//vs2017
#include
#include “CMap.h”
using namespace std;
int main()
{
SStudent sstu[] = {
{1,“李四”,95.5},
{2,“lisi”,98},
{3,“aslic”,98},
{1,“李思思”,98},
{2,“张三”,92},
{3,“王五”,93.8},
{4,“赵六”,89.5}
};
CMap my(2);
SStudent s1 = {5,"ccc",89};
SStudent s2 = {4,"dddd",109 };
my[1] =&s1;
my[5] = &s1;
//= { 7,"bbb",100 };
int i = 0;
while (i<_countof(sstu))
{
my.SetAt(sstu[i].nNumber, &sstu[i]);
i++;
}
my[4] = &s2;
*my[3] = { 3,"aaaa",95 };
SStudent *s;
if (my.Lookup(3, s))
{
cout << s->nNumber << "\t" << s->cName << "\t" << s->fChinese << endl;
}
else
{
cout << "没有找到" << endl;
};
my.RemoAt(1);
my.Print();
//delete []sstu;
return 0;
}
#pragma once
typedef struct SStudent
{
int nNumber;
char cName[20];
float fChinese;
}DATA;
typedef int KEY;
typedef DATA VALUE;
struct SNode
{
KEY key;
VALUE* pvalue;
SNode *pNext;
};
class CMap
{
//enum{ COUNT = 17 };
int m_nCount;
SNode* *m_pHash;
public:
CMap();
CMap(int nCount=17);
~CMap();
void SetAt(KEY key,VALUE value);
bool Lookup(const KEY key, VALUE * &value)const;
void Print() const;
void RemoAll();
VALUE& operator[](KEY key);
bool RemoAt(KEY key);
};
————————————————————————————
#include “CMap.h”
#include
#include
using namespace std;
CMap::CMap()
{
}
CMap::CMap(int nCount)
{
m_pHash = new SNode*[nCount];
memset(m_pHash,’\0’, sizeof(m_pHash)*nCount);
m_nCount = nCount;
}
CMap::~CMap()
{
RemoAll();
}
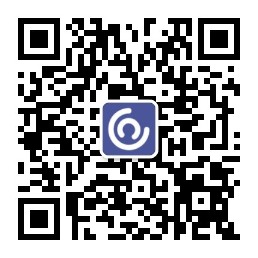
void CMap::RemoAll()
{
int i = 0;
while (i<m_nCount)
{
SNode *p = m_pHash[i], *p1;
while §
{
p1 = p;
p = p->pNext;
delete p1;
}
m_pHash[i] = NULL;
++i;
}
m_nCount = NULL;
}
void CMap::SetAt(KEY key,VALUE pvaule)
{
//operator = pvaule;
(this)[key] = pvaule;
/int n = key % m_nCount;
SNode p = new SNode;
p->key = key;
p->pvalue = pvaule;
p->pNext = NULL;
if (!m_pHash[n])
{
m_pHash[n] = p;
return;
}
SNode p1 = m_pHash[n];
SNode p2 = NULL;
while (p1)
{
if (p1->key==key)
{
p1->pvalue = pvaule;
return;
}
p2 = p1;
p1 = p1->pNext;
}
p2->pNext = p; */
}
bool CMap::Lookup(const KEY key,VALUE * &value) const
{
int n = key % m_nCount;
SNode *p = m_pHash[n];
while §
{
if (p->key == key)
{
value = p->pvalue;
return true;
}
p = p->pNext;
}
return false;
}
void CMap:: Print() const
{
int i=0;
while (i<m_nCount)
{
SNode *p = m_pHash[i];
cout << "第" <<i+1<<"条链表上的数据有"<< endl;
cout << "学号" << "\t" << "姓名" << "\t" << "成绩" << endl;
while (p)
{
cout << p->pvalue->nNumber <<"\t"<< p->pvalue->cName<<"\t" << p->pvalue->fChinese << endl;
p=p->pNext;
}
++i;
}
}
VALUE*& CMap::operator[](KEY key)
{
int n = key % m_nCount;
SNode* *p= &m_pHash[n];
while (*p)
{
if ((*p)->key==key)
{
return (*p)->pvalue;
}
p = &((*p)->pNext);
}
SNode *pData = new SNode;
pData->key = key;
pData->pNext = NULL;
*p = pData;
return pData->pvalue;
}
bool CMap::RemoAt(KEY key)
{
int n = key % m_nCount;
SNode* *p = &m_pHash[n];
while (*p)
{
if ((*p)->key==key)
{
SNode* p1 = *p;
*p = ((*p)->pNext);
delete p1;
return true;
}
p= &((*p)->pNext);
}
return false;
}