版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/guangyacyb/article/details/85143299
1、protobuf 安装
安装好的目录如下:
protobuf-2.4.1/
|-- bin
|-- include
`-- lib
2、创建一个proto文件
package PAY;
message Person
{
optional int32 age = 1;
optional bytes name = 2;
}
3、创建write.cpp ,用于将对象序列化到文件
#include <iostream>
#include <fstream>
#include "pay.pb.h"
using namespace std;
int main()
{
PAY::Person person;
person.set_age(10);
person.set_name("jack");
fstream out("myfile.txt", ios::binary | ios::out | ios::trunc);
if(!out)
{
cerr << "open file myfile failed!\n";
return -1;
}
if (!person.SerializeToOstream(&out))
{
cerr << "write error!\n";
return -1;
}
}
4、创建read.cpp,用于从文件反序列化对象
#include <iostream>
#include <fstream>
#include "pay.pb.h"
using namespace std;
int main()
{
PAY::Person person;
fstream in("myfile.txt", ios::binary | ios::in);
if (!person.ParseFromIstream(&in))
{
cout << "read error" << endl;
return -1;
}
cout << person.age() << endl;
cout << person.name() << endl;
}
5、创建makefile 文件
TARGET=libpay.a
WRITE=write
READ=read
OBJ = pay.pb.o
$(shell rm -f *.cc *.h)
PROTOBUF_INCL = -I./protobuf-2.4.1/include
PROTOBUF_LIB = -L./protobuf-2.4.1/lib -lprotobuf
all: $(TARGET) $(WRITE) $(READ)
clean:
rm -f $(TARGET) $(WRITE) $(READ) $(OBJ) pay.pb.h pay.pb.cc
install:
pay.pb.o:pay.pb.cc
g++ -c -ggdb3 $(PROTOBUF_INCL) $<
$(TARGET): $(OBJ)
ar -curv $@ $?
ranlib $@
$(WRITE):write.cpp
g++ -ggdb3 -o $@ $^ pay.pb.cc $(PROTOBUF_INCL) $(PROTOBUF_LIB)
$(READ):read.cpp
g++ -ggdb3 -o $@ $^ pay.pb.cc $(PROTOBUF_INCL) $(PROTOBUF_LIB)
pay.pb.cc:pay.proto
LD_LIBRARY_PATH+=./protobuf-2.4.1/lib/ ./protobuf-2.4.1/bin/protoc -I=. --cpp_out=. $^
6、创建一个执行脚本
#!/bin/bash
export LD_LIBRARY_PATH=protobuf-2.4.1/lib/
./write
./read
综上,代码目录结构为:
protodata/
|-- makefile
|-- myfile.txt
|-- pay.proto
|-- protobuf-2.4.1
| |-- bin
| |-- include
| `-- lib
|-- read.cpp
|-- test.sh
`-- write.cpp
记得要先创建文件myfile.txt
执行
./test.sh
结果:
扫描二维码关注公众号,回复:
4653491 查看本文章
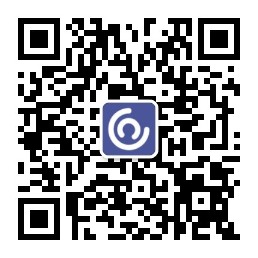
10
jack
参考: