I am learning the Image processing,so I have to learn a programming language to implement various algorithm,and I just want to learn python,so I choose the python.
2018/9/25:
At first,I want to do some simple manipulate,so I try to open a image,immediately some problems stoped me
1.no module named 'Image'
>>> import Image
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
import Image
ModuleNotFoundError: No module named 'Image'
The solution:
First I install the image module,but it doesn't work, so I serched the baidu, someone said that you should install PIL moudle, because Image module had been includede in PIL module,so I installed the PIL module,and I enter "import Image",however it still doesn't work,then continue to ask help from baidu,a guy said:"you should use 'from PIL import Image'",I followed his advise,it's amazing,it worked.
But I am still confused,so I reserched for some tips again,finaly I totaly know why I was wrong.here are the conclusions
-
The Image module is included in PIL module,so you should use "PIL.Image' to use the functions of Image
-
In fact,I installed the Image module, when i look up the module I installed,I found it's image rather than Iamge,you know python's programer is similar with liunx system,they are strictlky distinguish alphabetic size,so you should use "import iamge.
2.path problem
>>> im=Image.open("C:\Users\yexuehua\Desktop\1.png")
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
this is a very funny problem,because it is a specious segment,as a matter of fact,if we use '\' in the strings python will recongonize it as a escape character,so this is a wrong path for python.
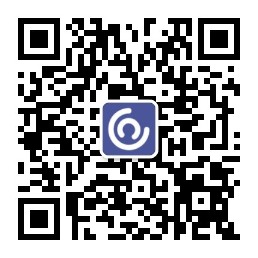
In orther to avoid this problem you should tell python this isn't a ESC,so you should add a 'r' before the string.In this way,it can work.
3.name 'imshow' is not defined
>>> imshow(im)
Traceback (most recent call last):
File "<pyshell#8>", line 1, in <module>
imshow(im)
NameError: name 'imshow' is not defined
I want to show the picture that I opened,but it output like this,again,"duniang",it turned out that I haven't install the matplotlib,this is a 2D drawing library,so if I want to show a picture,I must install this library.
2018/9/30:
After some days exploring,I begain to using the anaconda,beacuse I saw a lot of blogs which is telling about Image processing,most of them using the anaconda,because it include almost all the regular modules that image processing needs,so I install it,and I begain to follow a seria blogs to learn image processing.here is the url of blog:
https://www.cnblogs.com/denny402/p/5121501.html
Ok, I let's begain to look what I have learned these days
After some install course,the first thing I know from the blog is skimage package,It contains many submodules,every submodule has its own function,folowing list is the part of submodules:
Let's look into this package,
1.read/show/save/access pixels
if we want to read a iamge we can use
skimage.io.imread(fname)
"fname" is the path of picture.
if we want to show a picture,we can use
io.show(arr)
"arr" is the array of picture
if we want to read picture meanwhile convert it to gray,we can use
io.imread(fname,as_gray=Ture)
if we want to save image,we can use
io.imsave(name,arr)
Attention the name can be any path,any name.
So there are some simple operation of skimage package,it's very easy.and following code is some practice of these fuctions
@author: yexuehua
"""
from skimage import io,data,color
# only display the green channel
#im = io.imread('d:/python/visual_picture/2.jpg')
#a=im[:,:,1]
#io.imshow(a)
#genrate 5000 spiced salt randomly
#import numpy as np
#spice=data.chelsea()
#row,col,dims=spice.shape
#for i in range(5000):
# x=np.random.randint(0,row)
# y=np.random.randint(0,col)
# spice[x,y,:]=255
#io.imshow(spice)
#binaryzation
bina = data.astronaut()
bina_gray = color.rgb2gray(bina)
rows,clos=bina_gray.shape
for i in range(rows):
for j in range(clos):
if (bina_gray[i,j]>=0.5):
bina_gray[i,j]=1
else:
bina_gray[i,j]=0
io.imshow(bina_gray)
the pictures are read into program in the form of array,so access to array element is access to pixels.
the way to access colour picture is:
img[i,j,c](the i is row,the j is colum,the c is the colour channel)
the way to access gray picture is:
gray[i,j]
if we want to know some imformation about picture,we can use following functions:
from skimage import io,data
img=data.chelsea()
io.imshow(img)
print(type(img)) #显示类型
print(img.shape) #显示尺寸
print(img.shape[0]) #图片宽度
print(img.shape[1]) #图片高度
print(img.shape[2]) #图片通道数
print(img.size) #显示总像素个数
print(img.max()) #最大像素值
print(img.min()) #最小像素值
print(img.mean()) #像素平均值
2.data type and transition
We read the picture's data into numpy array,the type of array's data is so many,and they can convert from one to another,following table is the data type and data range:
following table is some function of data transition
we can also convert data type through the colour space of picture:
skimage.color.rgb2grey(rgb)
skimage.color.rgb2hsv(rgb)
skimage.color.rgb2lab(rgb)
skimage.color.gray2rgb(image)
skimage.color.hsv2rgb(hsv)
skimage.color.lab2rgb(lab)
for example,
from skimage import io,data,color
img=data.lena()
gray=color.rgb2gray(img)
io.imshow(gray)
Actually,we can use a function to do these work:
skimage.color.convert_colorspace(arr, fromspace, tospace)
For instance,
from skimage import io,data,color
img=data.coffee()
hsv=color.convert_colorspace(img,'RGB','HSV')
io.imshow(hsv)
Here is another intersting function, it can color picture based on the lable value,we can use this function to colour the sorted picture:
skimage.color.label2rgb(arr)
for example
from skimage import io,data,color
import numpy as np
img=data.astronut()
gray=color.rgb2gray(img)
rows,cols=gray.shape
labels=np.zeros([rows,cols])
for i in range(rows):
for j in range(cols):
if(gray[i,j]<0.4):
labels[i,j]=0
elif(gray[i,j]<0.75):
labels[i,j]=1
else:
labels[i,j]=2
dst=color.label2rgb(labels)
io.imshow(dst)
it's a very funny output isn't it?