概览:Ajax(Asynchronous Javascript And XML)
解释为Javascript语言与服务器进行异步交互,传输的数据为XML(当然,传输的数据不只是XML,现在更多使用json数据)。
同步交互:客户端发出一个请求后,需要等待服务器响应结束后,才能发出第二个请求;
异步交互:客户端发出一个请求后,无需等待服务器响应结束,就可以发出第二个请求。
向服务器发送请求的四个途径:
1.浏览器地址栏,默认get请求
2.form表单
get请求
post请求
3.a标签,默认get请求
4.Ajax请求
特点:
异步请求
局部刷新
get
post
基于jquery的Ajax的实现(以下均省略urls.py,自行添加)
cdn链接:<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.min.js"></script>
简单流程:
1: <script>2: $(".Ajax").click(function () {3: //发送Ajax的请求的简单流程4: $.ajax({5: url:"", //请求url6: type:"get", //请求方式post7: success:function(data){ //回调函数8: console.log(data) //data就是传来的数据9: }10: })11: });12: </script>
Ajax传递数据:
index.html
1: <input type="text" id="num1">+<input type="text" id="num2">=<input type="text" id="ret"> <button class="cal">计算</button>2: <script>3: $(".cal").click(function () {4: $.ajax({5: url: "/cal/",6: type: "post",7: data: {8: "n1":$("#num1").val(),9: "n2":$("#num2").val(),10: },11: success:function (data) {12: console.log(data);13: $("#ret").val(data);14: }15: })16: });17: </script>
views.py
1: def cal(request):2:3: n1 = int(request.POST.get("n1"))4: n2 = int(request.POST.get("n2"))5: ret = n1 + n26: return HttpResponse(ret)
基于Ajax的登录验证
1: <form>2: 用户名 <input type="text" id="user">3: 密码 <input type="password" id="pwd">4: <input type="button" value="submit" class="login_btn"><span class="error"></span>5: </form>6: <script>7: $(".login_btn").click(function () {8: $.ajax({9: url:"/login/",10: type:"post",11: data:{12: "user":$("#user").val(),13: "pwd":$("#pwd").val(),14: },15: success:function (data) {16: console.log(data);17: // data->json字符串18: // 反序列化->object {}19: var data = JSON.parse(data);20: if (data.user){21: location.href="http://www.baidu.com"22: }23: else {24: $(".error").html(data.msg).css({"color": "red", "margin-left": "10px"})25: }26: }27: })28: });29: </script>
models.py
1: class User(models.Model):
2: name = models.CharField(max_length=32)
3: pwd = models.CharField(max_length=32)
views.py
1: from app01.models import User
2: def login(request):
3:4: print(request.POST)
5: user = request.POST.get("user")6: pwd = request.POST.get("pwd")
7: userobj = User.objects.filter(name=user, pwd=pwd).first()8: res = {"user": None, "msg": None}9: if userobj:
10: res["user"] = userobj.name11: else:
12: res["msg"] = "wrong!"13: import json
14: return HttpResponse(json.dumps(res))
基于form表单的文件上传
file_put.html
1: <h3>基于form表单的文件上传</h3>2: <form action="" method="post" enctype="multipart/form-data">3: 用户名 <input type="text" name="user">4: 头像 <input type="file" name="avatar">5: <input type="submit" >
views.py
扫描二维码关注公众号,回复:
4683267 查看本文章
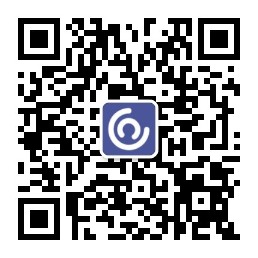
1: def file_put(request):2:3: if request.method == "POST":4: print(request.POST)5: print(request.FILES)6: file_obj = request.FILES.get("avatar")7: with open(file_obj.name, "wb") as f:8: for line in file_obj:9: f.write(line)10:11: return HttpResponse("OK")12:13: return render(request, "file_put.html")
请求头ContentType
ContentType指的是请求体的编码类型,常见的类型共有3种:
1 application/x-www-form-urlencoded
这应该是最常见的 POST 提交数据的方式了。浏览器的原生表单,如果不设置 enctype 属性,那么最终就会以 application/x-www-form-urlencoded 方式提交数据。请求类似于下面这样(无关的请求头在本文中都省略掉了):2 multipart/form-data
这又是一个常见的 POST 数据提交的方式。我们使用表单上传文件时,必须让表单的 enctype 等于 multipart/form-data。3 application/json
application/json 这个 Content-Type 作为响应头大家肯定不陌生。实际上,现在越来越多的人把它作为请求头,用来告诉服务端消息主体是序列化后的 JSON 字符串。由于 JSON 规范的流行,除了低版本 IE 之外的各大浏览器都原生支持 JSON.stringify,服务端语言也都有处理 JSON 的函数,使用 JSON 不会遇上什么麻烦。
基于Ajax的文件上传
html文件
1: <body>2: <h3>基于Ajax的文件上传</h3>3:4: <form action="" method="post" enctype="multipart/form-data">5: 用户名 <input type="text" id="user">6: 头像 <input type="file" id="avatar">7: <input type="button" class="btn" value="Ajax">8: </form>9: <script>10: $(".btn").click(function () {11: var formdata = new FormData();12: formdata.append("user", $("#user").val());13: formdata.append("avatar", $("#avatar")[0].files[0]);14: $.ajax({15: url:"",16: type:"post",17: contentType:false,18: processData:false,19: data:formdata,20: success:function (data) {21: console.log(data);22: }23: });24: });25: </script>26: </body>
views.py
1: def file_put(request):2:3: if request.method == "POST":4: print("body-->", request.body)5: print("POST-->", request.POST)6: print(request.FILES)7: # dowload file code8: # file_obj = request.FILES.get("avatar")9: # with open(file_obj.name, "wb") as f:10: # for line in file_obj:11: # f.write(line)12:13: return HttpResponse("OK")14:15: return render(request, "index.html")