所需工具下载
redis可视化工具,下载地址:添加链接描述
1:maven 引用jar包
<!--redis pom-->
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-redis</artifactId>
</dependency>
<!--序列化,反序列化-->
<dependency>
<groupId>com.dyuproject.protostuff</groupId>
<artifactId>protostuff-core</artifactId>
<version>1.0.8</version>
</dependency>
<dependency>
<groupId>com.dyuproject.protostuff</groupId>
<artifactId>protostuff-runtime</artifactId>
<version>1.0.8</version>
</dependency>
2.配置yml文件
#redis 配置
redis:
database: 0
host: 127.0.0.1
port: 6379
password:
jedis:
pool:
max-idle: 8 #连接池最大连接数(使用负值表示没有限制)
min-idle: 0 # 连接池中的最小空闲连接
max-active: 8 # 连接池最大阻塞等待时间(使用负值表示没有限制)
max-wait: -1 # 连接池中的最大空闲连接
timeout: 5000 # 连接超时时间(毫秒)
3.实现redis读取方法service类
创建redis 接口
import java.util.List;
/**
* @ClassName RedisService
* @Description TODO
* @Author
* @Date 2018/11/21 17:20
* @Version 1.0
**/
public interface RedisService {
/**
* 存入单个对象
*
* @param key
* @param obj
* @return
* @since : 2018年11月21日
*/
public <T> boolean putCacheObj(String key, T obj) ;
/**
* 存入单个对象
* 没有默认前缀
* @param key
* @param obj
* @return
* @since : 2018年11月21日
*/
public <T> boolean putCacheNotPrefix(String key, T obj) ;
/**
* 存入单个对象并设定 有效时间
*
* @param key
* @param obj
* @param expireTime
* @since : 2018年11月21日
*/
public <T> void putCacheWithExpireTime(String key, T obj, final long expireTime) ;
/**
* 存入单个值
* @param key
* @param values
* @since : 2018年11月21日
*/
public void putCache(String key, String values) ;
/**
* 存入单个值并设定有效时间
* @param key
* @param values
* @param expireTime
* @since : 2018年11月21日
*/
public void putCacheWithExpireTime2(String key, String values, final long expireTime) ;
/**
* 存入一个集合
*
* @param key
* @param objList
* @return
* @since : 2018年11月21日
*/
public <T> boolean putListCache(String key, List<T> objList) ;
/**
* 存入一个集合并设定 有效时间
*
* @param key
* @param objList
* @param expireTime
* @return
* @since : 2018年11月21日
*/
public <T> boolean putListCacheWithExpireTime(String key, List<T> objList, final long expireTime) ;
/**
* 获取单个对象
*
* @param key
* @param targetClass
* @return
* @since : 2018年11月21日
*/
public <T> T getCache(final String key, Class<T> targetClass);
/**
* 获取单个对象
* 不带默认前缀
* @param key
* @param targetClass
* @return
* @since : 2018年11月21日
*/
public <T> T getCacheNotPrefix(final String key, Class<T> targetClass) ;
/**
* 获取单个值
* @param key
* @return
* @since : 2018年11月21日
*/
public String getCache(final String key) ;
/**
* 获取单个值
* 没有默认前缀
* @param key
* @return
* @since : 2018年11月21日
*/
public String getCacheNotPrefix(final String key);
/**
* 获取一个集合
*
* @param key
* @param targetClass
* @return
* @since : 2018年11月21日
*/
public <T> List<T> getListCache(final String key, Class<T> targetClass) ;
/**
* 精确删除key
*
* @param key
* @since : 2018年11月21日
*/
public void deleteCache(String key) ;
/**
* 精确删除key
* 没有默认前缀
* @param key
* @since : 2018年11月21日
*/
public void delCacheNotPrefix(String key) ;
/**
* 模糊删除key
*
* @param pattern
* @since : 2018年11月21日
*/
public void deleteCacheWithPattern(String pattern);
/**
* 清空所有缓存
*
* @since : 2018年11月21日
*/
public void clearCache() ;
/**
* List-新增一个元素
*
* @param key
* @param values
* @return List中元素的个数
* @since : 2018年11月21日
*/
public Long lPush(String key, String values);
/**
* List-索引首位置插入一个元素
*
* @param key
* @param values
* @return List中元素的个数
* @since : 2018年11月21日
*/
public Long lPushX(String key, String values);
/**
* List-索引尾位置插入一个元素
*
* @param key
* @param values
* @return List中元素的个数
* @since : 2018年11月21日
*/
public Long rPushX(String key, String values) ;
/**
* List-查询索引尾位置的元素
*
* @param key
* @param index
* @return 元素内容
* @since : 2018年11月21日
*/
public String lIndex(String key, long index) ;
/**
* List-查询元素的个数
*
* @param key
* @return 查询元素的个数
* @since : 2018年11月21日
*/
public Long lLen(String key);
/**
* List-移除指定位索引位置的元素
*
* @param key
* @param indext
* @return
* @since : 2018年11月21日
*/
public String lPop(String key) ;
}
redis接口实现
import com.springmybatis.mybat.Service.redis.RedisService;
import com.springmybatis.mybat.util.ProtoStuffSerializerUtil;
import org.springframework.stereotype.Service;
import org.springframework.dao.DataAccessException;
import org.springframework.data.redis.connection.RedisConnection;
import org.springframework.data.redis.core.RedisCallback;
import org.springframework.data.redis.core.RedisTemplate;
import javax.annotation.Resource;
import java.util.List;
import java.util.Set;
/**
* @ClassName RedisServiceImpl
* @Description TODO
* @Author
* @Date 2018/11/21 17:21
* @Version 1.0
**/
@Service("redisService")
public class RedisServiceImpl implements RedisService {
/* spring redis 模版 */
@Resource
private RedisTemplate<String, String> redisTemplate;
/* redis key值 操作前缀 */
private String prefixname = "cache_";
public RedisTemplate<String, String> getRedisTemplate() {
return redisTemplate;
}
public void setRedisTemplate(RedisTemplate<String, String> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public String getPrefixname() {
return prefixname;
}
public void setPrefixname(String prefixname) {
this.prefixname = prefixname;
}
/**
* 存入单个对象
*
* @param key
* @param obj
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> boolean putCacheObj(String key, T obj) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = ProtoStuffSerializerUtil.serialize(obj);
boolean result = redisTemplate.execute(new RedisCallback<Boolean>() {
@Override
public Boolean doInRedis(RedisConnection connection) throws DataAccessException {
return connection.setNX(bkey, bvalue);
}
});
return result;
}
/**
* 存入单个对象
* 没有默认前缀
* @param key
* @param obj
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> boolean putCacheNotPrefix(String key, T obj) {
final byte[] bkey = key.getBytes();
final byte[] bvalue = ProtoStuffSerializerUtil.serialize(obj);
boolean result = redisTemplate.execute(new RedisCallback<Boolean>() {
@Override
public Boolean doInRedis(RedisConnection connection) throws DataAccessException {
return connection.setNX(bkey, bvalue);
}
});
return result;
}
/**
* 存入单个对象并设定 有效时间
*
* @param key
* @param obj
* @param expireTime
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> void putCacheWithExpireTime(String key, T obj, final long expireTime) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = ProtoStuffSerializerUtil.serialize(obj);
redisTemplate.execute(new RedisCallback<Boolean>() {
@Override
public Boolean doInRedis(RedisConnection connection) throws DataAccessException {
connection.setEx(bkey, expireTime, bvalue);
return true;
}
});
}
/**
* 存入单个值
* @param key
* @param values
* @since : 2018年11月21日
* @author : wangdk
*/
public void putCache(String key, String values) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = values.getBytes();
redisTemplate.execute(new RedisCallback<Boolean>() {
@Override
public Boolean doInRedis(RedisConnection connection) throws DataAccessException {
connection.set(bkey, bvalue);
return true;
}
});
}
/**
* 存入单个值并设定有效时间
* @param key
* @param values
* @param expireTime
* @since : 2018年11月21日
* @author : wangdk
*/
public void putCacheWithExpireTime2(String key, String values, final long expireTime) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = values.getBytes();
redisTemplate.execute(new RedisCallback<Boolean>() {
@Override
public Boolean doInRedis(RedisConnection connection) throws DataAccessException {
connection.setEx(bkey, expireTime, bvalue);
return true;
}
});
}
/**
* 存入一个集合
*
* @param key
* @param objList
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> boolean putListCache(String key, List<T> objList) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = ProtoStuffSerializerUtil.serializeList(objList);
boolean result = redisTemplate.execute(new RedisCallback<Boolean>() {
@Override
public Boolean doInRedis(RedisConnection connection) throws DataAccessException {
return connection.setNX(bkey, bvalue);
}
});
return result;
}
/**
* 存入一个集合并设定 有效时间
*
* @param key
* @param objList
* @param expireTime
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> boolean putListCacheWithExpireTime(String key, List<T> objList, final long expireTime) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = ProtoStuffSerializerUtil.serializeList(objList);
boolean result = redisTemplate.execute(new RedisCallback<Boolean>() {
@Override
public Boolean doInRedis(RedisConnection connection) throws DataAccessException {
connection.setEx(bkey, expireTime, bvalue);
return true;
}
});
return result;
}
/**
* 获取单个对象
*
* @param key
* @param targetClass
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> T getCache(final String key, Class<T> targetClass) {
byte[] result = redisTemplate.execute(new RedisCallback<byte[]>() {
@Override
public byte[] doInRedis(RedisConnection connection) throws DataAccessException {
return connection.get((prefixname + key).getBytes());
}
});
if (result == null) {
return null;
}
return ProtoStuffSerializerUtil.deserialize(result, targetClass);
}
/**
* 获取单个对象
* 不带默认前缀
* @param key
* @param targetClass
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> T getCacheNotPrefix(final String key, Class<T> targetClass) {
byte[] result = redisTemplate.execute(new RedisCallback<byte[]>() {
@Override
public byte[] doInRedis(RedisConnection connection) throws DataAccessException {
return connection.get(key.getBytes());
}
});
if (result == null) {
return null;
}
return ProtoStuffSerializerUtil.deserialize(result, targetClass);
}
/**
* 获取单个值
* @param key
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public String getCache(final String key) {
byte[] result = redisTemplate.execute(new RedisCallback<byte[]>() {
@Override
public byte[] doInRedis(RedisConnection connection) throws DataAccessException {
return connection.get((prefixname + key).getBytes());
}
});
if (result == null) {
return null;
}
return new String(result);
}
/**
* 获取单个值
* 没有默认前缀
* @param key
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public String getCacheNotPrefix(final String key) {
byte[] result = redisTemplate.execute(new RedisCallback<byte[]>() {
@Override
public byte[] doInRedis(RedisConnection connection) throws DataAccessException {
return connection.get(key.getBytes());
}
});
if (result == null) {
return null;
}
return new String(result);
}
/**
* 获取一个集合
*
* @param key
* @param targetClass
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public <T> List<T> getListCache(final String key, Class<T> targetClass) {
byte[] result = redisTemplate.execute(new RedisCallback<byte[]>() {
@Override
public byte[] doInRedis(RedisConnection connection) throws DataAccessException {
return connection.get((prefixname + key).getBytes());
}
});
if (result == null) {
return null;
}
return ProtoStuffSerializerUtil.deserializeList(result, targetClass);
}
/**
* 精确删除key
*
* @param key
* @since : 2018年11月21日
* @author : wangdk
*/
public void deleteCache(String key) {
redisTemplate.delete((prefixname + key));
// Long result = redisTemplate.execute(new RedisCallback<Long>() {
// @Override
// public Long doInRedis(RedisConnection connection) throws
// DataAccessException {
// return connection.del(key.getBytes());
// }
// });
// return result;
}
/**
* 精确删除key
* 没有默认前缀
* @param key
* @since : 2018年11月21日
* @author : wangdk
*/
public void delCacheNotPrefix (String key) {
redisTemplate.delete(key);
}
/**
* 模糊删除key
*
* @param pattern
* @since : 2018年11月21日
* @author : wangdk
*/
public void deleteCacheWithPattern(String pattern) {
Set<String> keys = redisTemplate.keys(pattern);
redisTemplate.delete(keys);
}
/**
* 清空所有缓存
*
* @since : 2018年11月21日
* @author : wangdk
*/
public void clearCache() {
deleteCacheWithPattern(prefixname + "|*");
}
/**
* List-新增一个元素
*
* @param key
* @param values
* @return List中元素的个数
* @since : 2018年11月21日
* @author : wangdk
*/
public Long lPush(String key, String values) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = values.getBytes();
Long result = redisTemplate.execute(new RedisCallback<Long>() {
@Override
public Long doInRedis(RedisConnection connection) throws DataAccessException {
return connection.lPush(bkey, bvalue);
}
});
return result;
}
/**
* List-索引首位置插入一个元素
*
* @param key
* @param values
* @return List中元素的个数
* @since : 2018年11月21日
* @author : wangdk
*/
public Long lPushX(String key, String values) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = values.getBytes();
Long result = redisTemplate.execute(new RedisCallback<Long>() {
@Override
public Long doInRedis(RedisConnection connection) throws DataAccessException {
return connection.lPushX(bkey, bvalue);
}
});
return result;
}
/**
* List-索引尾位置插入一个元素
*
* @param key
* @param values
* @return List中元素的个数
* @since : 2018年11月21日
* @author : wangdk
*/
public Long rPushX(String key, String values) {
final byte[] bkey = (prefixname + key).getBytes();
final byte[] bvalue = values.getBytes();
Long result = redisTemplate.execute(new RedisCallback<Long>() {
@Override
public Long doInRedis(RedisConnection connection) throws DataAccessException {
return connection.rPushX(bkey, bvalue);
}
});
return result;
}
/**
* List-查询索引尾位置的元素
*
* @param key
* @param index
* @return 元素内容
* @since : 2018年11月21日
* @author : wangdk
*/
public String lIndex(String key, long index) {
final byte[] bkey = (prefixname + key).getBytes();
byte[] result = redisTemplate.execute(new RedisCallback<byte[]>() {
@Override
public byte[] doInRedis(RedisConnection connection) throws DataAccessException {
return connection.lIndex(bkey, index);
}
});
return new String(result);
}
/**
* List-查询元素的个数
*
* @param key
* @return 查询元素的个数
* @since : 2018年11月21日
* @author : wangdk
*/
public Long lLen(String key) {
final byte[] bkey = (prefixname + key).getBytes();
Long result = redisTemplate.execute(new RedisCallback<Long>() {
@Override
public Long doInRedis(RedisConnection connection) throws DataAccessException {
return connection.lLen(bkey);
}
});
if(result==null){
result=0L;
}
return result;
}
/**
* List-移除指定位索引位置的元素
*
* @param key
* @param indext
* @return
* @since : 2018年11月21日
* @author : wangdk
*/
public String lPop(String key) {
final byte[] bkey = (prefixname + key).getBytes();
String result = redisTemplate.execute(new RedisCallback<String>() {
@Override
public String doInRedis(RedisConnection connection) throws DataAccessException {
return new String(connection.lPop(bkey));
}
});
return result;
}
}
测试结果
import com.springmybatis.mybat.Service.redis.RedisService;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
@RunWith(SpringRunner.class)
@SpringBootTest
public class MybatApplicationTests {
@Autowired
public RedisService redisService;
@Test
public void contextLoads() {
redisService.putCache("你好","你好,redis");
System.out.println(redisService.getCache("你好"));
}
}
打印出结果
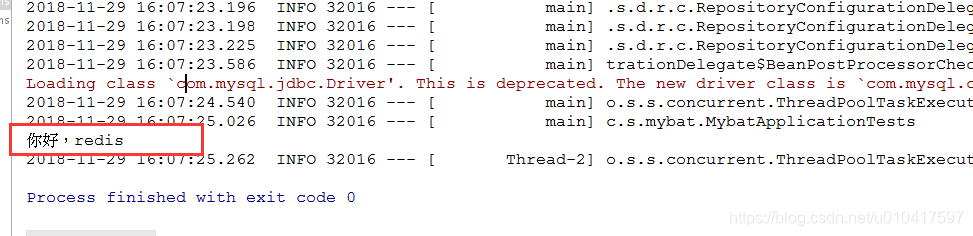
通过redis可视化工具
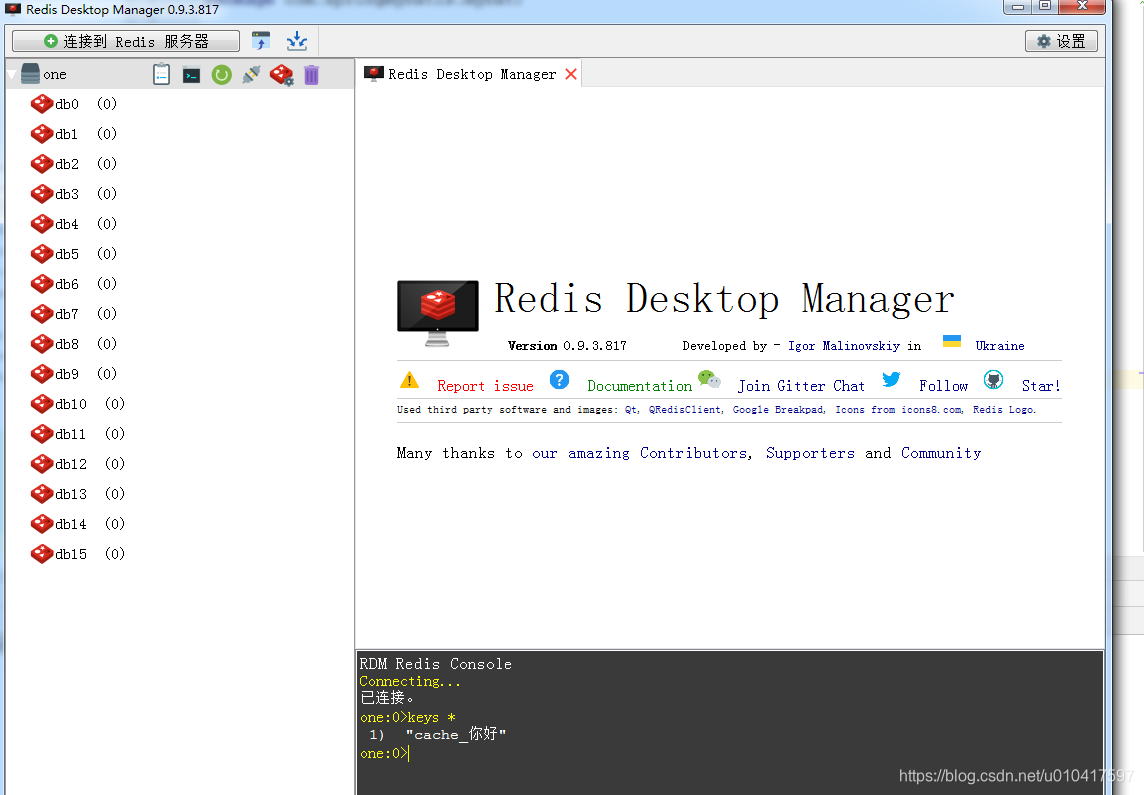