HttpServletResponse response) {
String fileName = path.substring(path.lastIndexOf("/") + 1);
if (fileName != null) {
// 设置文件路径
File file = new File(demoConfig.getUploadPath() + fileName);
// File file = new File(realPath , fileName);
if (file.exists()) {
response.setContentType("application/force-download");// 设置强制下载不打开
response.addHeader("Content-Disposition", "attachment;fileName=" + fileName);// 设置文件名
byte[] buffer = new byte[1024];
FileInputStream fis = null;
BufferedInputStream bis = null;
try {
fis = new FileInputStream(file);
bis = new BufferedInputStream(fis);
OutputStream os = response.getOutputStream();
int i = bis.read(buffer);
while (i != -1) {
os.write(buffer, 0, i);
i = bis.read(buffer);
}
return null;
} catch (Exception e) {
e.printStackTrace();
} finally {
if (bis != null) {
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
return null;
}
@RequestMapping("/preView")
@ResponseBody
public void preView(@RequestParam("path") String path, HttpServletResponse response) throws Exception {
String fileName = path.substring(path.lastIndexOf("/") + 1);
if (fileName != null) {
// 设置文件路径
File file = new File(demoConfig.getUploadPath() + fileName);
response.setContentType("application/pdf"); //预览pdf时的设置
FileInputStream in = new FileInputStream(file);
OutputStream out = response.getOutputStream();
byte[] b = new byte[512];
while ((in.read(b)) != -1) {
out.write(b);
}
out.flush();
in.close();
out.close();
}
}
10. File.html
<!DOCTYPE html>
<html>
<meta charset="utf-8">
<head th:include="include :: header">
</head>
<body class="gray-bg">
<div class="wrapper wrapper-content animated fadeInRight">
<div class="col-sm-12">
<div class="ibox">
<div class="ibox-body">
<div id="exampleToolbar" role="group">
</div>
<div >
<!-- COMPONENT START -->
<button type="button" class="layui-btn" id="test1">
<i class="fa fa-cloud"></i>上传文件
</button>
</div>
<table id="exampleTable" data-mobile-responsive="true">
</table>
</div>
</div>
</div>
</div>
<div th:include="include :: footer"></div>
<script src="/js/layui.js"></script>
<script type="text/javascript" src="/js/appjs/common/file/file.js"></script>
</body>
</html>
10. File.js
var prefix = "/common/file"
$(function() {
load();
layui.use('upload', function () {
var upload = layui.upload;
//执行实例
var uploadInst = upload.render({
elem: '#test1', //绑定元素
url: '/common/file/upload', //上传接口
size: 300*1024*1024, //上传大小限制,300M
accept: 'file',
done: function (r) {
layer.msg(r.msg);
reLoad();
},
error: function (r) {
layer.msg(r.msg);
}
});
});
});
function load() {
$('#exampleTable')
.bootstrapTable(
{
method : 'get', // 服务器数据的请求方式 get or post
url : prefix + "/list", // 服务器数据的加载地址
//showRefresh : true,
//showToggle : true,
//showColumns : true,
iconSize : 'outline',
toolbar : '#exampleToolbar',
striped : true, // 设置为true会有隔行变色效果
dataType : "json", // 服务器返回的数据类型
pagination : true, // 设置为true会在底部显示分页条
// queryParamsType : "limit",
// //设置为limit则会发送符合RESTFull格式的参数
singleSelect : false, // 设置为true将禁止多选
// contentType : "application/x-www-form-urlencoded",
// //发送到服务器的数据编码类型
pageSize : 10, // 如果设置了分页,每页数据条数
pageNumber : 1, // 如果设置了分布,首页页码
search : true, // 是否显示搜索框
showColumns : true, // 是否显示内容下拉框(选择显示的列)
sidePagination : "client", // 设置在哪里进行分页,可选值为"client" 或者
// "server"
queryParams : function(params) {
return {
limit: params.limit,
offset:params.offset,
username:$('#searchName').val(),
sort:'create_date',
order:'desc'
};
},
responseHandler: function(data){
return data.rows;
},
// //请求服务器数据时,你可以通过重写参数的方式添加一些额外的参数,例如 toolbar 中的参数 如果
// queryParamsType = 'limit' ,返回参数必须包含
// limit, offset, search, sort, order 否则, 需要包含:
// pageSize, pageNumber, searchText, sortName,
// sortOrder.
// 返回false将会终止请求
columns : [
{
checkbox : true
},
{
field : 'id', // 列字段名
title : '序号' // 列标题
},
{
field : 'name',
title : '文件名',
formatter : function(value, row, index) {
var e = '<a href="#" mce_href="#" title="预览" onclick="preView(\''
+ row.url
+ '\')"><i class="fa fa-eye"></i> '+row.name+'</a> ';
return e;
}
},
{
field : 'size',
title : '文件大小'
},
{
field : 'type',
title : '类型'
},
{
field : 'createDate',
title : '创建时间'
},
{
title : '操作',
field : 'id',
align : 'center',
formatter : function(value, row, index) {
var e = '<a class="btn btn-primary btn-sm" href="#" mce_href="#" title="下载" onclick="downLoad(\''
+ row.url
+ '\')"><i class="fa fa-download"></i>下载</a> ';
var d = '<a class="btn btn-primary btn-sm" href="#" mce_href="#" title="编辑" onclick="edit(\''
+ row.id
+ '\')"><i class="fa fa-edit"></i>编辑</a> ';
var f = '<a class="btn btn-warning btn-sm" href="#" title="删除" mce_href="#" onclick="remove(\''
+ row.id
+ '\')"><i class="fa fa-remove"></i>删除</a> ';
return e+d+f;
}
} ]
});
}
function reLoad() {
$('#exampleTable').bootstrapTable('refresh');
}
function preView(path){
window.open(prefix+"/preView?path="+path);
}
function downLoad(path) {
window.location.href= prefix+"/download?path="+path;
}
function remove(id) {
layer.confirm('确定要删除选中的记录?', {
btn : [ '确定', '取消' ]
}, function() {
$.ajax({
url : prefix+"/remove",
type : "post",
data : {
'id' : id
},
success : function(r) {
if (r.code==0) {
layer.msg(r.msg);
reLoad();
}else{
layer.msg(r.msg);
}
}
});
})
}
function batchRemove() {
var rows = $('#exampleTable').bootstrapTable('getSelections'); // 返回所有选择的行,当没有选择的记录时,返回一个空数组
if (rows.length == 0) {
layer.msg("请选择要删除的数据");
return;
}
layer.confirm("确认要删除选中的'" + rows.length + "'条数据吗?", {
btn : [ '确定', '取消' ]
// 按钮
}, function() {
var ids = new Array();
//遍历所有选择的行数据,取每条数据对应的ID
$.each(rows, function(i, row) {
ids[i] = row['id'];
});
$.ajax({
type:'POST',
data:{"ids":ids},
url:prefix+'/batchRemove',
success:function(r){
if (r.code==0) {
layer.msg(r.msg);
reLoad();
}else{
layer.msg(r.msg);
}
}
});
}, function() {
});
}
扫描二维码关注公众号,回复:
4737571 查看本文章
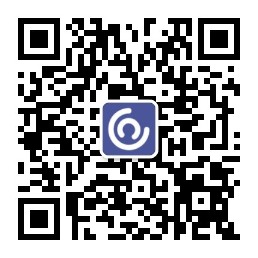