965. Univalued Binary Tree
A binary tree is univalued if every node in the tree has the same value.
Return true
if and only if the given tree is univalued.
Example 1:
Input: [1,1,1,1,1,null,1]
Output: true
Example 2:
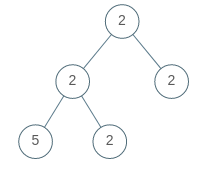
Input: [2,2,2,5,2]
Output: false
Note:
- The number of nodes in the given tree will be in the range
[1, 100]
. - Each node's value will be an integer in the range
[0, 99]
.
Code:
/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */ class Solution { public: bool isUnivalTree(TreeNode* root) { solve(root, root->val); return ans; } void solve(TreeNode* root, int num) { if (root->val != num) ans = false; if (root->left != NULL) solve(root->left, num); if (root->right != NULL) solve(root->right, num); } private: bool ans = true; };
967. Numbers With Same Consecutive Differences
Return all non-negative integers of length N
such that the absolute difference between every two consecutive digits is K
.
Note that every number in the answer must not have leading zeros except for the number 0
itself. For example, 01
has one leading zero and is invalid, but 0
is valid.
You may return the answer in any order.
Example 1:
Input: N = 3, K = 7
Output: [181,292,707,818,929]
Explanation: Note that 070 is not a valid number, because it has leading zeroes.
Example 2:
Input: N = 2, K = 1
Output: [10,12,21,23,32,34,43,45,54,56,65,67,76,78,87,89,98]
Code:
class Solution { public: vector<int> numsSameConsecDiff(int N, int K) { vector<int> ans; if (N == 1) { for (int i = 0; i < 10; ++i) ans.push_back(i); return ans; } for (int i = 1; i < 10; ++i) { string s = to_string(i); dfs(s, N, K, ans); } return ans; } void dfs(string s, const int N, const int K, vector<int>& ans) { if (s.length() == N) { ans.push_back(stoi(s)); return ; } int lastNum = s[s.length()-1] - '0'; int temp = lastNum + K; string dummy = s; if (temp >= 0 && temp < 10) { dummy += to_string(temp); dfs(dummy, N, K, ans); } if (K != 0) { int temp = lastNum - K; if (temp >= 0 && temp < 10) { s += to_string(temp); dfs(s, N, K, ans); } } } };